Arduino - Touch Sensor
Touch sensor (also called touch button or touch switch) is widely used to control devices (e,g. touchable lamp). It has the same functionality as a button. It is used instead of the button on many new devices because it makes the product look neat.
In this tutorial, we will learn how to use the touch sensor with Arduino.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About Touch Sensor
Pinout
Touch sensor has 3 pins:
- GND pin: needs to be connected to GND (0V)
- VCC pin: needs to be connected to VCC (5V or 3.3v)
- SIGNAL pin: is an output pin: LOW when it is NOT touched, HIGH when it is touched. This pin needs to be connected to Arduino's input pin.
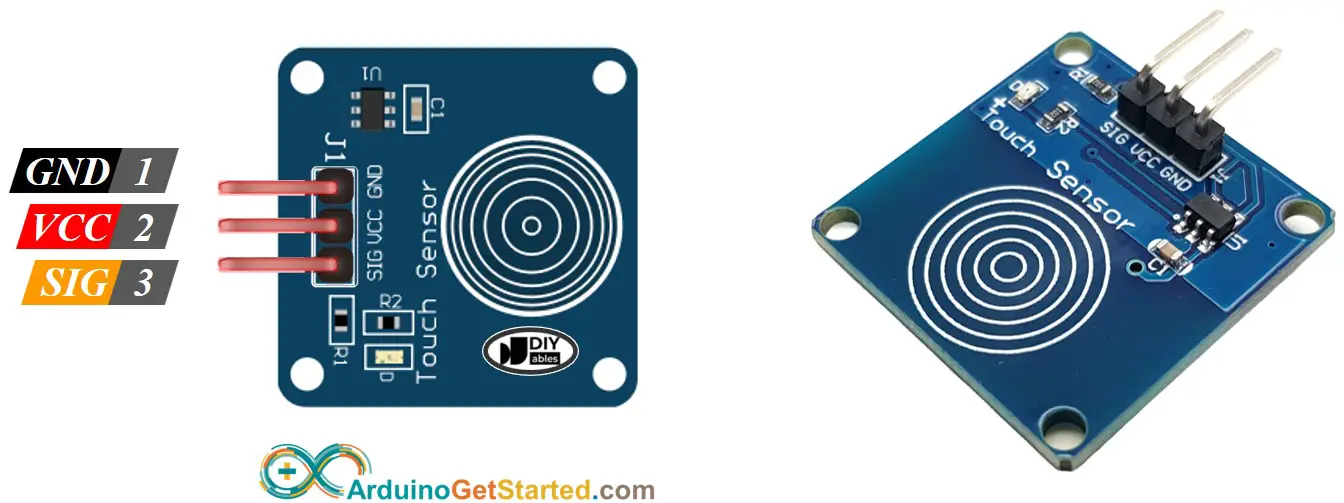
How It Works
- When the sensor is NOT touched, the sensor's SIGNAL pin is LOW
- When the sensor is touched, the sensor's SIGNAL pin is HIGH
Arduino - Touch Sensor
The touch sensor's SIGNAL pin is connected to an Arduino's input pin.
By reading the state of Arduino's pin (configured as an input pin), we can detect whether the touch sensor is touched or not.
Wiring Diagram
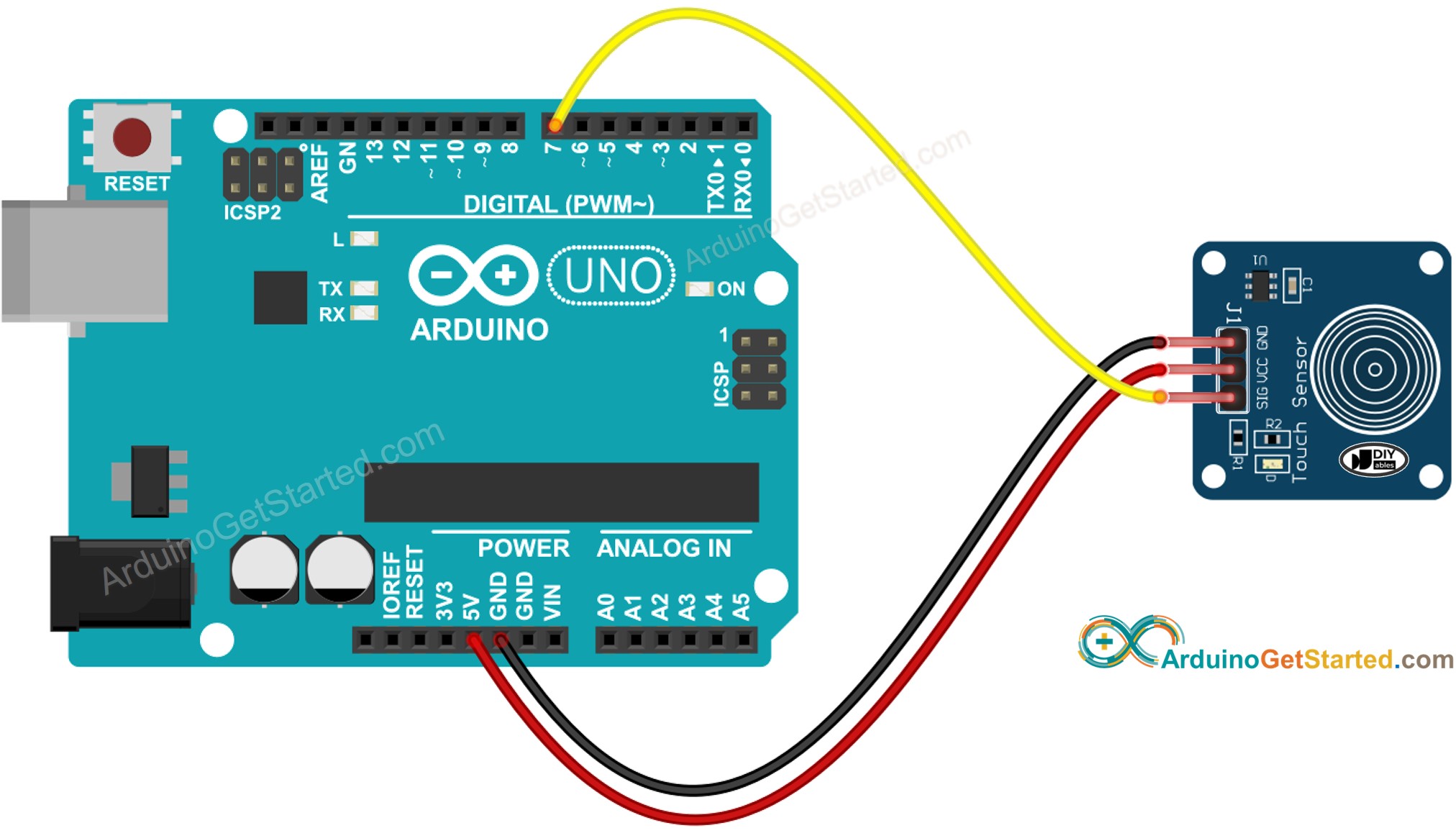
This image is created using Fritzing. Click to enlarge image
How To Program For Touch Sensor
- Initializes the Arduino pin to the digital input mode by using pinMode() function. For example, pin 7
- Reads the state of the Arduino pin by using digitalRead() function.
※ NOTE THAT:
There are two wide-used use cases:
- The first: If the input state is HIGH, do something. If the input state is LOW, do another thing in reverse.
- The second: If the input state is changed from LOW to HIGH (or HIGH to LOW), do something.
Depending on the application, we choose one of them. For example, in case of using a touch sensor to control an LED:
- If we want the LED to be ON when the sensor is touched and OFF when the sensor is NOT touched, we SHOULD use the first use case.
- If we want the LED to be toggle between ON and OFF each time we touch the sensor, we SHOULD use the second use case.
- How to detect the state change from LOW to HIGH
Touch Sensor - Arduino Code
We will run four example codes:
- Reads the value from the touch sensor and print to the Serial Monitor.
- Controls LED according to the sensor's state.
- Detects the sensor is touched or released.
- Toggles LED when the sensor is touched (This is the most common-used).
Reads the value from the touch sensor and print to the Serial Monitor
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Touch your finger to the sensor and release.
- See the result on Serial Monitor.
Controls LED according to the sensor's state
If the sensor is touched, turn LED on. If the sensor is not touched, turn LED off.
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Touch your finger to the sensor and keep touching.
- See LED state ⇒ LED should be on.
- Release your finger from the sensor.
- See LED state ⇒ LED should be off.
Detects the sensor is touched or released
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Touch your finger to the sensor and keep touching.
- See the result on Serial Monitor.
- Release your finger from the sensor.
- See the result on Serial Monitor.
Toggles LED when the sensor is touched.
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Touch your finger to the sensor and release.
- See LED state ⇒ LED should be on.
- Touch your finger to the sensor and release.
- See LED state ⇒ LED should be off.
- Touch your finger to the sensor and release.
- See LED state ⇒ LED should be on.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.