ezAnalogKeypad Library - Analog Keypad Example
When your project uses a keypad but IO pins are not enough, you can use an Analog Keypad (three pins). The analog keypad allows you to use only a single analog input pin. This tutorials will teach you how to the analog keypad with Arduino. It also works with ESP32, ESP8266, or other platform.
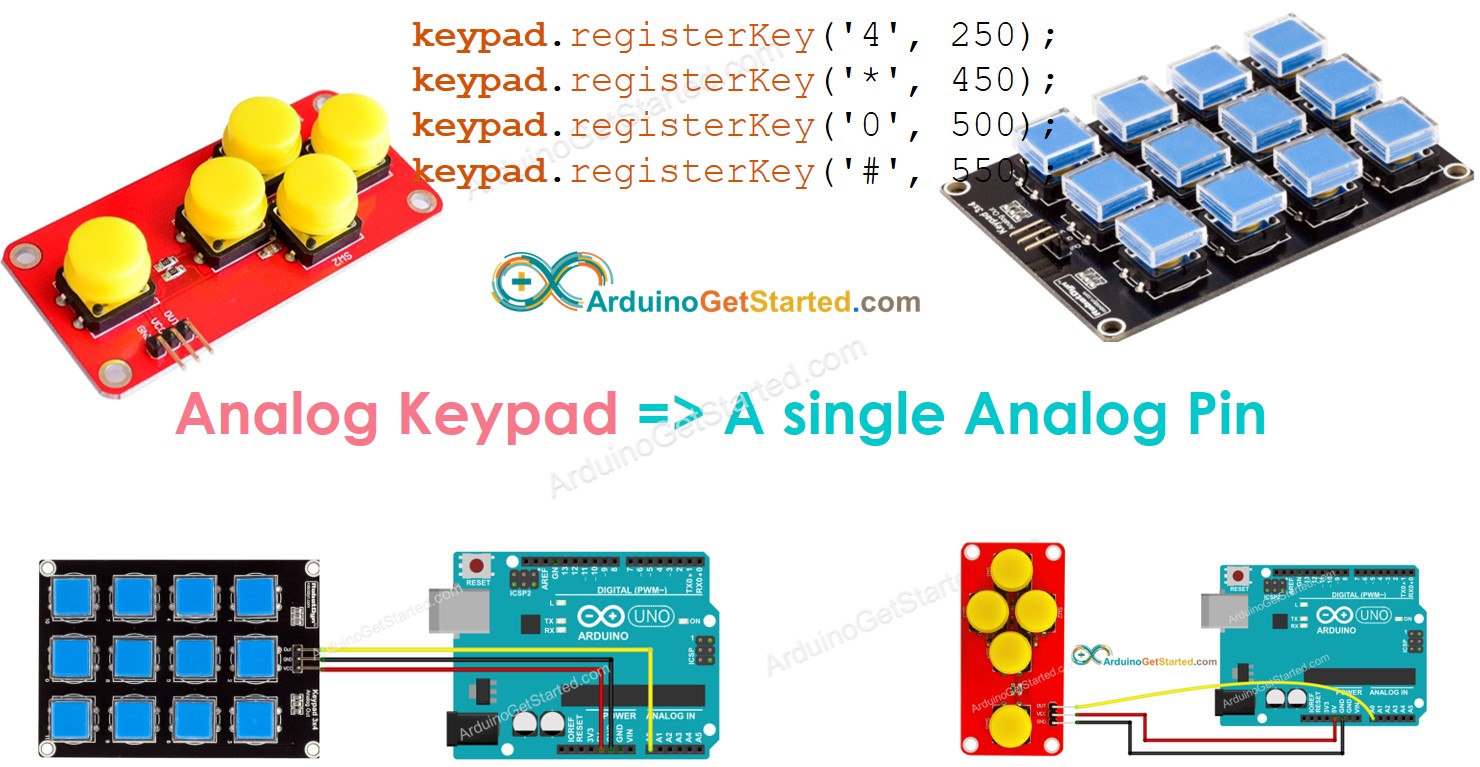
If you want to use the standard keypad, see this Arduino - Keypad tutorial
Hardware Required
Additionally, some links direct to products from our own brand, DIYables .
About ezAnalogKeypad Library
About Analog Keypad
Pinout
An analog keypad has three pins:
- VCC pin: connect this pin to VCC (5V or 3.3v)
- GND pin: connect this pin to GND (0V)
- Output pin: outputs the voltage according to the key press.
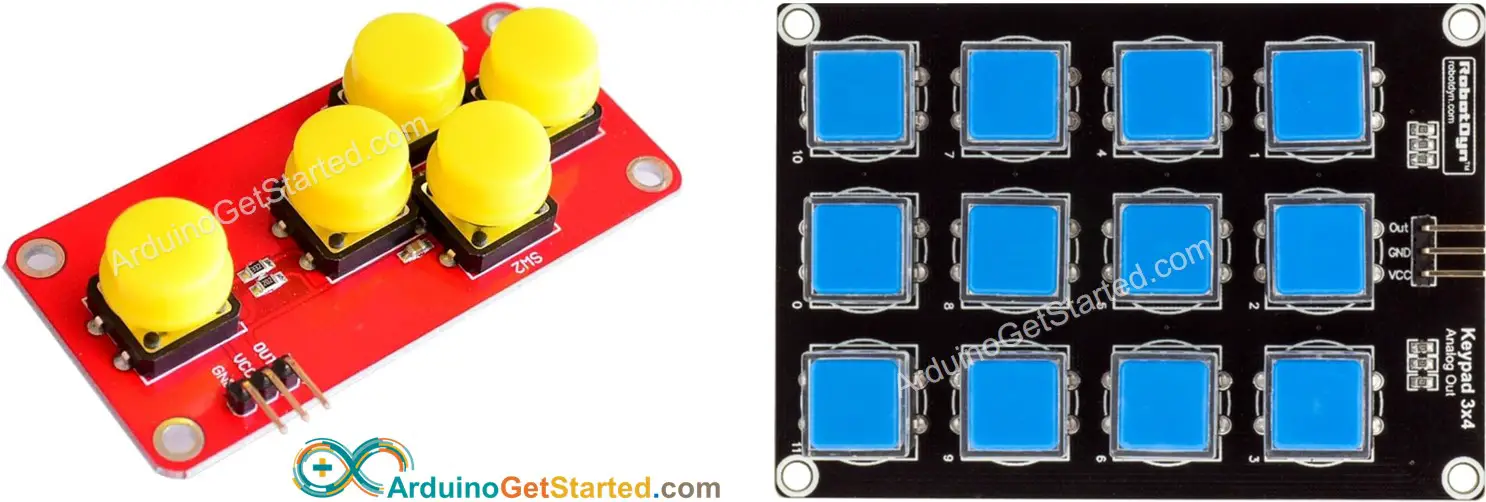
The analog keypad connects to a single analog input pin of Arduino, ESP32, ESP8266... When a key is pressed, the analog value is changed to a specific value. This value is diffrent from each key. The ezAnalogKeypad library reads the analog value determine which key is pressed by finding a key that has a nearest value.
⇒ What we needs to do first: determine the analog value for each key when it is pressed, and also the analog value when no key is pressed
The analog value when a key is pressed is depending on the following factors:
- How keys are wired
- The value of resistors
- The voltage supplies for the keypad
- The voltage reference of ADC
- The resolution of ADC (e.g. Arduino Uno is 10-bit ADC, ESP32 is 12-bit ADC)
⇒ The simplest way to find the analog values is run a test for callibration. By doing this way, we do not need to care about the above factors. We will learn how to do callibration in the next steps.
Wiring Diagram
- 12-key analog keypad (3x4)
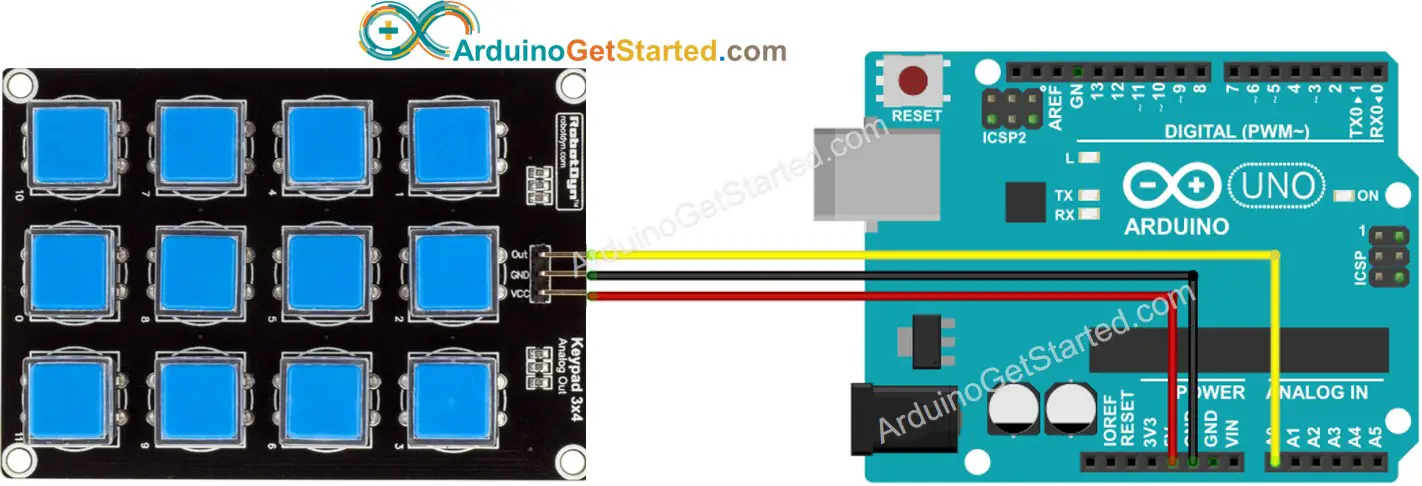
This image is created using Fritzing. Click to enlarge image
- 5-key analog keypad (12 key)
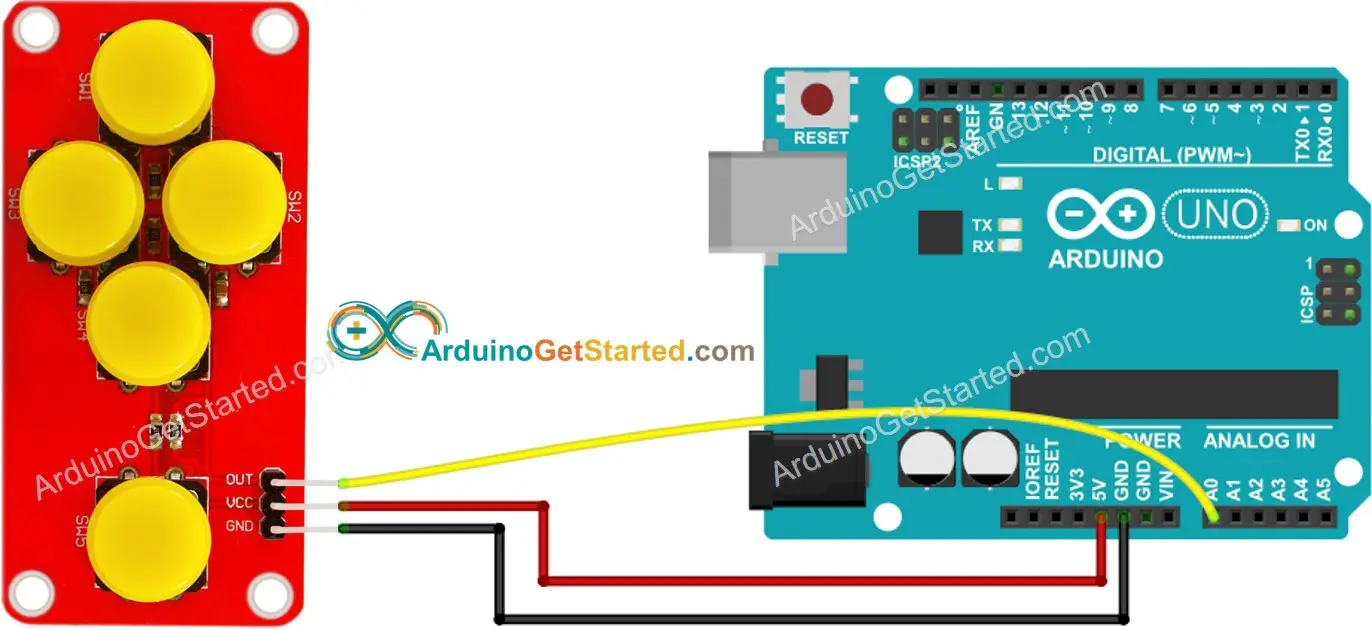
Arduino Code
Calibration
- Install ezAnalogKeypad library. See How To
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- On Arduino IDE, Go to File Examples ezAnalogKeypad Calibration example
- Click Upload button on Arduino IDE to upload code to Arduino
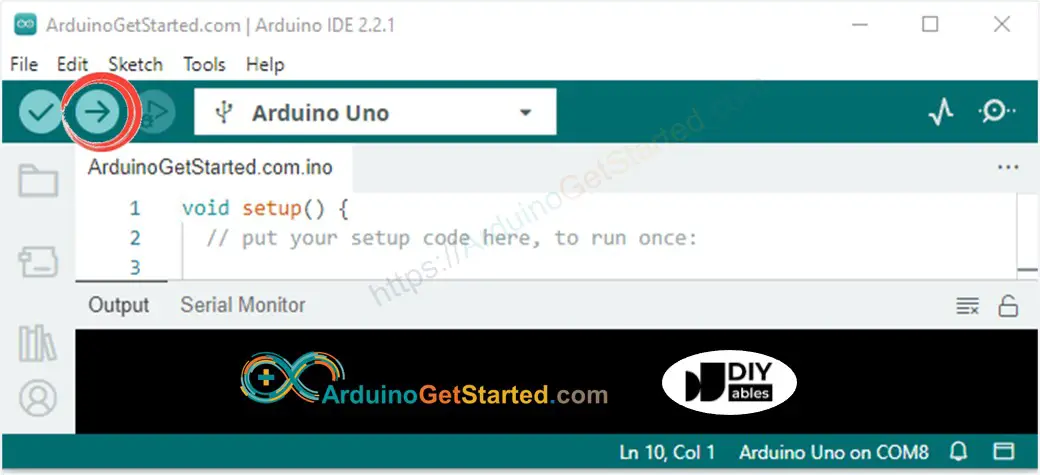
- See the output on Serial Monitor
- Write down the analog value when no key is pressed. This value will be put in keypad.setNoPressValue(value) later
- Press key one by one and write down the analog value for each key. These value will be put in keypad.registerKey(key, value) later
※ NOTE THAT:
analogValue can be fluctuated a little. You can choose a middle value. The library does not compare the analogValue equally. Instead, the library find the nearest match value.
Update the example code
- On Arduino IDE, Go to File Examples ezAnalogKeypad AnalogKeypad example
- Update the value you wrote down in the previous callibration process into the example code
- Click Upload button on Arduino IDE to upload code to Arduino
- Press key one by one
- See the result on Serial Monitor
Code Explanation
Read the line-by-line explanation in comment lines of source code!
※ NOTE THAT:
To save memory, the maximum keys can be registered is 20 by default. If you want to increase or decrease the maximum keys (to save more memory), define ezAnalogKeypad_MAX_KEY before including the library. For example: