Arduino - Temperature Sensor
There are many type of temperature sensors can works with Arduino such as LM35, TH02, HDC1000 or HTS221... In this tutorial, we are going to learn how to use waterproof DS18B20 temperature sensor with Arduino. This sensor is inexpensive, easy to use and look neat.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
Buy Note: Many DS18B20 sensors on the market are low-quality. We highly recommend buying the sensor from the DIYables brand using the link above. We tested it, and it worked well.
About One Wire Temperature Sensor - DS18B20
Pinout
DS18B20 temperature sensor has three pins:
- GND pin: needs to be connected to GND (0V)
- VCC pin: needs to be connected to VCC (5V or 3.3V)
- DATA pin: is 1-Wire Data bus. It should be connected to a digital pin on Arduino.
The sensor usually has two forms: TO-92 package (looks like a transistor) and waterproof probe. We use the waterproof probe form in this tutorial.
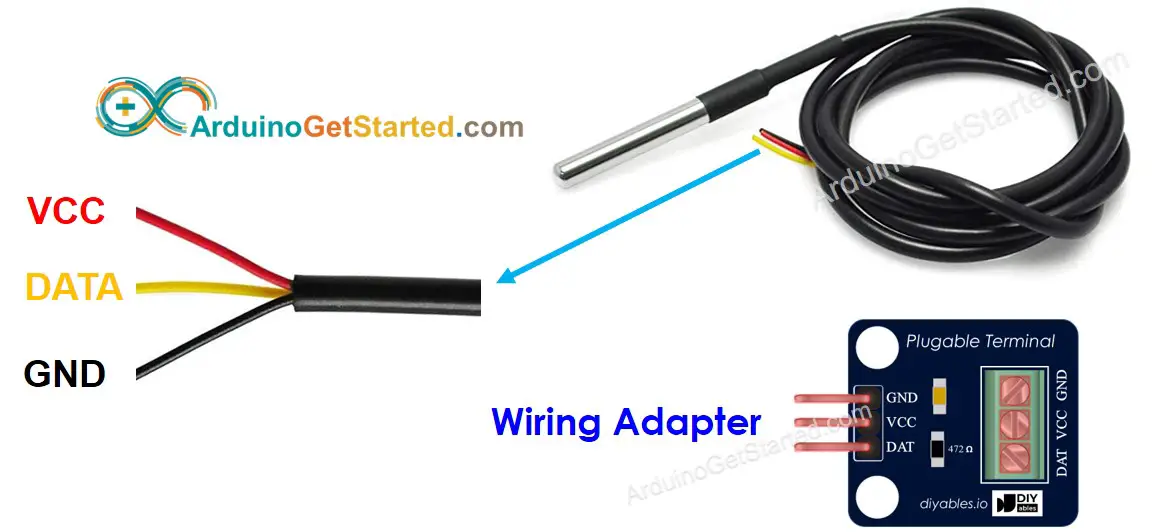
Connecting a DS18B20 temperature sensor to an Arduino requires a pull-up resistor, which can be a hassle. However, some manufacturers simplify the process by offering a wiring adapter that has a built-in pull-up resistor and a screw terminal block, making it much easier.
Wiring Diagram
- Wiring diagram with breadboard
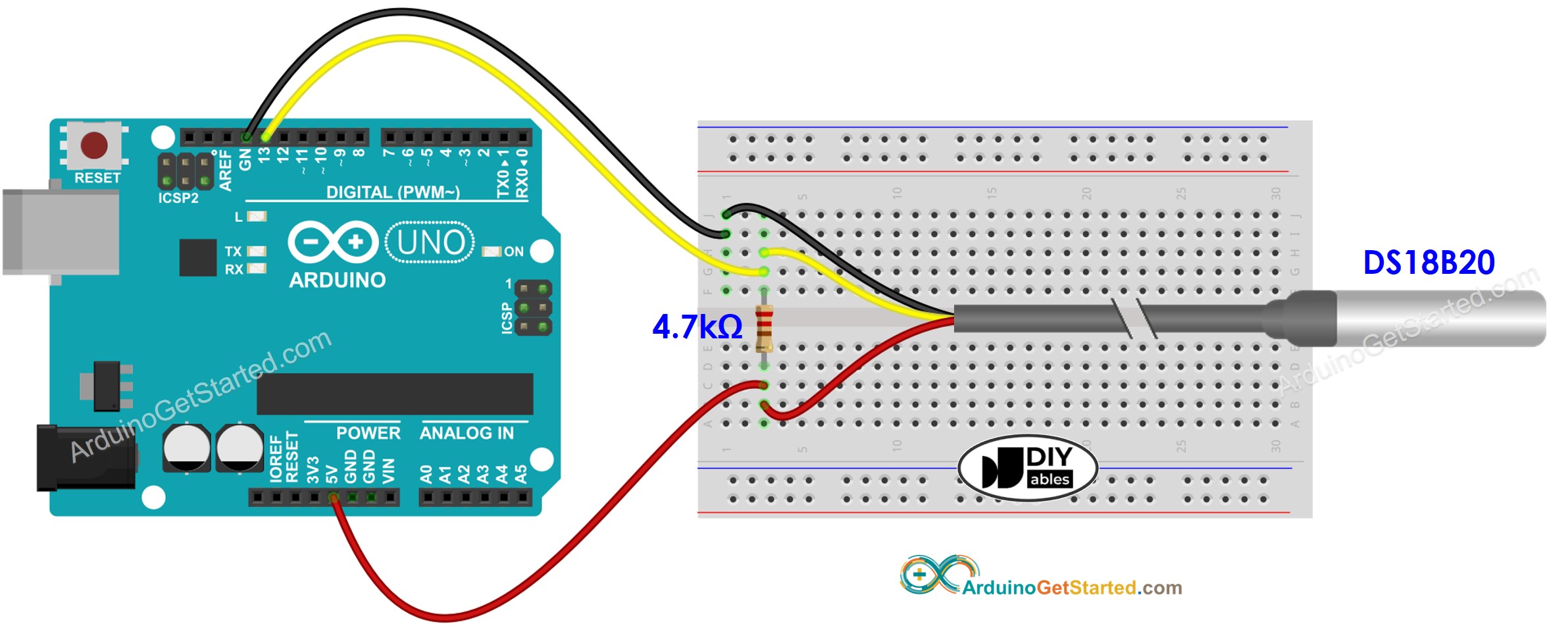
This image is created using Fritzing. Click to enlarge image
- Wiring diagram with adapter (recommended)
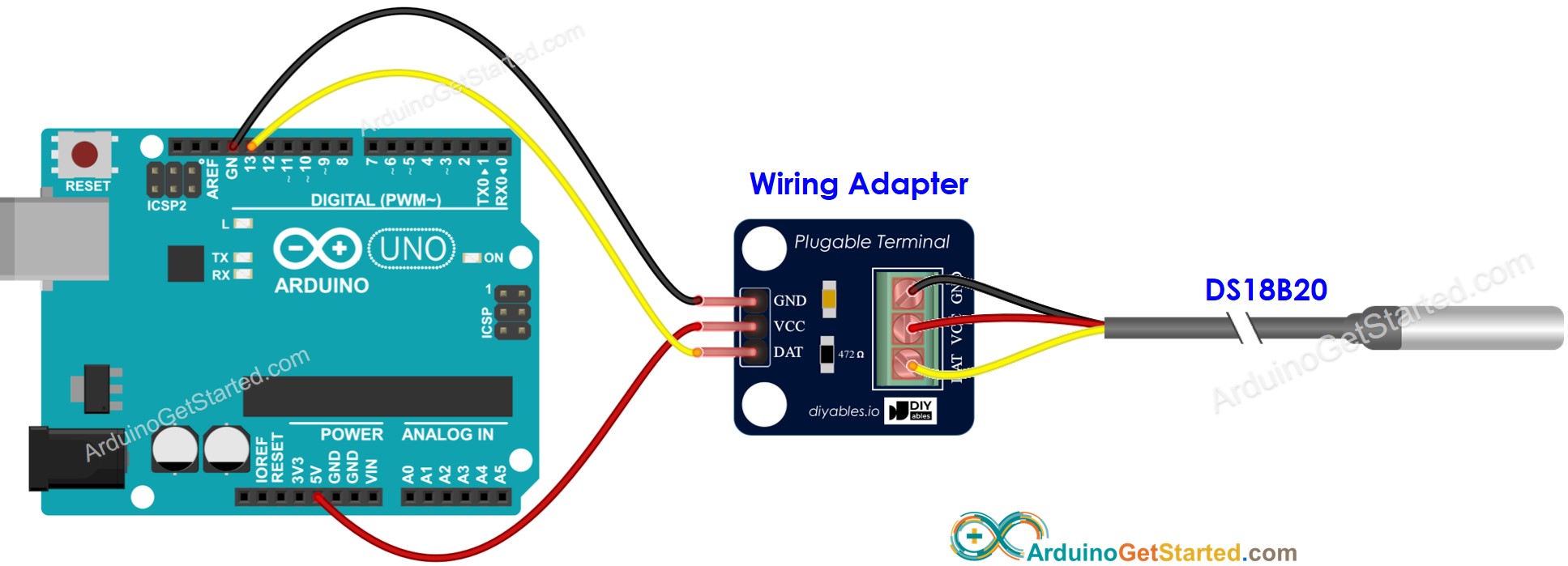
This image is created using Fritzing. Click to enlarge image
- Real wiring diagram with adapter
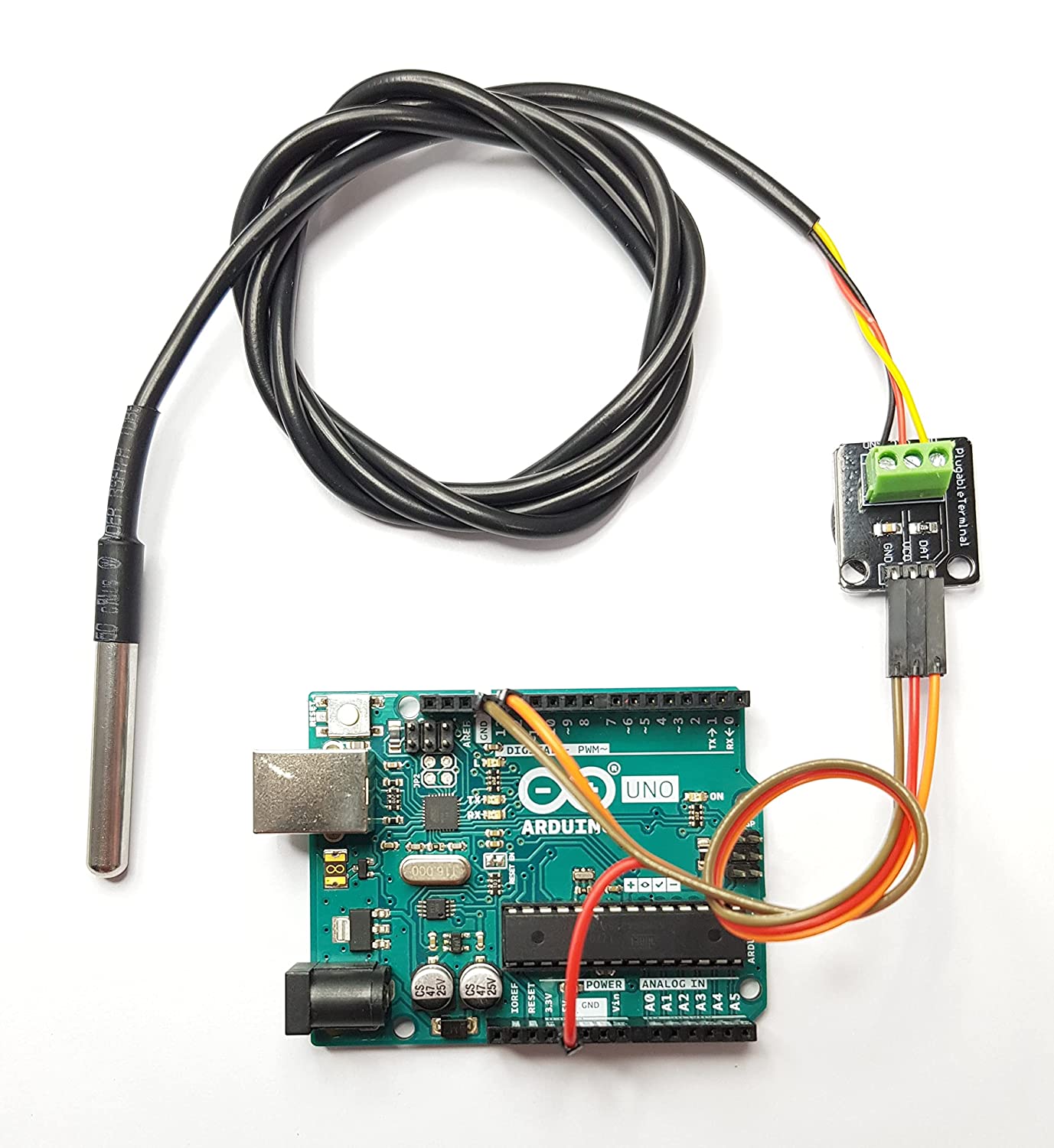
This image is created using Fritzing. Click to enlarge image
We suggest purchasing a DS18B20 sensor that comes with a wiring adapter for easy connection. The adapter has a built-in resistor, eliminating the need for a separate one in the wiring.
How To Program For DS18B20 Temperature Sensor
- Include the library:
- Declare OneWire and DallasTemperature object corresponding to the pin connected to sensor's DATA pin
- Initialize the sensor:
- Send the command to get temperatures:
- Read temperature in Celsius:
- (Optional) Convert Celsius to Fahrenheit:
Arduino Code
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “DallasTemperature”, then find the DallasTemperature library by Miles Burton.
- Click Install button to install DallasTemperature library.
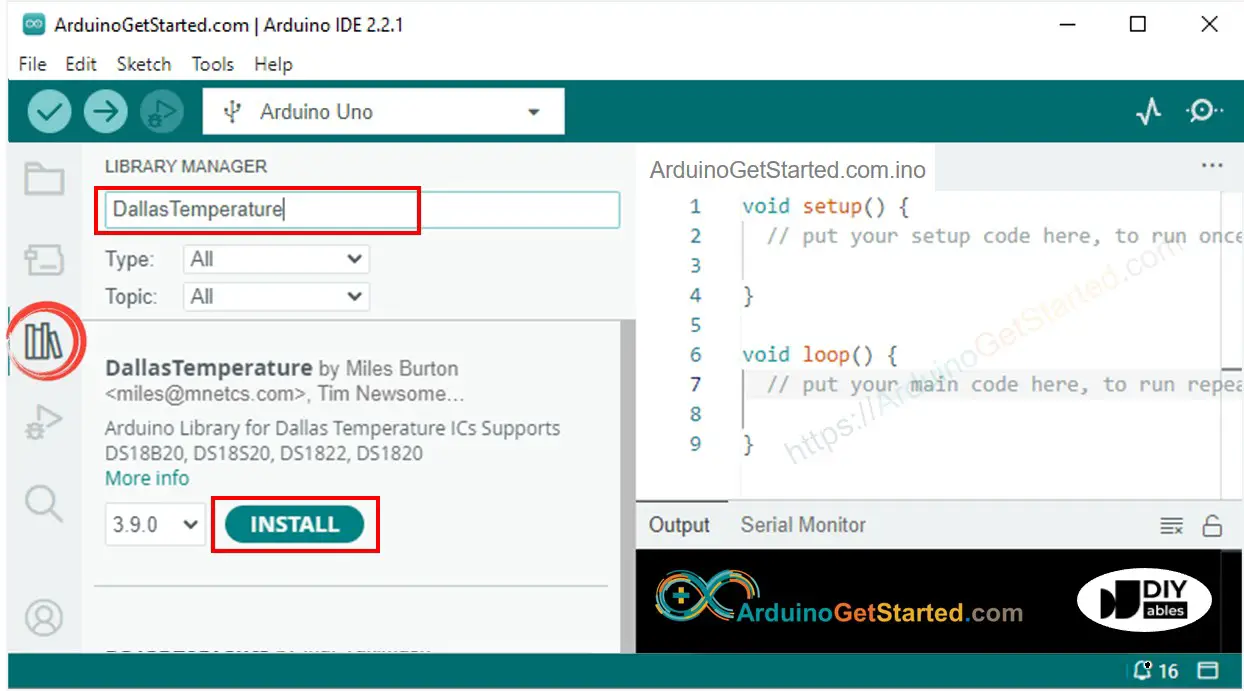
- You will be asked to install the library dependency
- Click Install All button to install OneWire library.
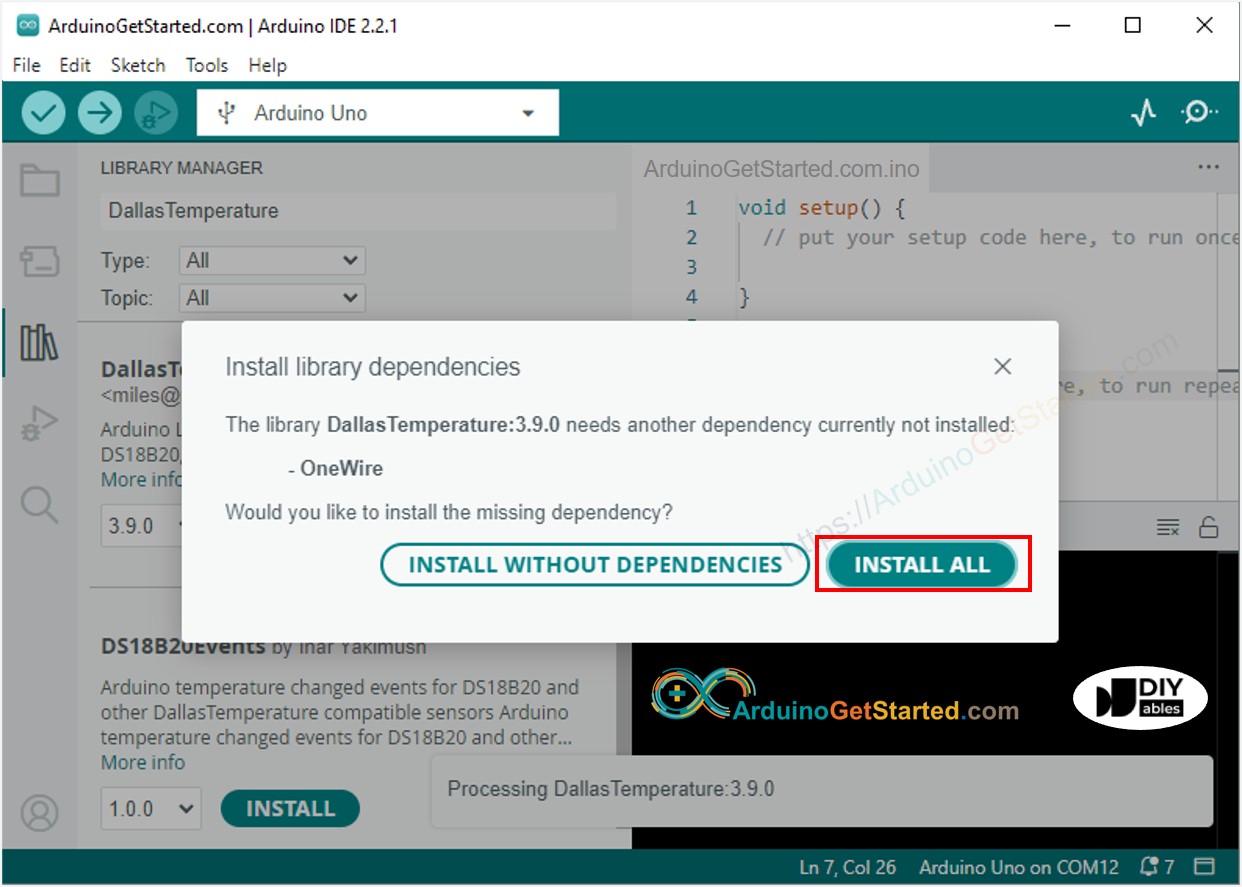
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Put the sensor on hot and cold water, or grasp the sensor by your hand
- See the result on Serial Monitor.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.