Arduino - Buzzer Library
When using a piezo buzzer, beginners usually run into the trouble that buzzer code blocks other Arduino code because of delau() function.
The ezBuzzer (easy buzzer) library is designed to solve that problems and make it easy to use for not only beginners but also experienced users. It is created by ArduioGetStarted.com.
Library Features
- Supports generating a beep
- Supports playing a melody
- Supports stopping playing a melody
- All functions are non-blocking (no delay() function)
※ NOTE THAT:
If this library is useful for your work, you can say thanks to us by buying us a coffee: PayPal.Me/arduinogetstarted
How To Install Library
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezBuzzer”, then find the buzzer library by ArduinoGetStarted
- Click Install button to install ezBuzzer library.
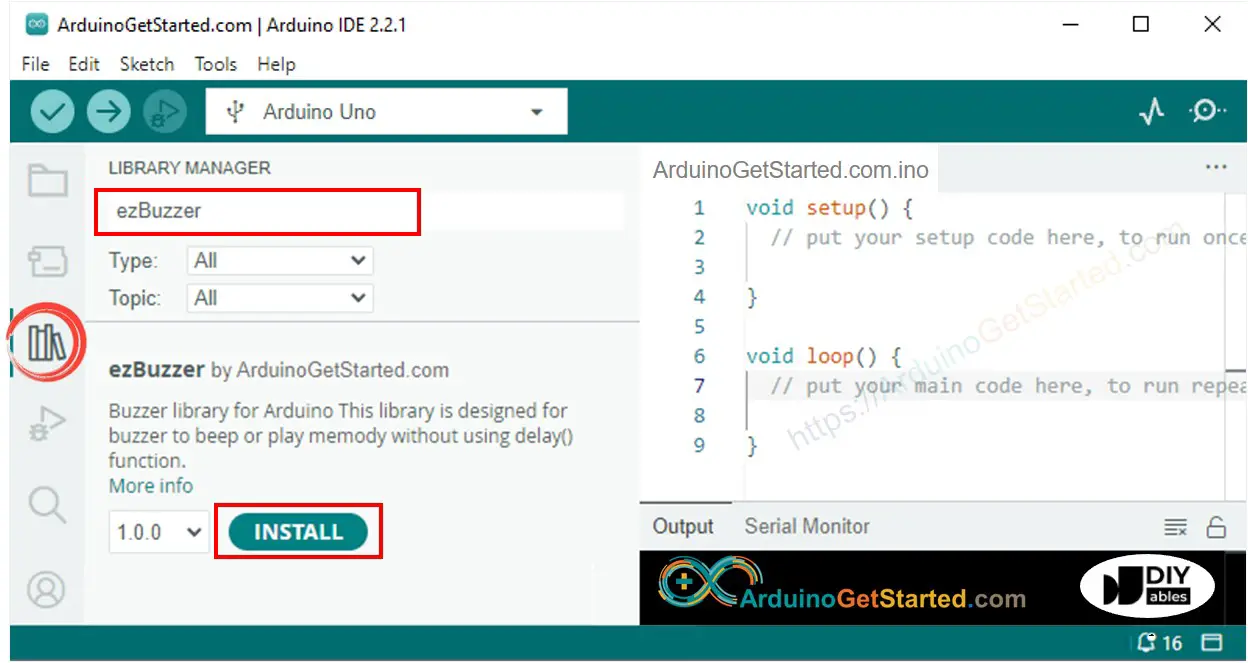
Or you can download it on Github
Examples
Functions
- ezBuzzer()
- stop()
- beep()
- playMelody()
- getState()
- loop()
References
ezBuzzer()
Description
This function creates a new instance of the ezBuzzer class that represents a particular buzzer attached to your Arduino board.
Syntax
Parameters
pin: int - Arduino's pin that connects to the buzzer.
Return
A new instance of the ezBuzzer class.
Example
stop()
Description
This function stop playing beep or melody. It will set the state of buzzer to BUZZER_IDLE.
Syntax
Parameters
None
Return
None
beep()
Description
This function generates a beep. The beep is run on background without blocking other Arduino code. When a beep is ended, The function will set the state of buzzer to BUZZER_IDLE.
Syntax
Parameters
- beepTime: unsigned long - the time buzzer beeps (in milliseconds).
- delay: unsigned long - the time delay befor beeping (in milliseconds).
Return
None
playMelody()
Description
This function play a melody. The melody is run on background without blocking other Arduino code. When a melody is ended, The function will set the state of buzzer to BUZZER_IDLE.
Syntax
Parameters
- melody: int melody[] - An int array stores note's information.
- noteDurations: int noteDurations[] - An int array stores note's duration.
- length: int - The number of notes stores in melody[] array.
Return
None
getState()
Description
This function returns the state of the buzzer.
Syntax
Parameters
None
Return
One of the following states of buzzer:
- BUZZER_IDLE
- BUZZER_BEEP_DELAY
- BUZZER_BEEPING
- BUZZER_MELODY
loop()
Description
This function does play beep, melody on background. It MUST be called in the main loop() function.
Syntax
Parameters
None
Return
None