Arduino - Control Door Lock via Web
In this tutorial, we will learn how to use Arduino to control a door lock through a web interface that can be accessed using a web browser on a computer or smartphone. Here's how it all works:
- We will program Arduino to act as a web server
- When you type the IP address of your Arduino into the web browser, the web browser makes HTTP request to Arduino.
- Arduino sends back to the web browser with a web page. The web page will show:
- Whether the door is currently locked or unlocked.
- A button to unlock the door.
- Another button to lock the door.
- If this is the first time you use Arduino Uno R4, see how to setup environment for Arduino Uno R4 on Arduino IDE.
- Copy the above code and open with Arduino IDE
- Change the wifi information (SSID and password) in the code to yours
- Click Upload button on Arduino IDE to upload code to Arduino
- Open the Serial Monitor
- Check out the result on Serial Monitor.
- You will see an IP address, for example: 192.168.0.2. This is the IP address of the Arduino Web Server
- Open a web browser and enter one of the three formats below into the address bar:
- Please note that the IP address may be different. Make sure to check the current value on the Serial Monitor.
- Additionally, you will observe the following output on the Serial Monitor.
- Check the door lock state
- You will see the web page of Arduino board on the web browser as below:
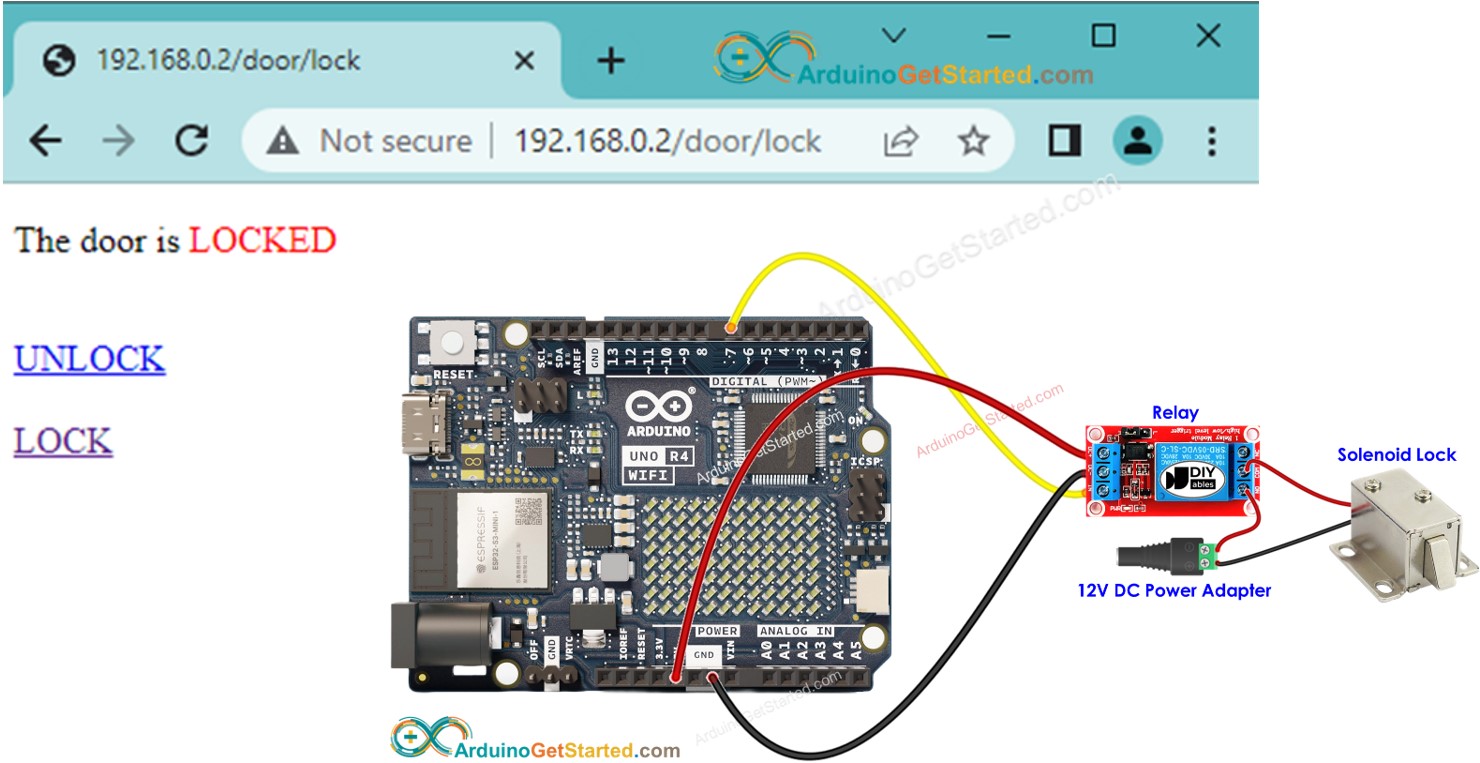
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables .
Additionally, some links direct to products from our own brand, DIYables .
About Solenoid Lock and Arduino Uno R4
If you're not familiar with Arduino Uno R4 and solenoid lock (like their pinout, how they work, and how to program them), you can learn all about them in the following tutorials:
Wiring Diagram
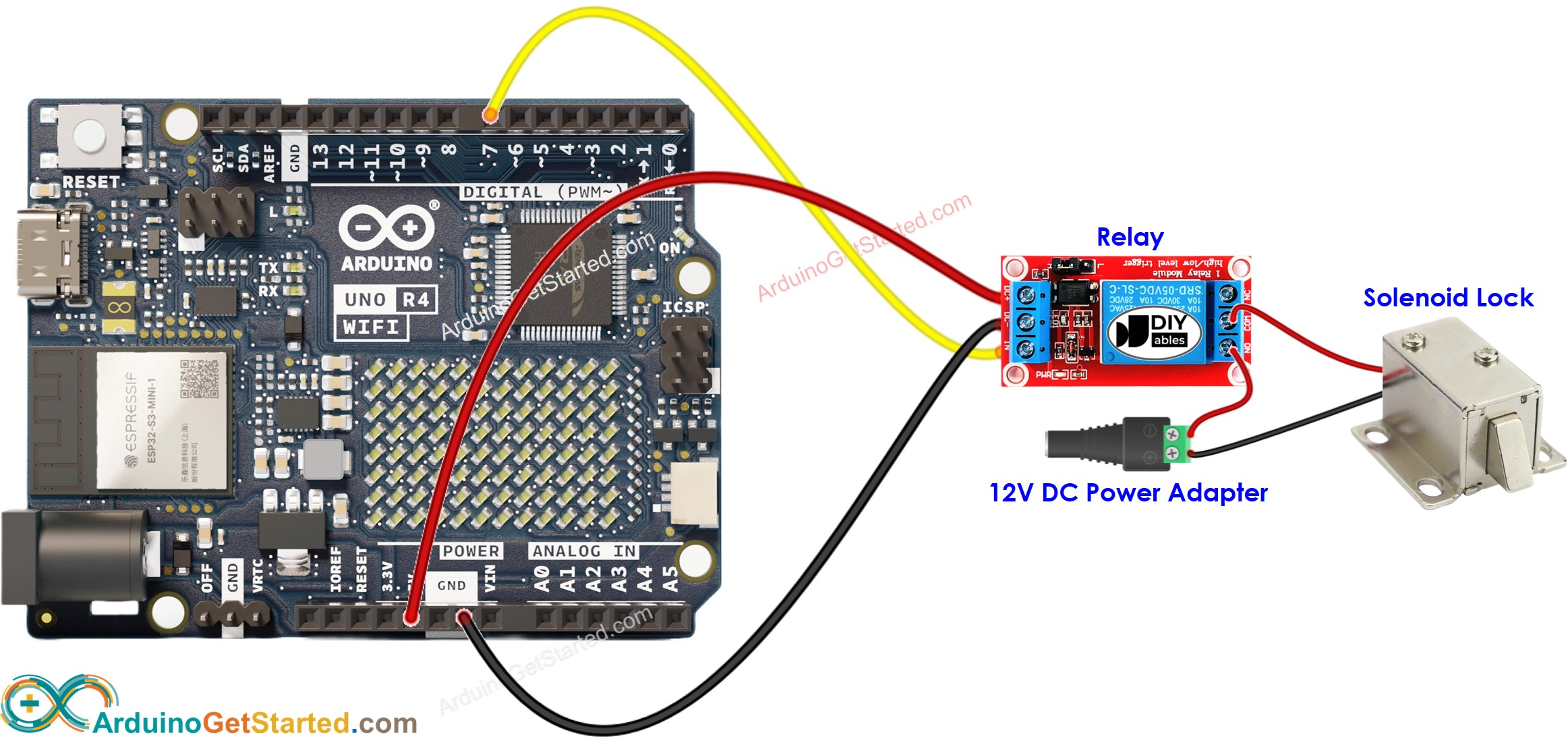
This image is created using Fritzing. Click to enlarge image
Arduino Code
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/tutorials/arduino-controls-door-lock-via-web
*/
#include <WiFiS3.h>
#define RELAY_PIN 7 // Arduino pin connected to the solenoid lock via relay
const char ssid[] = "YOUR_WIFI_SSID"; // change your network SSID (name)
const char pass[] = "YOUR_WIFI_PASSWORD"; // change your network password (use for WPA, or use as key for WEP)
int status = WL_IDLE_STATUS;
int doorState = LOW;
WiFiServer server(80);
void setup() {
//Initialize serial and wait for port to open:
Serial.begin(9600);
pinMode(RELAY_PIN, OUTPUT); // set arduino pin to output mode
String fv = WiFi.firmwareVersion();
if (fv < WIFI_FIRMWARE_LATEST_VERSION) {
Serial.println("Please upgrade the firmware");
}
// attempt to connect to WiFi network:
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to SSID: ");
Serial.println(ssid);
// Connect to WPA/WPA2 network. Change this line if using open or WEP network:
status = WiFi.begin(ssid, pass);
// wait 10 seconds for connection:
delay(10000);
}
server.begin();
// you're connected now, so print out the status:
printWifiStatus();
}
void loop() {
// listen for incoming clients
WiFiClient client = server.available();
if (client) {
// read the first line of HTTP request header
String HTTP_req = "";
while (client.connected()) {
if (client.available()) {
Serial.println("New HTTP Request");
HTTP_req = client.readStringUntil('\n'); // read the first line of HTTP request
Serial.print("<< ");
Serial.println(HTTP_req); // print HTTP request to Serial Monitor
break;
}
}
// read the remaining lines of HTTP request header
while (client.connected()) {
if (client.available()) {
String HTTP_header = client.readStringUntil('\n'); // read the header line of HTTP request
if (HTTP_header.equals("\r")) // the end of HTTP request
break;
Serial.print("<< ");
Serial.println(HTTP_header); // print HTTP request to Serial Monitor
}
}
if (HTTP_req.indexOf("GET") == 0) { // check if request method is GET
// expected header is one of the following:
// - GET door/unlock
// - GET door/lock
if (HTTP_req.indexOf("door/unlock") > -1) { // check the path
doorState = HIGH;
digitalWrite(RELAY_PIN, doorState); // unlock the door
Serial.println("Unlock the door");
} else if (HTTP_req.indexOf("door/lock") > -1) { // check the path
doorState = LOW;
digitalWrite(RELAY_PIN, doorState); // lock the door
Serial.println("Lock the door");
} else {
Serial.println("No command");
}
}
// send the HTTP response
// send the HTTP response header
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close"); // the connection will be closed after completion of the response
client.println(); // the separator between HTTP header and body
// send the HTTP response body
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<head>");
client.println("<link rel=\"icon\" href=\"data:,\">");
client.println("</head>");
client.println("<p>");
client.println("The door is ");
if (doorState == LOW)
client.println("<span style=\"color: red;\">LOCKED</span>");
else
client.println("<span style=\"color: red;\">UNLOCKED</span>");
client.println("</p>");
client.println("<br>");
client.println("<a href=\"/door/unlock\">UNLOCK</a>");
client.println("<br><br>");
client.println("<a href=\"/door/lock\">LOCK</a>");
client.println("</html>");
client.flush();
// give the web browser time to receive the data
delay(10);
// close the connection:
client.stop();
}
}
void printWifiStatus() {
// print your board's IP address:
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
// print the received signal strength:
Serial.print("signal strength (RSSI):");
Serial.print(WiFi.RSSI());
Serial.println(" dBm");
}
Quick Steps
COM6
Attempting to connect to SSID: YOUR_WIFI
IP Address: 192.168.0.2
signal strength (RSSI):-39 dBm
Autoscroll
Clear output
9600 baud
Newline
192.168.0.2
192.168.0.2/door/unlock
192.168.0.2/door/lock
COM6
Attempting to connect to SSID: YOUR_WIFI
IP Address: 192.168.0.2
signal strength (RSSI):-41 dBm
New HTTP Request
<< GET /door/unlock HTTP/1.1
<< Host: 192.168.0.2
<< Connection: keep-alive
<< Cache-Control: max-age=0
<< Upgrade-Insecure-Requests: 1
<< User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64)
<< Accept: text/html,application/xhtml+xml,application/xml
<< Accept-Encoding: gzip, deflate
<< Accept-Language: en-US,en;q=0.9
Unlock the door
Autoscroll
Clear output
9600 baud
Newline
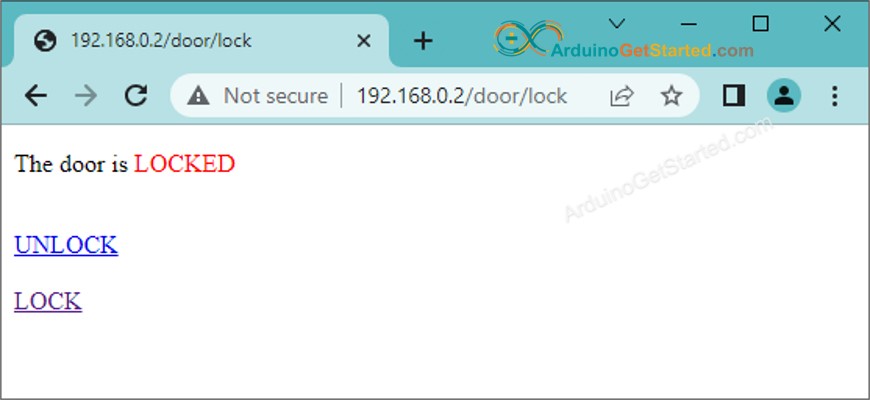
If you desire to enhance the web page's appearance with an impressive graphic user interface (UI), you can refer to the Arduino - Web Server tutorial for inspiration and guidance.