Arduino - Potentiometer fade LED
In a previous tutorial, We have learned how a potentiometer triggers a LED. In this tutorial, We are going to learn how to change the brightness of LED according to the potentiometer's output value
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About LED and Potentiometer
If you do not know about LED and potentiometer (pinout, how it works, how to program ...), learn about them in the following tutorials:
Wiring Diagram
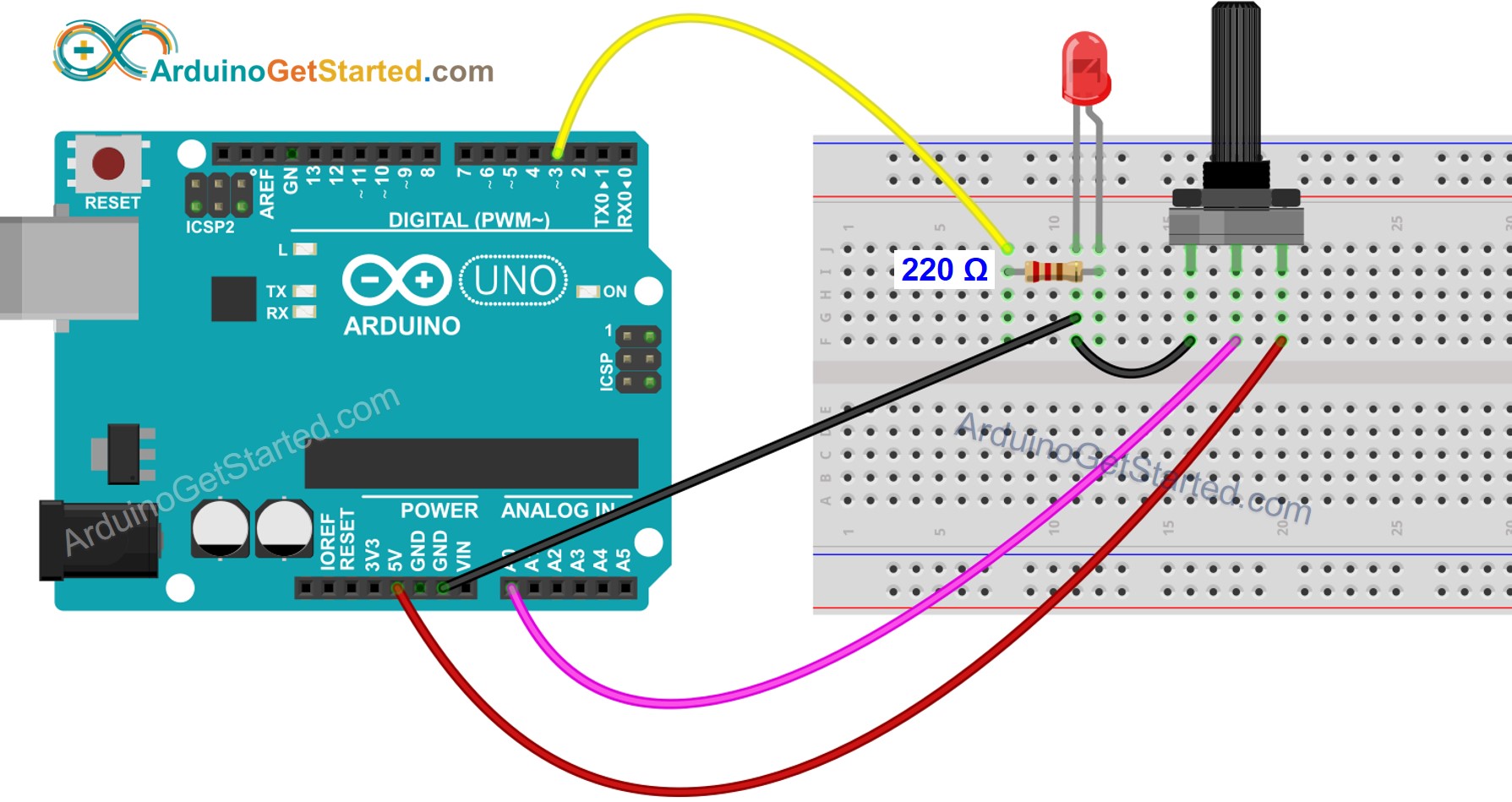
This image is created using Fritzing. Click to enlarge image
How To Program
- Reads the input on analog pin A0 (value between 0 and 1023)
- Scales it to brightness (value between 0 and 255)
- Sets the brightness LED that connects to pin 3
Arduino Code
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Open Serial Monitor
- Rotate the potentiometer
- See the LED
- See the result on Serial Monitor
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
LED on Commercial Products
Small LEDs usually are used to indicate the status of devices. For examples:
Big LEDs usually are used for lighting. They can be combined into groups. For examples: