Arduino - Stepper Motor and Limit Switch
In this Arduino tutorial, We are going to learn how to use Arduino, limit switch, L298N driver and stepper motor. In detail, we are going to learn:
- How to stop stepper motor when a limit switch is touched
- How to change the direction of stepper motor when a limit switch is touched
- How to change the direction of stepper motor when two limit switches is touched
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About Stepper Motor and Limit Switch
If you do not know about stepper motor and limit switch (pinout, how it works, how to program ...), learn about them in the following tutorials:
- Arduino - Limit Switch tutorial
- Arduino - Controls Stepper Motor tutorial
Wiring Diagram
This tutorial provides the Arduino codes for two cases: One stepper motor + one limit switch, One stepper motor + two limit switches.
- Wiring diagram between the stepper motor and a limit switch
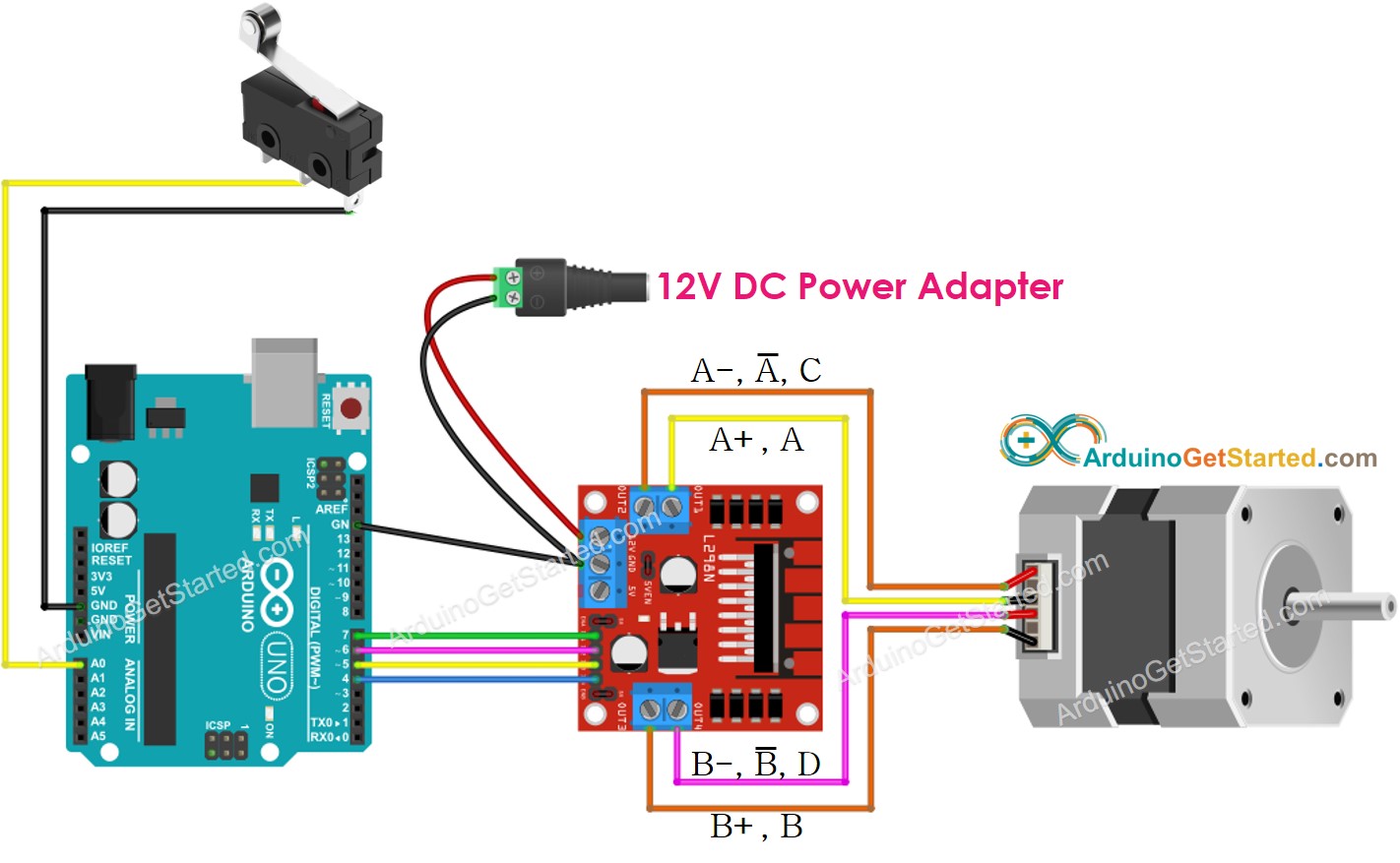
This image is created using Fritzing. Click to enlarge image
- Wiring diagram between the stepper motor and two limit switches
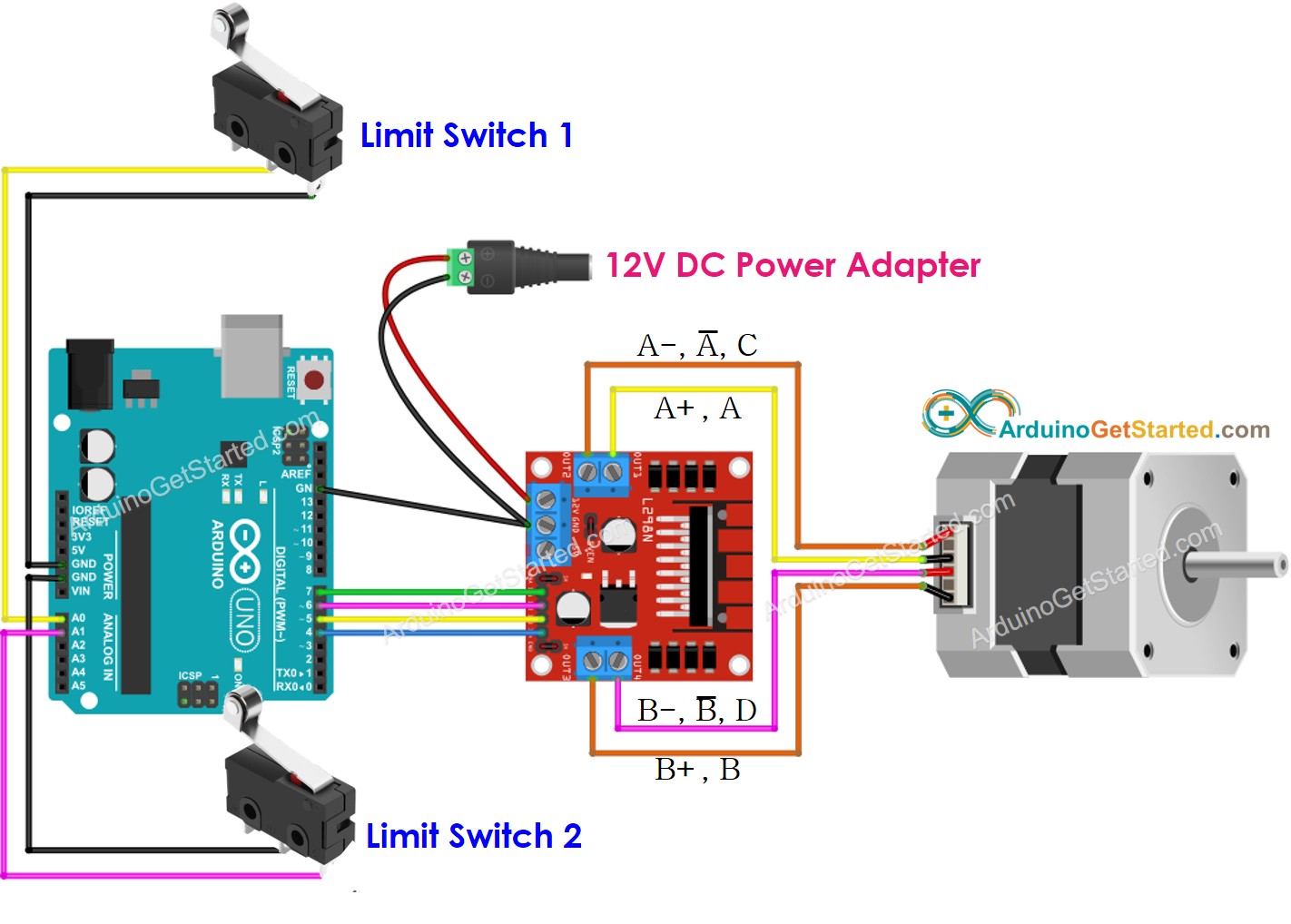
This image is created using Fritzing. Click to enlarge image
※ NOTE THAT:
Depending on the stepper motor, the wiring connection between the stepper motor and L298N may be different. Please take a close look at this Arduino - Stepper Motor tutorial to see how to connect the stepper motor to the L298N motor driver.
Arduino Code - Stop Stepper Motor by a Limit Switch
There are several ways to make a stepper motor stop:
- Call stepper.stop() function: This way does NOT stop the stepper motor immediately but gradually
- Do NOT call stepper.run() function: This way stops the stepper motor immediately
The below code make a stepper motor spin infinitely and stop immediately when a limit switch is touched
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezButton”, then find the button library by ArduinoGetStarted.com
- Click Install button to install ezButton library.
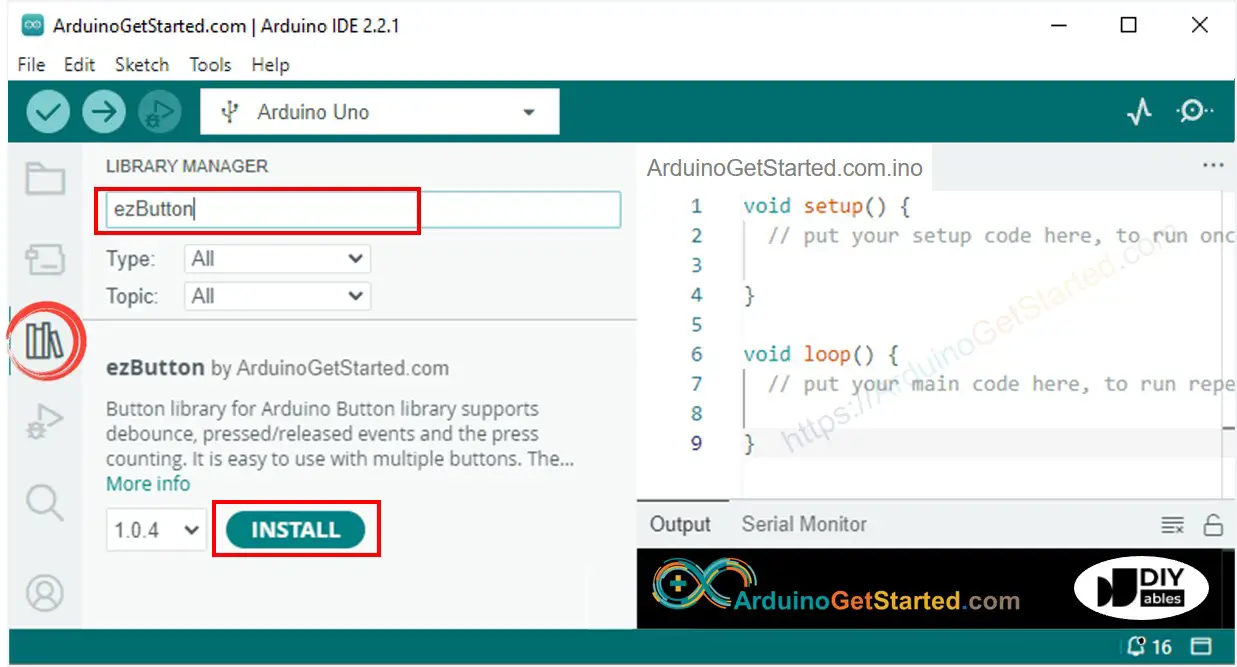
- Search “AccelStepper”, then find the AccelStepper library by Mike McCauley
- Click Install button to install AccelStepper library.
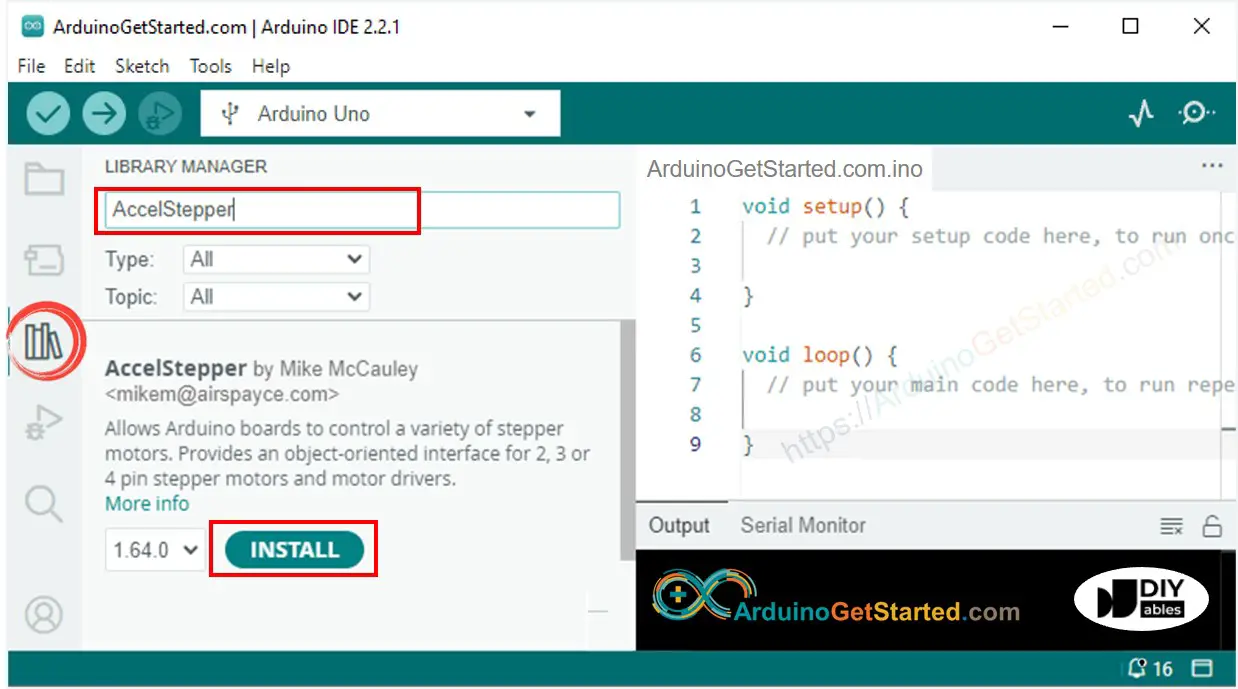
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- If the wiring is correct, you will see the motor rotates clockwise direction.
- Touch the limit switch
- You will see the motor is stopped immediately
- The result on Serial Monitor looks like below
Code Explanation
Read the line-by-line explanation in comment lines of code!
Arduino Code - Change Direction of Stepper Motor by a Limit Switch
The below code make a stepper motor spin infinitely and change its direction when a limit switch is touched
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- If the wiring is correct, you will see the motor spins in the clockwise direction.
- Touch the limit switch
- You will see the stepper motor's direction is changed to the anti-clockwise
- Touch the limit switch again
- You will see the stepper motor's direction is changed to clockwise
- The result on Serial Monitor looks like below
Arduino Code - Change Direction of Stepper Motor by two Limit Switches
The below code make a stepper motor spin infinitely and change its direction when one of two limit switches is touched
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- If the wiring is correct, you will see the motor spins in the clockwise direction.
- Touch the limit switch 1
- You will see the stepper motor's direction is changed to anti-clockwise
- Touch the limit switch 2
- You will see the stepper motor's direction is changed to clockwise
- The result on Serial Monitor looks like below
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.