Arduino - Servo Motor controlled by Potentiometer
In a previous tutorial, We have learned how a potentiometer triggers a servo motor. In this tutorial, We are going to learn how to rotate a servo motor according to the potentiometer's output value
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About Servo Motor and Potentiometer
If you do not know about servo motor and potentiometer (pinout, how it works, how to program ...), learn about them in the following tutorials:
Wiring Diagram
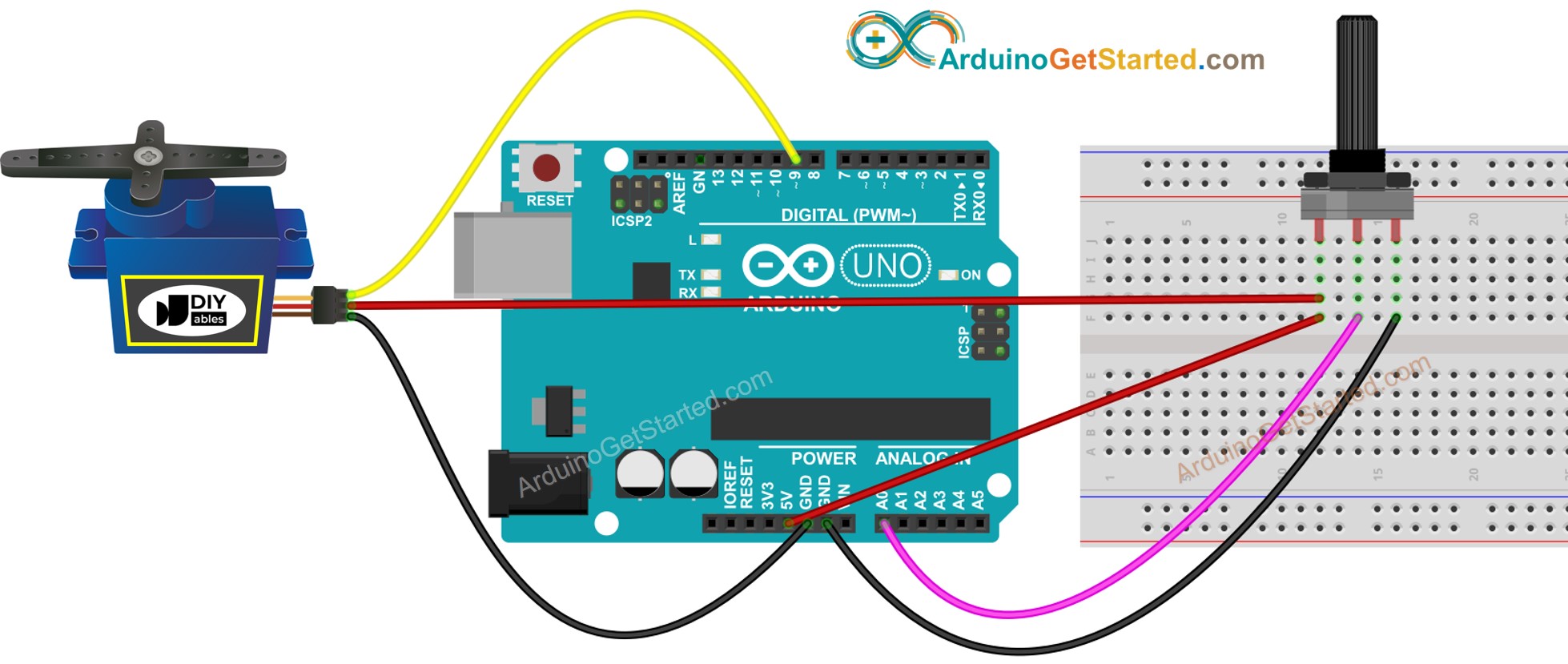
This image is created using Fritzing. Click to enlarge image
How To Program
- Reads the value of the potentiometer (value between 0 and 1023)
- Scales it to angle (value between 0 and 180)
- Sets the servo position according to the angle
Arduino Code
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
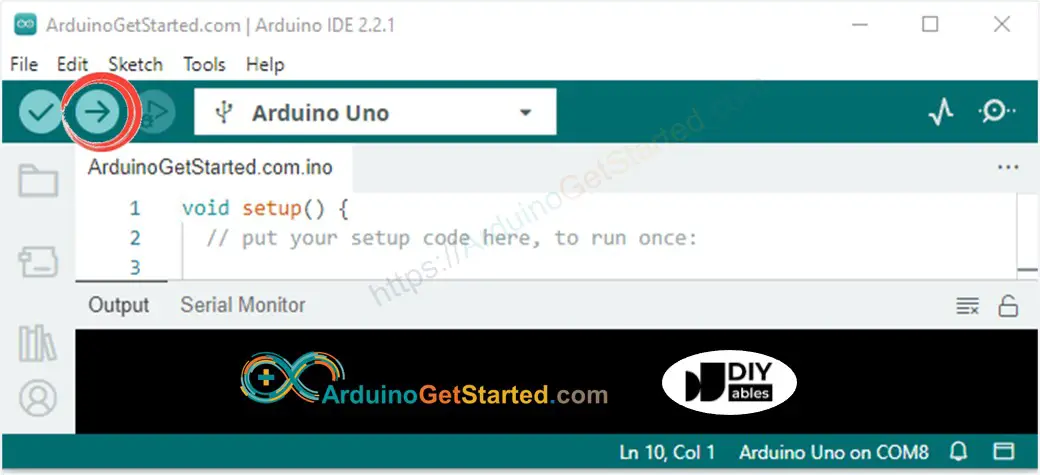
- Open Serial Monitor
- Rotate the potentiometer
- See the servo motor's rotation
- See the result on Serial Monitor
Code Explanation
Read the line-by-line explanation in comment lines of source code!
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.