Arduino RS232 to WiFi
In this tutorial, we are going to learn how to use the Arduino Uno R4 WiFi to create a converter that connects Serial RS232 devices to WiFi. With this setup, the Arduino will read data from a serial RS232 interface and send it to a TCP server, either in the same local network or over the internet. It can also receive data from the TCP server and send it back through the serial RS232 interface.
Following these steps will help you set up flexible communication bridges between serial RS-232 devices and TCP/IP servers using Arduino.
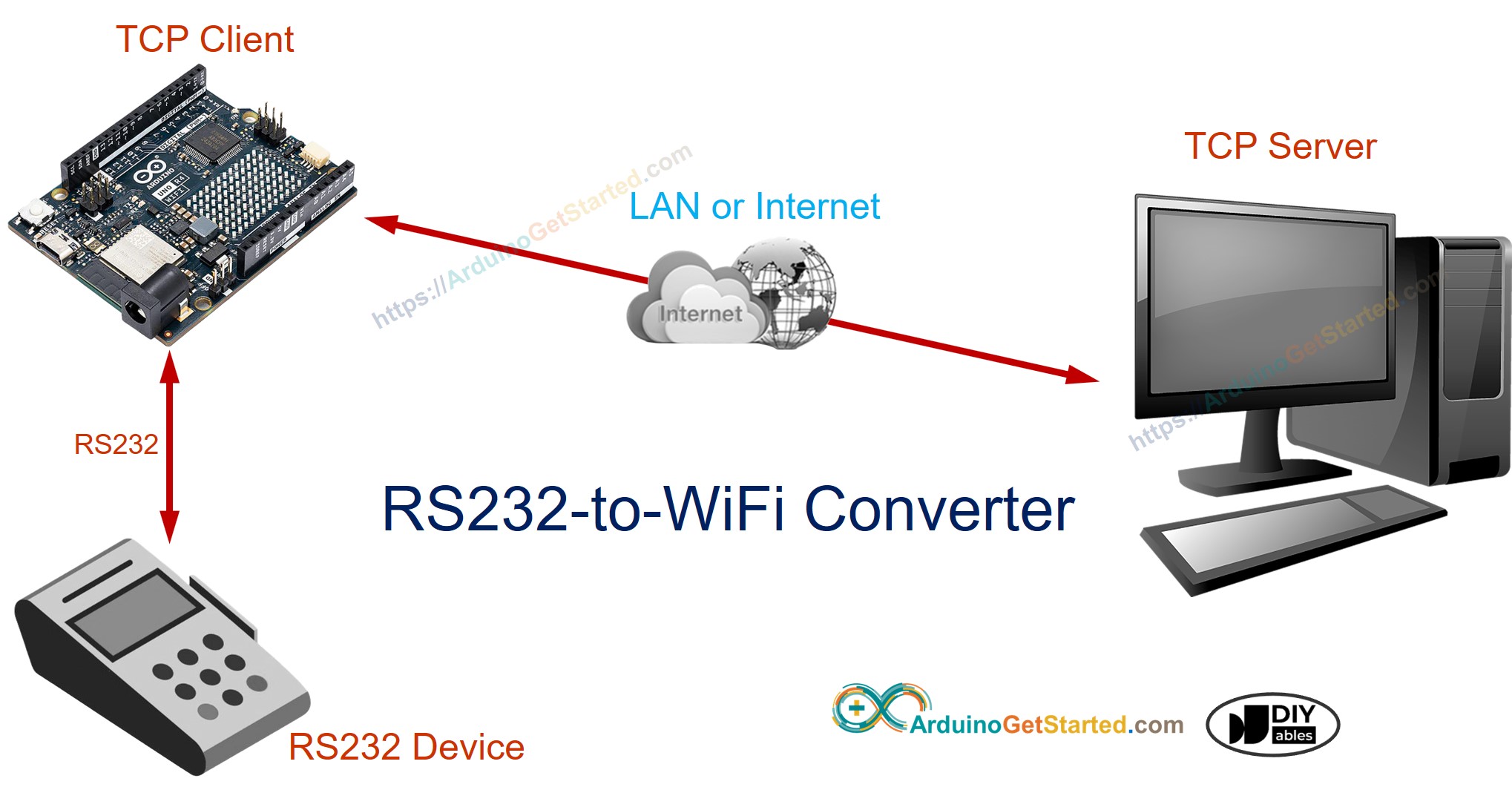
Hardware Required
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About RS232 and TCP
If you do not know about how to use RS232 and TCP communication with Arduino, learn about them in the following tutorials:
- Arduino - RS232 tutorial
- Arduino - TCP Client tutorial
How RS232 to WiFi converter Works
- Arduino connects to a serial device via the RS232 interface.
- Arduino acts like a TCP client, connecting to a TCP server. The TCP server could be a software running on your computer or another Arduino programmed to act as a TCP server.
- Arduino reads information from the serial RS232 interface and sends it to the TCP server.
- Arduino also reads data from the TCP connection and sends it back through the serial RS232 interface.
Wiring Diagram
- Wiring diagram if using hardware serial
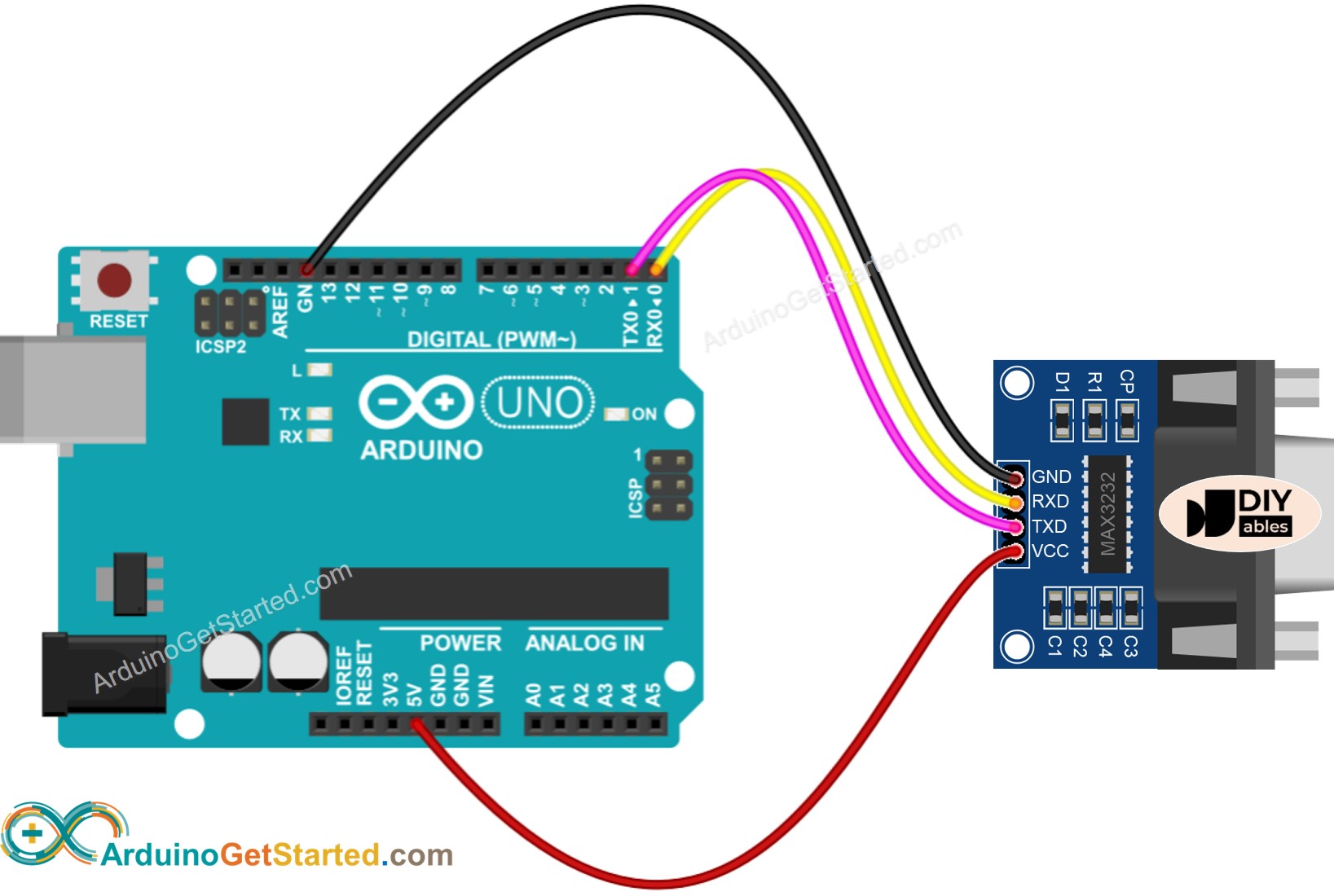
This image is created using Fritzing. Click to enlarge image
- Wiring diagram if using software serial
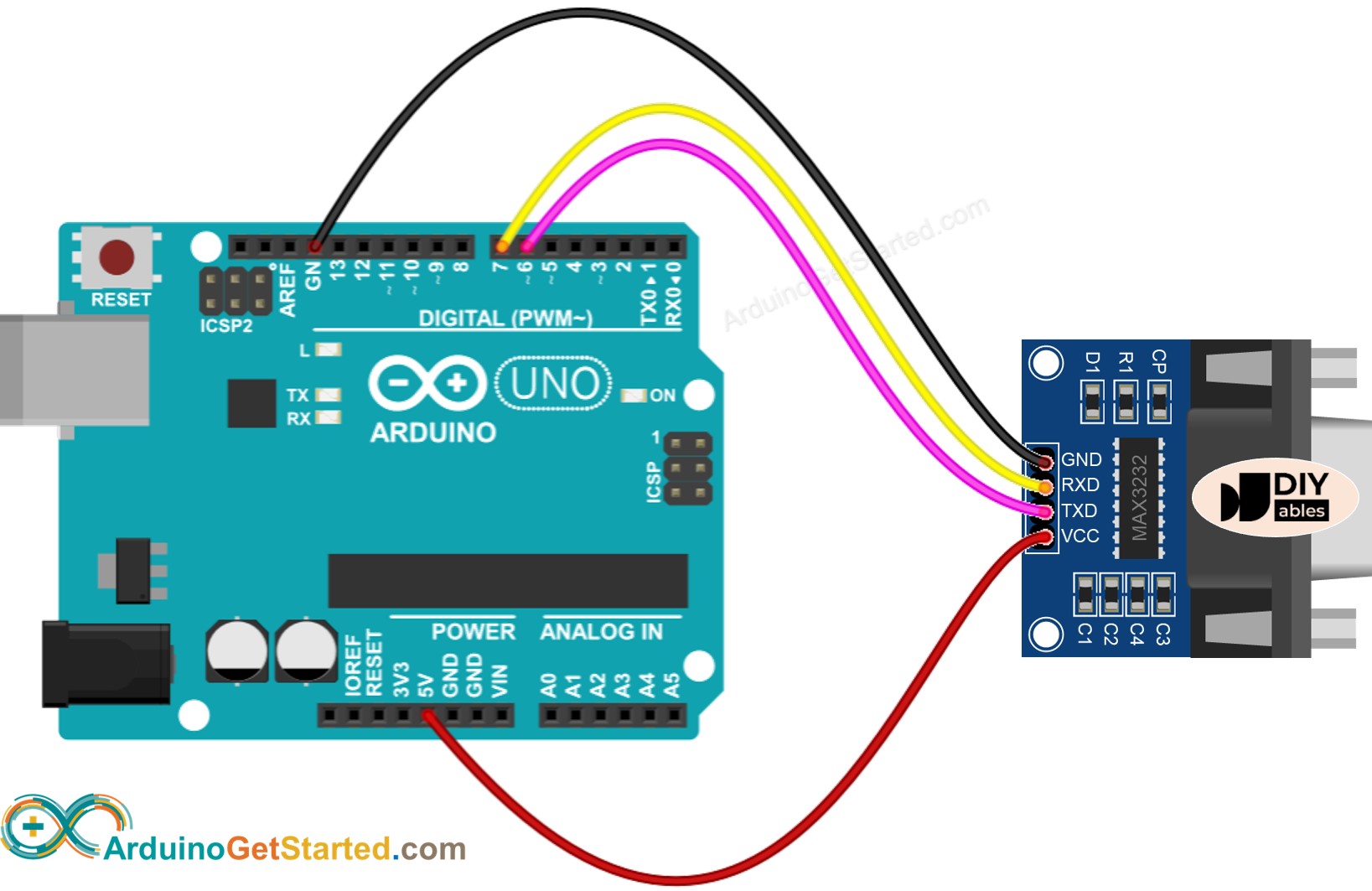
This image is created using Fritzing. Click to enlarge image
Arduino Code for Hardware Serial
Arduino Code for Software Serial
Testing
You can do a test by sending data in the following flows:
- Serial Software (on your PC) → RS-232 → Arduino → WiFi → TCP Server Software (on your PC).
- TCP Server Software (on your PC) → WiFi → Arduino → RS-232 → Serial Software (on your PC).
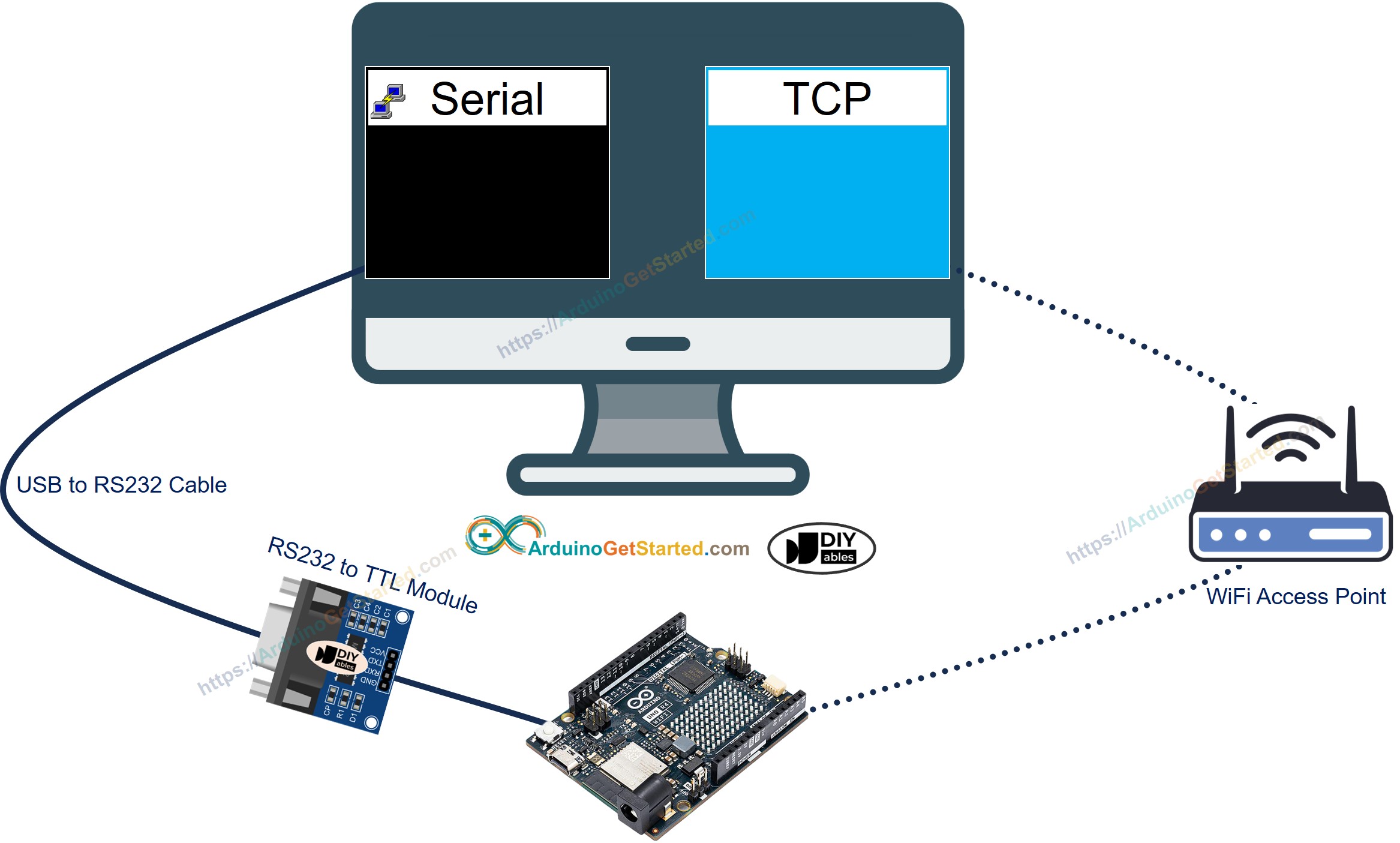
To do it, follow the below steps:
- If this is the first time you use Arduino Uno R4, see how to setup environment for Arduino Uno R4 on Arduino IDE.
- Connect Arduino Uno R4 WiFi to your PC by using TTL-to-RS232 module and RS232-to-USB cable as above wiring diagram
- Install a TCP server software program like ezTerm
- Open the Serial program and configure the Serial parameters (COM port, baurate...)
- Open the TCP server program and configure it as TCP Server, then click Listen button
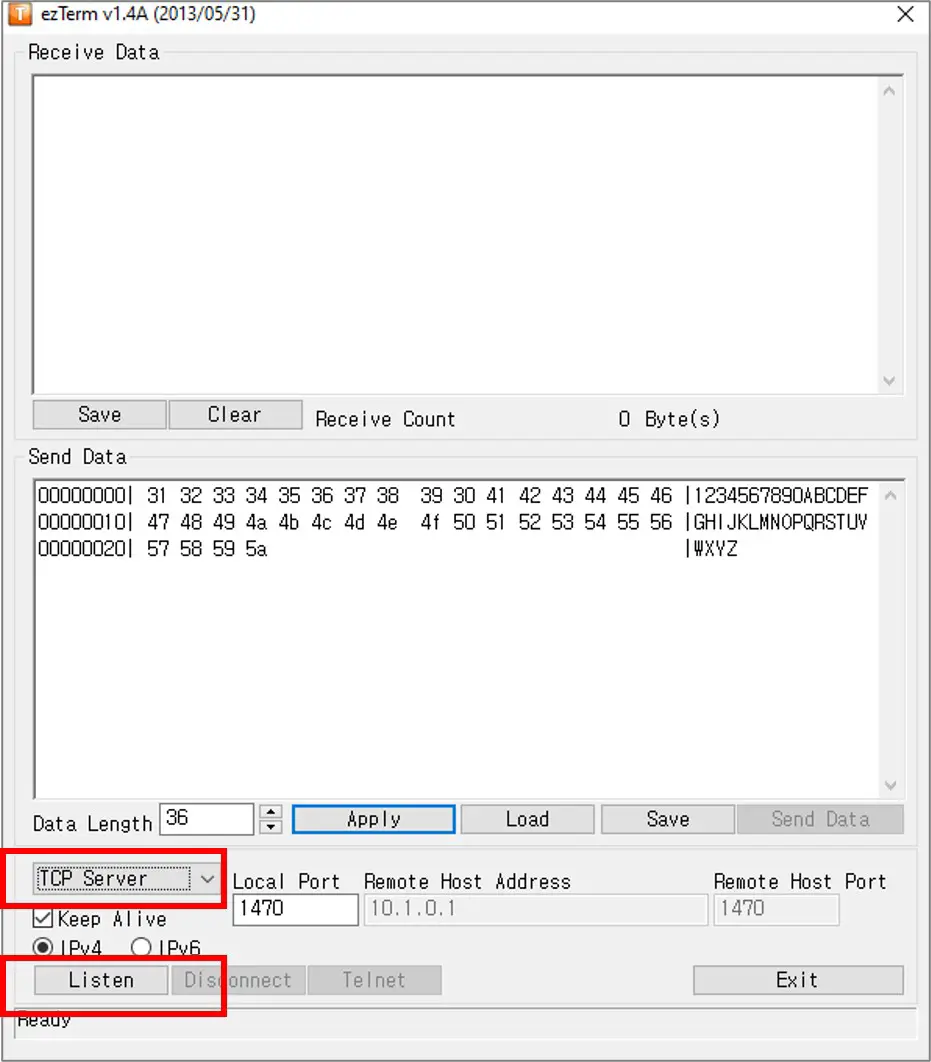
- Open Command Prompt on your PC.
- Find the IP address of your PC by running the below command:
- The output looks like below:
- Update the IP address of TCP Server (Your PC) in the Arduino code. In the above example: 192.168.0.26
- Compile and upload code to Arduino board by clicking Upload button on Arduino IDE
- Type some data from the Serial program to send it to Arduino via Serial.
- If successful, you will see the echo data on the TCP server software.
- Type some data from the TCP server program to send it to Arduino via TCP.
- If successful, you will see the echo data on the Serial program.
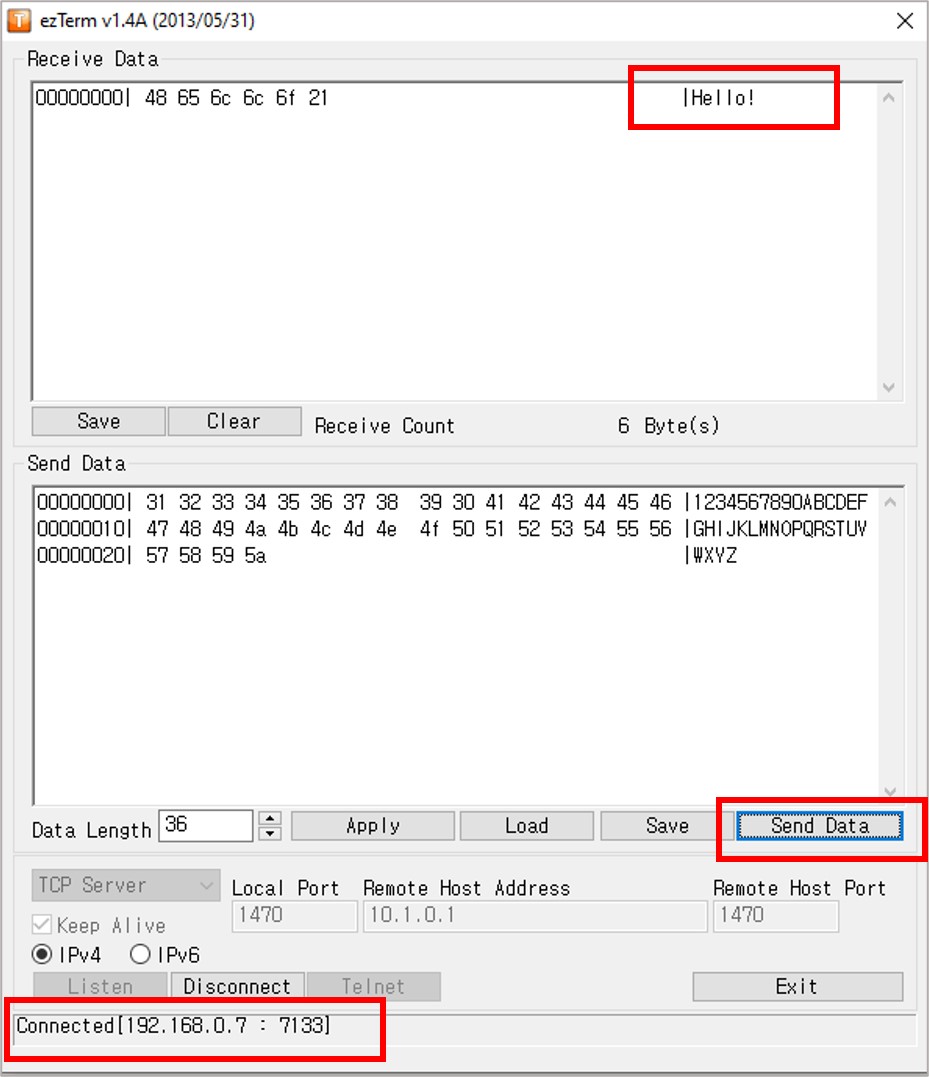
If you want to use a comercial RS232-To-Ethernet converter, you can buy CSE-H53N Serial To Ethernet Converter
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.