Arduino - OLED Clock
In this tutorial, we are going to learn how to make OLED clock by:
- Reading time (hour, minute, second) from DS3231 RTC module and display it on an OLED
- Reading time (hour, minute, second) from DS1307 RTC module and display it on an OLED
You can choose one of two RTC modules: DS3231 and DS1307. See DS3231 vs DS1307
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables .
Additionally, some links direct to products from our own brand, DIYables .
About OLED, DS3231 and DS1307 RTC module
If you do not know about OLED, DS3231 and DS1307 (pinout, how it works, how to program ...), learn about them in the following tutorials:
Install OLED and RTC Libraries
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “SSD1306”, then find the SSD1306 library by Adafruit
- Click Install button to install the library.
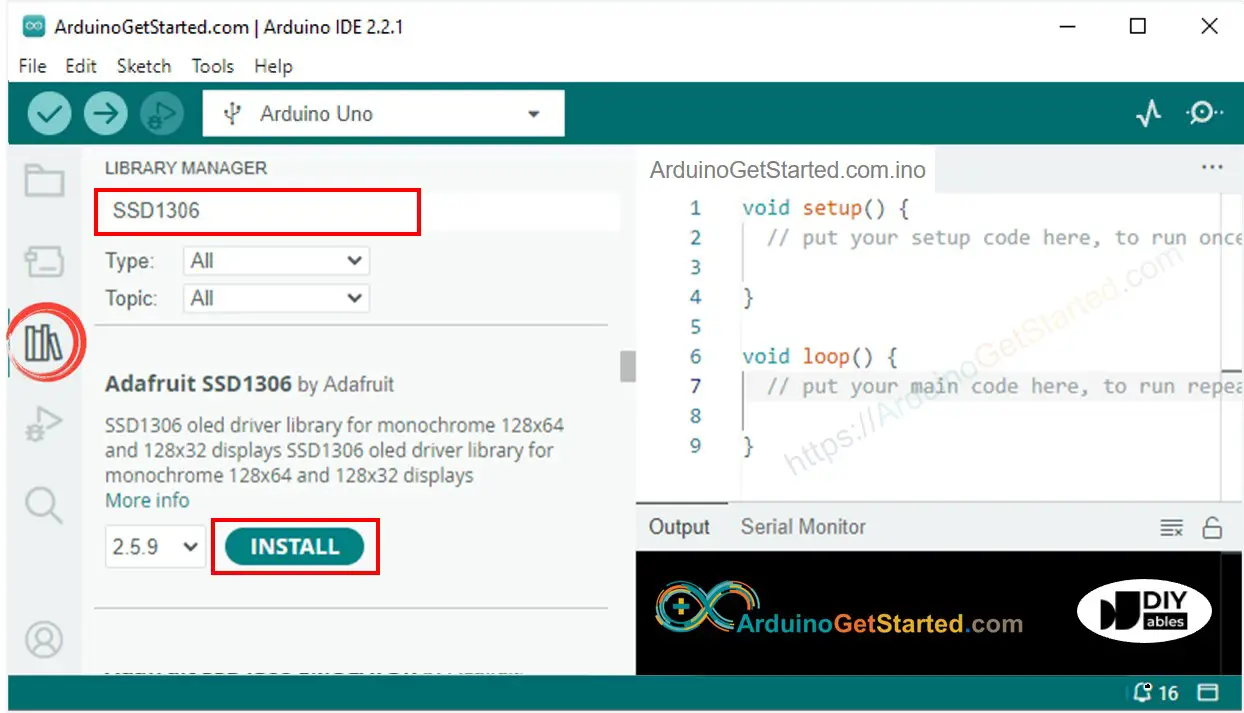
- You will be asked for intalling some other library dependencies
- Click Install All button to install all library dependencies.
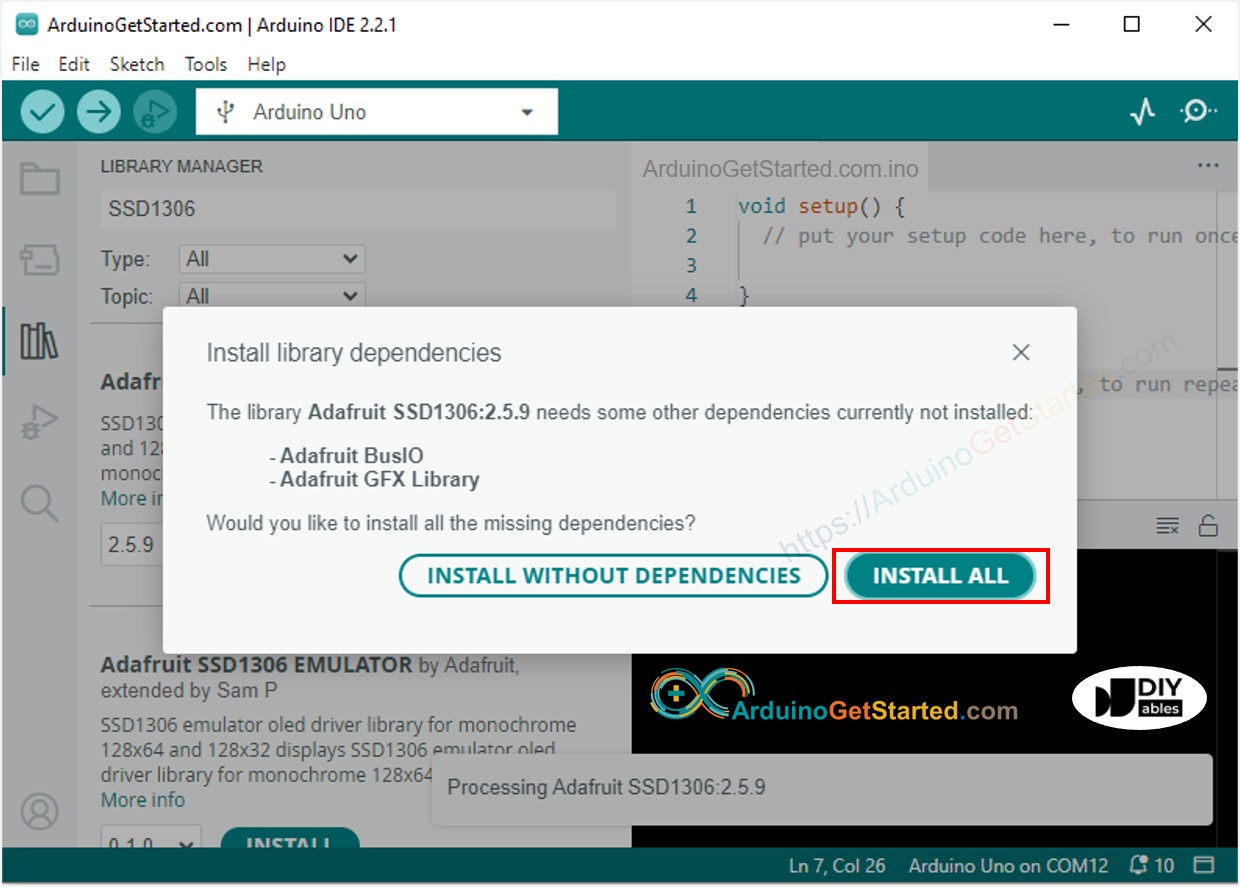
- Search “RTClib”, then find the RTC library by Adafruit. This library works with both DS3231 and DS1307
- Click Install button to install RTC library.
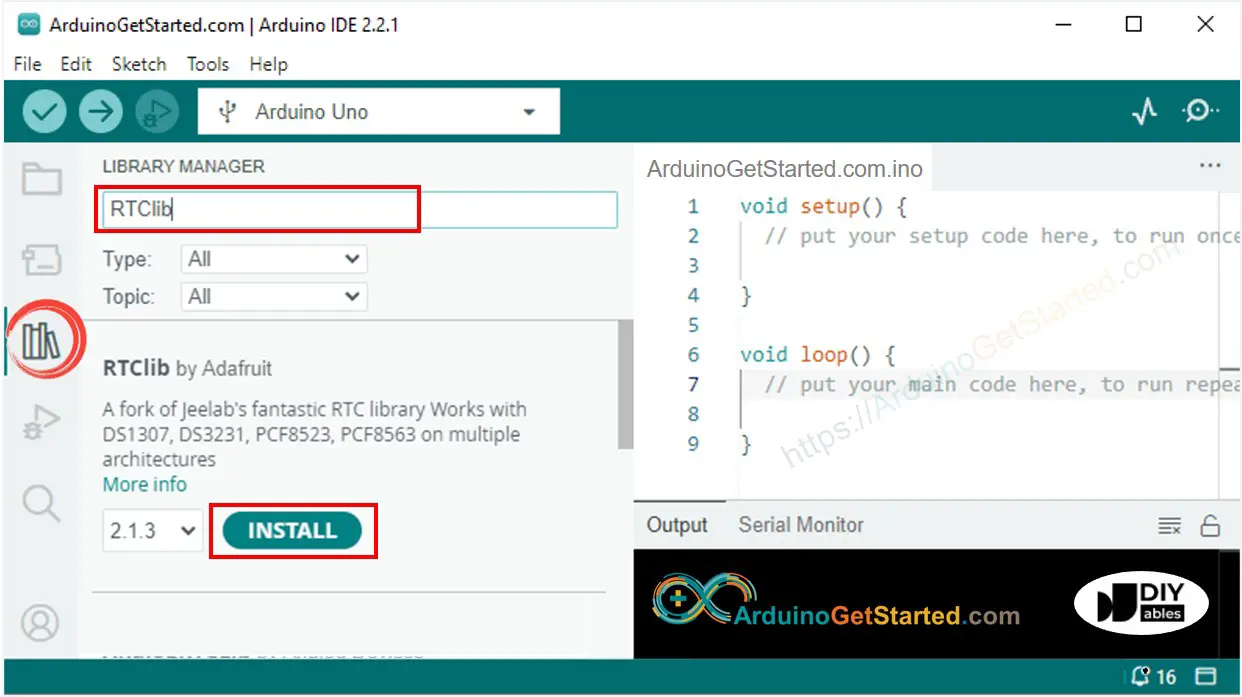
- You may be asked for intalling some other library dependencies
- Click Install All button to install all library dependencies.
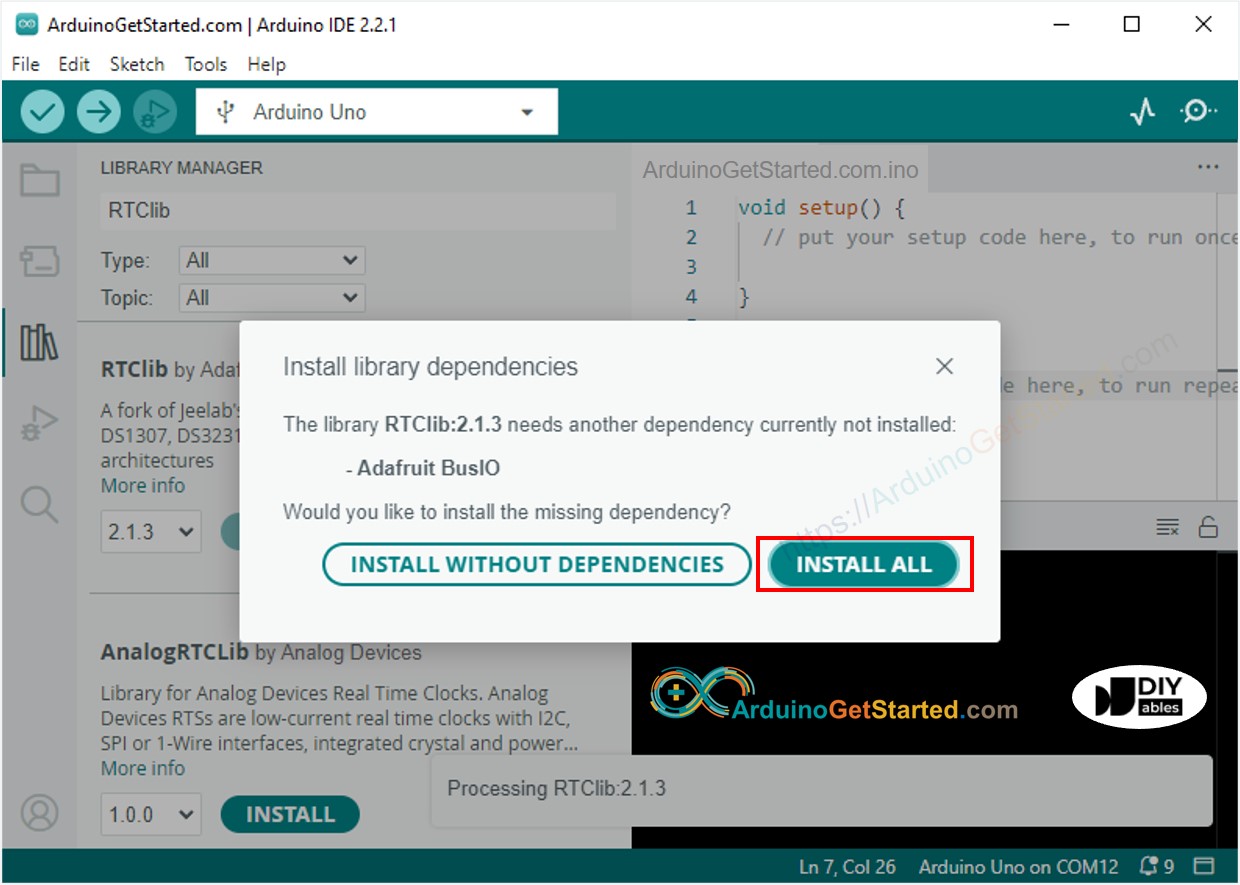
Reading time from DS3231 RTC module and display it on OLED
Wiring Diagram
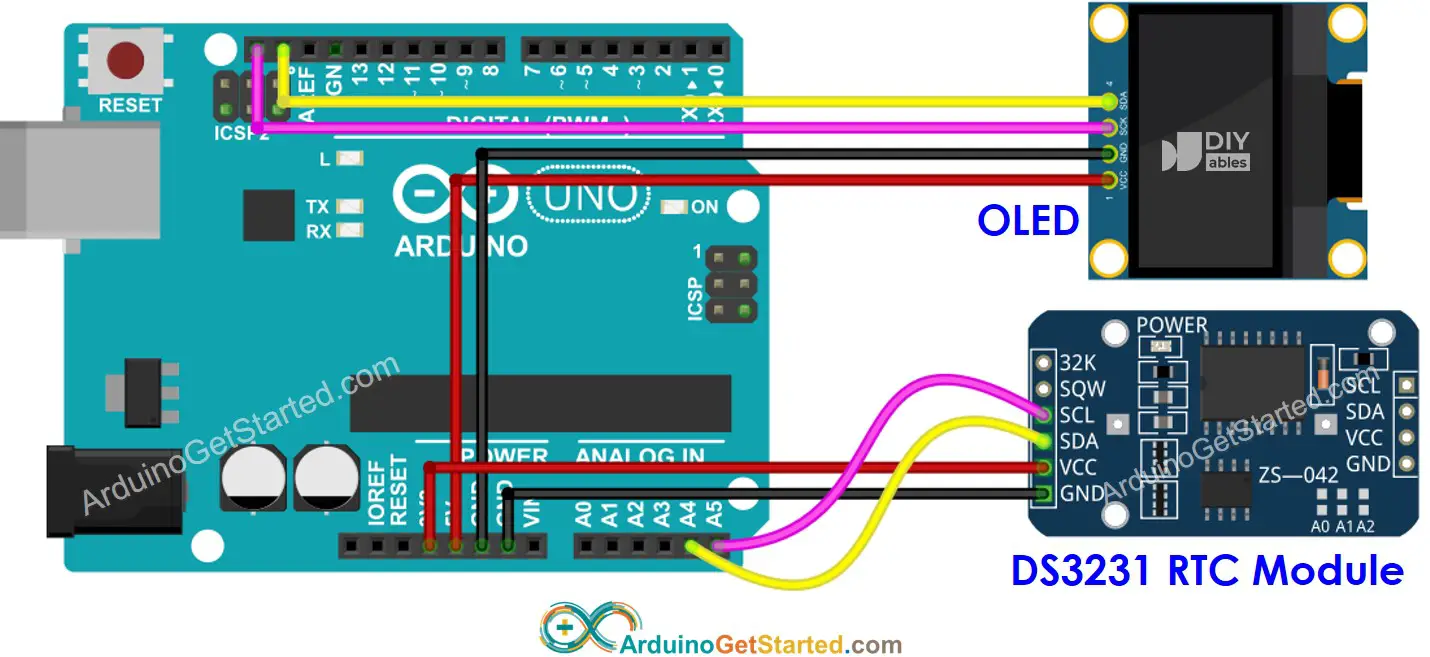
This image is created using Fritzing. Click to enlarge image
Arduino Code - DS3231 and OLED
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/tutorials/arduino-oled-clock
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <RTClib.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // // create SSD1306 display object connected to I2C
RTC_DS3231 rtc;
String time;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(1); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
// SETUP RTC MODULE
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (true);
}
// automatically sets the RTC to the date & time on PC this sketch was compiled
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
time.reserve(10); // to avoid fragmenting memory when using String
}
void loop() {
DateTime now = rtc.now();
time = "";
time += now.hour();
time += ':';
time += now.minute();
time += ':';
time += now.second();
oledDisplayCenter(time);
}
void oledDisplayCenter(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// display on horizontal and vertical center
oled.clearDisplay(); // clear display
oled.setCursor((SCREEN_WIDTH - width) / 2, (SCREEN_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See the result on OLED
Reading time from DS1307 RTC module and display it on OLED
Wiring Diagram
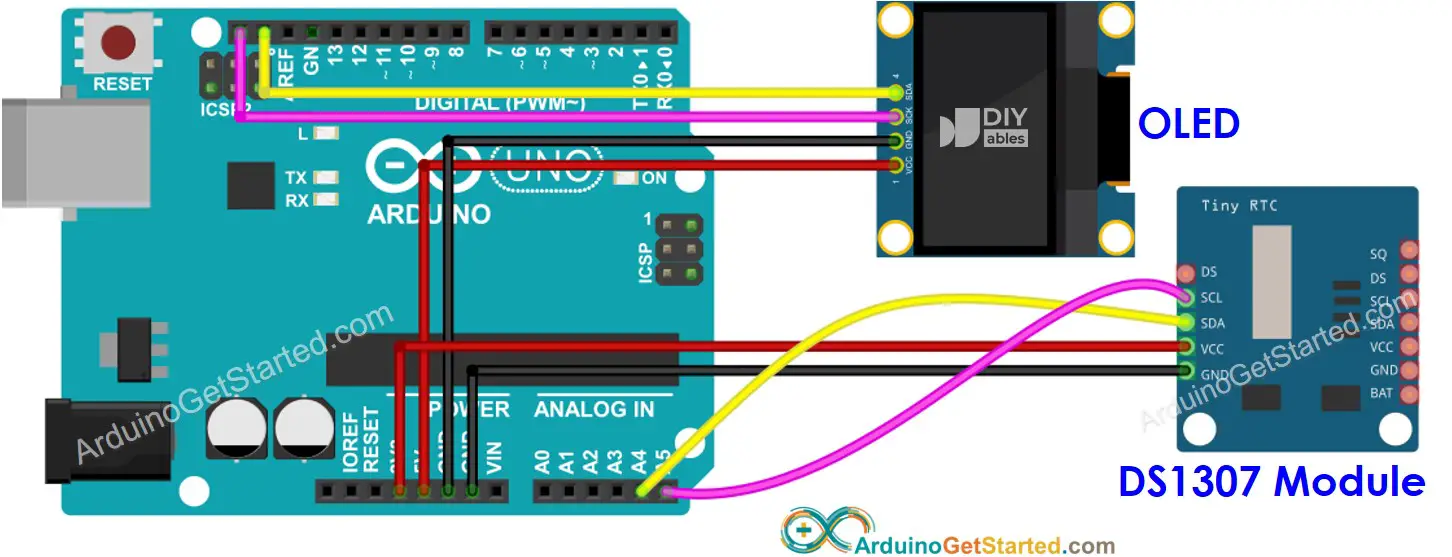
This image is created using Fritzing. Click to enlarge image
Arduino Code - DS1307 and OLED
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/tutorials/arduino-oled-clock
*/
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <RTClib.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // // create SSD1306 display object connected to I2C
RTC_DS1307 rtc;
String time;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(1); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
// SETUP RTC MODULE
if (! rtc.begin()) {
Serial.println("Couldn't find RTC");
Serial.flush();
while (true);
}
// automatically sets the RTC to the date & time on PC this sketch was compiled
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
time.reserve(10); // to avoid fragmenting memory when using String
}
void loop() {
DateTime now = rtc.now();
time = "";
time += now.hour();
time += ':';
time += now.minute();
time += ':';
time += now.second();
oledDisplayCenter(time);
}
void oledDisplayCenter(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// display on horizontal and vertical center
oled.clearDisplay(); // clear display
oled.setCursor((SCREEN_WIDTH - width) / 2, (SCREEN_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See the result on OLED
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.