Arduino - MySQL
Arduino can collect sensor data and store it on the MySQL database. Arduino can also get data (command) from MySQL database and control LED, motor, actuator, device...
In this tutorial, we are going to learn:
- The best way for Arduino to interact with MySQL database
- How Arduino insert data to MySQL database
- How Arduino update data to MySQL database
- How Arduino get data from MySQL database
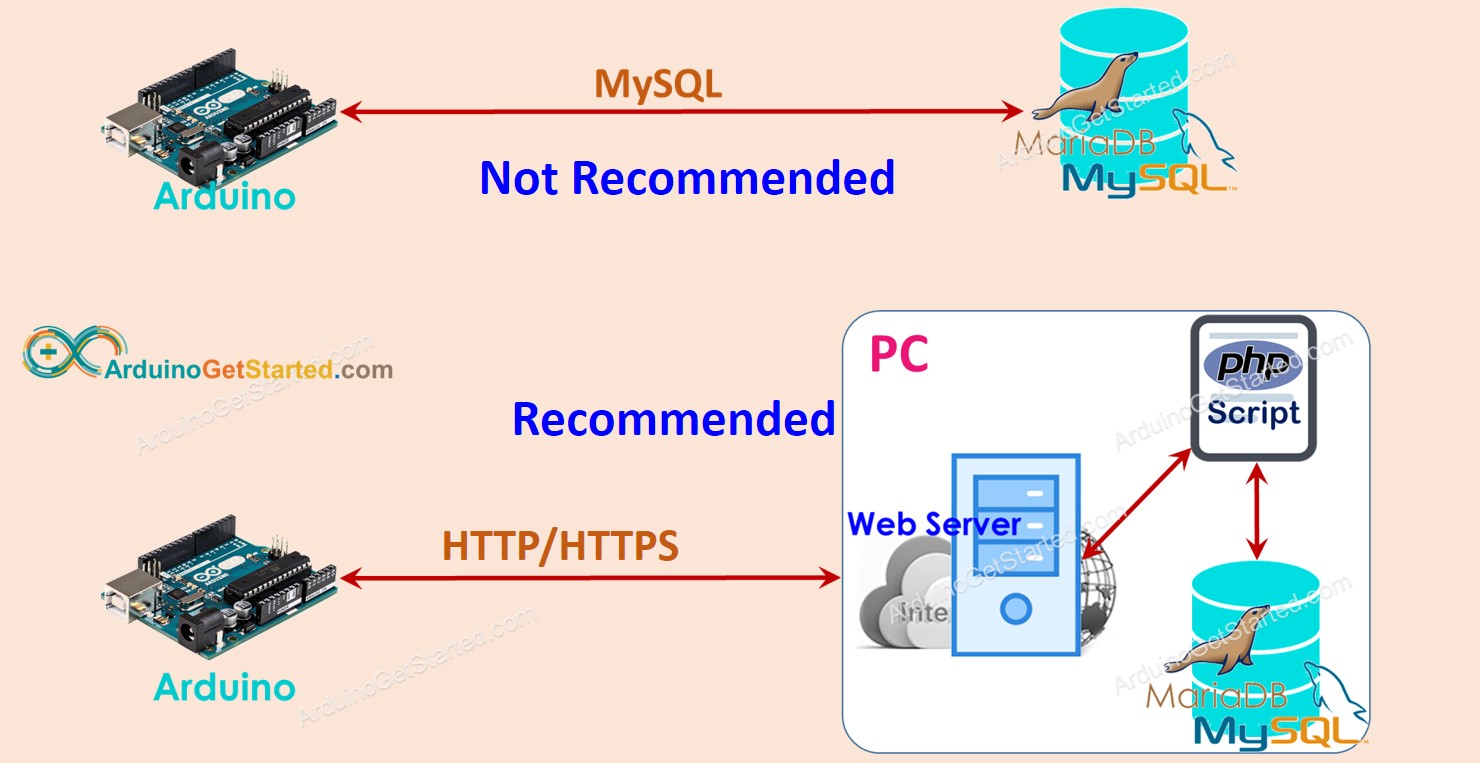
The tutorial provides the Arduino code for two cases:
- Arduino Uno R4 WiFi
- Arduino Uno/Mega with Ethernet Shield
Hardware Required
Alternatively if using Ethernet:
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
Arduino - MySQL
If you do not have much knowledge of the system architecture, two terms: MySQL Database and MySQL Server can be understood as the same. You will find the differences later when you know a lot about the system architecture.
There are two ways for Arduino to interact with the MySQL database:
- Arduino interacts directly to MySQL Server via MySQL connection (called direct way)
- Arduino interacts indirectly to MySQL Server via HTTP connection (called indirect way)
Let's find the best one.
Arduino interacts directly to MySQL Server
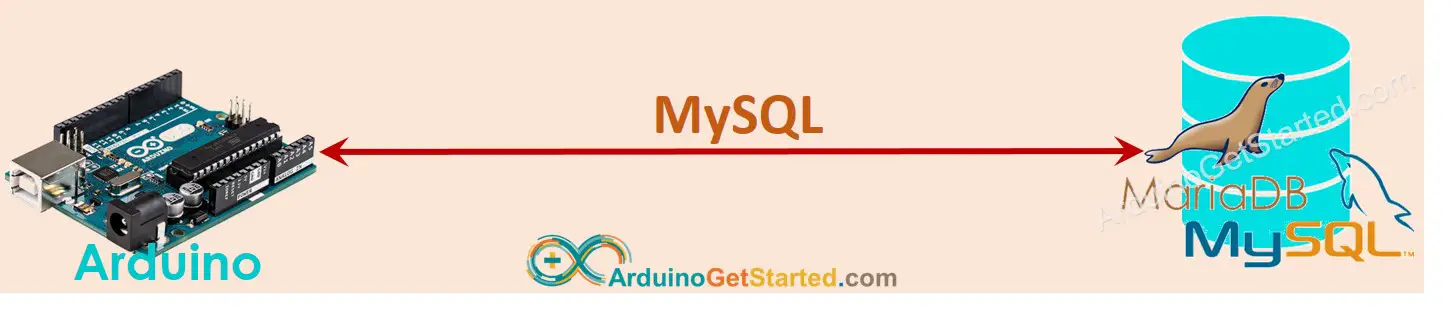
This sounds simpler but there are many disadvantages:
- This allows a MySQL User account to remotely access MySQL database ⇒ This is dangerous from the security point of view, even if limited privileges were granted to the user account.
- The data MUST be processed in Arduino and/or MySQL server ⇒ This increases the complexity of Arduino code and MySQL script. Especially, it consumes a lot of Arduino resources (Memory and CPU usage).
- MySQL server may return a very big amount of data to Arduino in some cases ⇒ This can make Arduino run out of memory.
- Most of the available MySQL libraries do not support SSL/TLS. The data including username/password will be sent in plain text ⇒ another security issue.
Arduino interacts indirectly to MySQL Server via HTTP/HTTPS
This indirect way solves all problems that the direct way has. Before seeing how the indirect way overcomes the disadvantages of the direct way, let's see how it works first
How it works
- Step 1: Arduino makes HTTP Request to Web Server
- Step 2: Web Server runs PHP script
- Step 3: PHP script gets data from HTTP Request, processes the data, and then interacts with MySQL database.
- Step 4: PHP script processes the result and returns the result to Arduino via HTTP Response
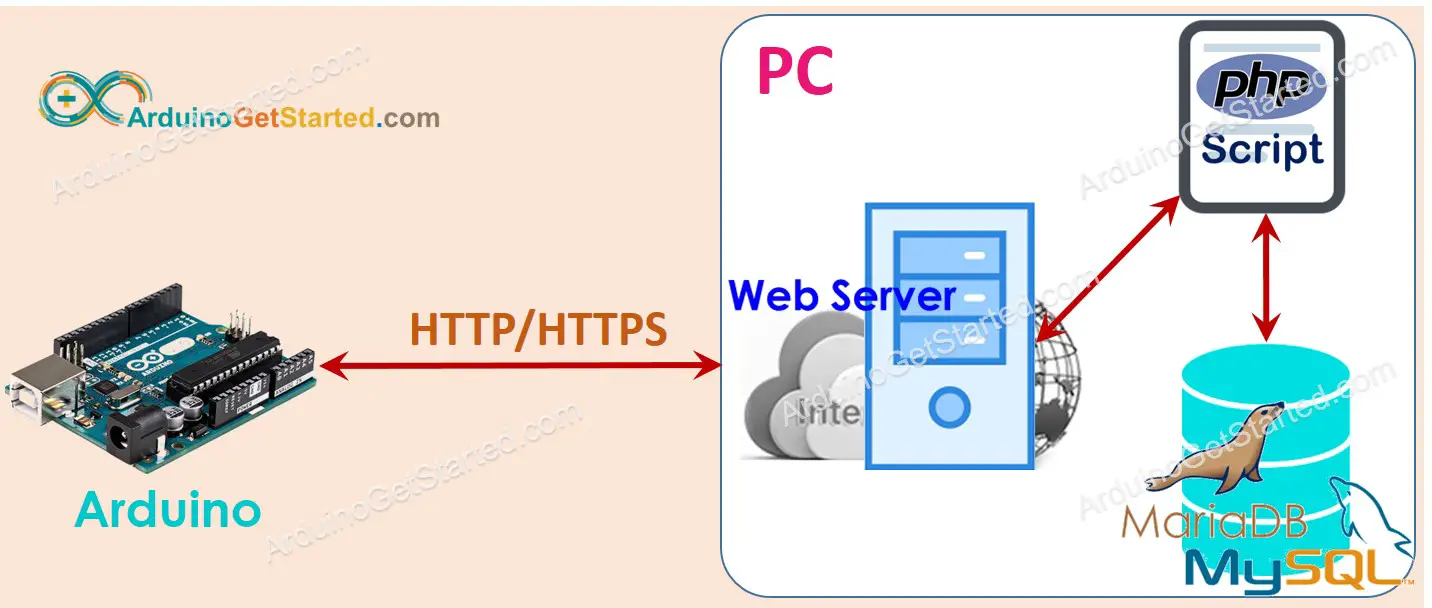
In this tutorial, the Web server, MySQL server will be installed on PC.
It looks complicated but not. Now Let's see how the indirect way overcomes the disadvantages of the direct way.
- By installing the MySQL server and HTTP server in the same physical server, We can limit a MySQL User account to access localhost ONLY. Moreover, the username/password of the MySQL account is stored on Server (step 3), this makes the system more secure.
- Data is processed by a PHP script(step 3 and step 4). This reduces the works and complexity for Arduino and MySQL servers. Processing data using PHP code is much easier than the Arduino code and MySQL script.
- PHP script can process the data and send only necessary data to Arduino (Step 4) to prevent Arduino from running out of memory.
- Most of Ethernet/WiFi libraries supports TLS/SSL that allows us to make HTTPS request. By using HTTPS, the data is encrypted and securely exchanged over the Internet.
In step 1, we can use another username/password to do authentication between Arduino and Web Server. Please note that the HTTP username/password should be different from the MySQL username/password for security reasons.
With those advantages, the rest of this tutorial will present how to use Arduino with MySQL via indirect way.
Arduino - MySQL via HTTP/HTTPS
We need to do the following step:
- Install MySQL server, Web server, and PHP on your PC
- Enable MySQL and Web server
- Create a MySQL User account
- Create a MySQL database
- Create a MySQL table
- Write one or more PHP script files
- Write Arduino code
Now let's do it step-by-step.
1. Install MySQL server, Web server, and PHP on your PC
Fortunately, the XAMPP package includes all of these. We just need to install one time
- Download XAMPP from this link
- Install it.
After installing, you will see C:\xampp\htdocs folder on your PC. This is where you put PHP code (see later).
2. Enable MySQL and Web server
- Open XAMPP Control Panel
- Click Start button to enable MySQL and Web server (See the below image)
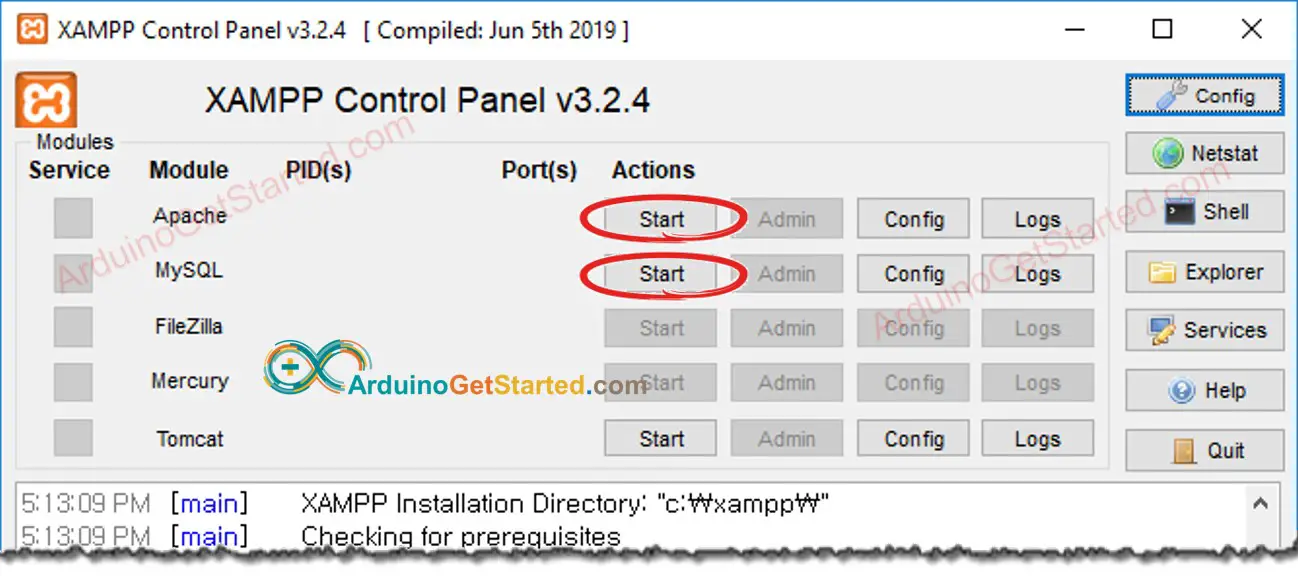
3. Create a MySQL User account
We will create a MySQL account that can connect to the MySQL database from localhost only.
- Even if username/password are revealed, the attackers still cannot access your MySQL database unless they take control of your PC.
- Because PHP and MySQL are installed on the same PC, PHP can use this username/password to connect to the MySQL database.
Let's create a MySQL user account with username is Arduino and password is ArduinoGetStarted.com:
- Open Command Prompt on your PC. Do not close it until the end of the tutorial.
- Type the following command on Command Prompt:
- By default, MySQL has root account without password. You should add password
(e.g. your-root-password) for root account by typing the following command on Command Prompt:
- Type the following command on Command Prompt:
- Type your-root-password and press Enter
- Create a MySQL user account with username is Arduino and password is ArduinoGetStarted.com by coping the below commands and paste on Command Prompt:
Now you successfully created and MySQL user account. Memorize the username/password, It will be used in PHP script.
4. Create a MySQL database
Let's create a database named db_arduino by typing the following command on Command Prompt:
5. Create a MySQL table
Let's create a table named tbl_temp by coping the below commands and paste on Command Prompt:
6. Write one or more PHP files
Create a PHP file named insert_temp.php that gets temperature from HTTP Request and inserts it into the database.
- Place this file inside C:\xampp\htdocs folder
- Get your PC's IP address. If you do not know how to, google it.
- Test PHP code by open a web browser (e.g. Chrome) and access this link: http://192.168.0.26/insert_temp.php?temperature=27.5 . Note that you need to replace the above IP address with your PC address.
- The output on the web browser
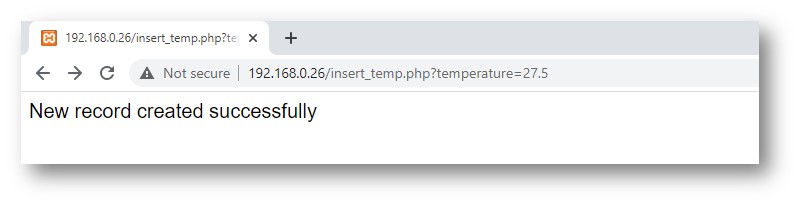
- Check whether data is stored in database by typing the following command on Command Prompt:
As you can see, the temperature of 27.5 is stored in the database. The next step is to write Arduino that makes a similar HTTP Request to your PC.
7. Write Arduino code
We will use Arduino Uno and Ethernet Shield or Arduino Uno R4 WiFi for the test
The below Arduino code makes HTTP to your PC to insert a temperature of 29.1°C into the database
Arduino Code for Arduino Uno R4 WiFi
Arduino Code for Arduino Uno/Mega and Ethernet Shield
Quick Steps
- If using the Ethernet Shield, stack Ethernet Shield on Arduino Uno
- Connect Ethernet Cable to Ethernet Shield
- Connect Arduino Uno to PC via USB cable
- Change IP address on the code by your PC's IP address
- Compile and upload code to Arduino
- Open Serial Monitor
- The result on Serial Monitor
- Check whether data is stored in database by typing the following command on Command Prompt:
As you can see, the temperature 29.1 is stored in database.
How Arduino insert, update or get data to/from MySQL database
In the above example, we have learned how to insert data into the MySQL database. For updating and getting data from the database, it is similar. You only need to change MySQL queries on the PHP script. You can learn more from W3Schools
Advanced Usage
To increase the security
- You can change Arduino code to make HTTPS instead of HTTP. See Arduino - HTTPS
- You can use a username/password to do authentication between Arduino and the Web server. See Basic access authentication
※ NOTE THAT:
To make a complete system with the highest security level, we need to do more (such as MySQL injection prevention, making HTTPS become REST API, using Json format for data ...). Howerver, this tutorial is dedicated for beginners to learn Arduino. We made it as simple as possible. After learning this tutorial, users can expand it.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.