Arduino - LED Matrix via Web
In this tutorial, we are going to learn how to control an LED Matrix through a web interface using a browser on a PC or smartphone, utilizing the Arduino Uno R4 WiFi. In detail, the Arduino Uno R4 WiFi will be programmed to work as a web server. Let's assume that the IP address of the Arduino Uno R4 WiFi is 192.168.0.2. Here are the details of how it works:
- When you enter 192.168.0.2 into the web browser, the browser sends a request to the Arduino, and the Arduino responds with a web page that contains a message box.
- You type a message to the message box and click the send button. The message is sent to Arduino.
- Arduino displays the message on the LED Matrix.
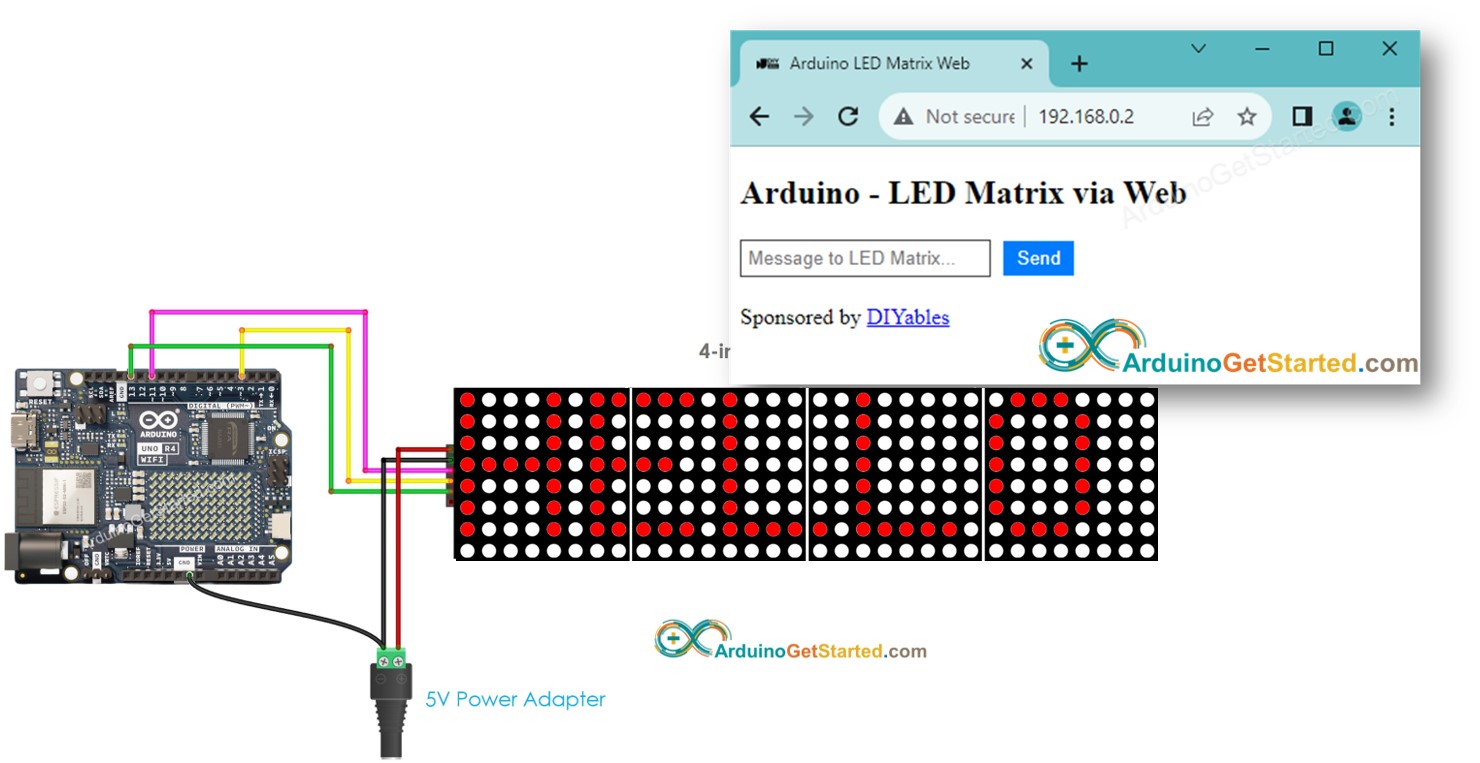
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand, DIYables .
Additionally, some links direct to products from our own brand, DIYables .
About LED Matrix and Arduino Uno R4
If you do not know about LED Matrix and Arduino Uno R4 (pinout, how it works, how to program ...), learn about them in the following tutorials:
Wiring Diagram
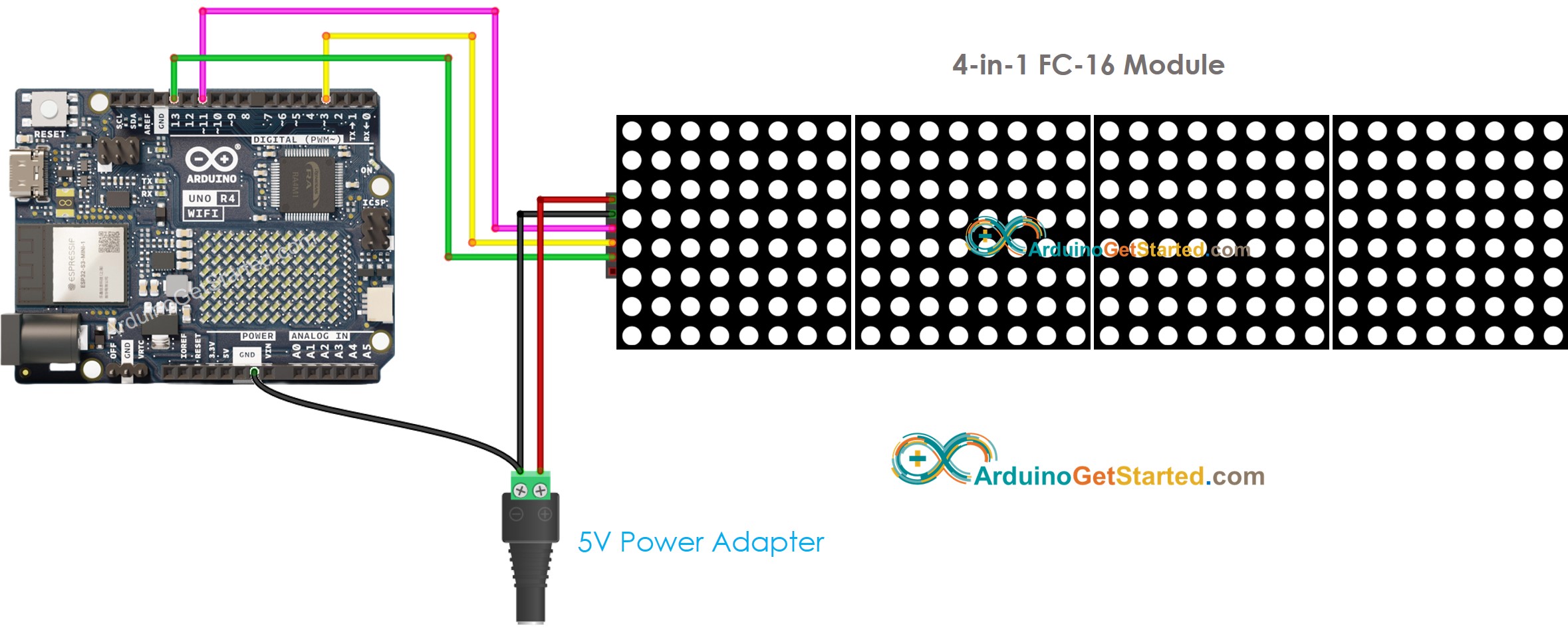
This image is created using Fritzing. Click to enlarge image
Arduino Code
Quick Steps
- If this is the first time you use Arduino Uno R4, see how to setup environment for Arduino Uno R4 on Arduino IDE.
- Open the Arduino IDE.
- Search “MD_Parola”, then find the MD_Parola library
- Click Install button.
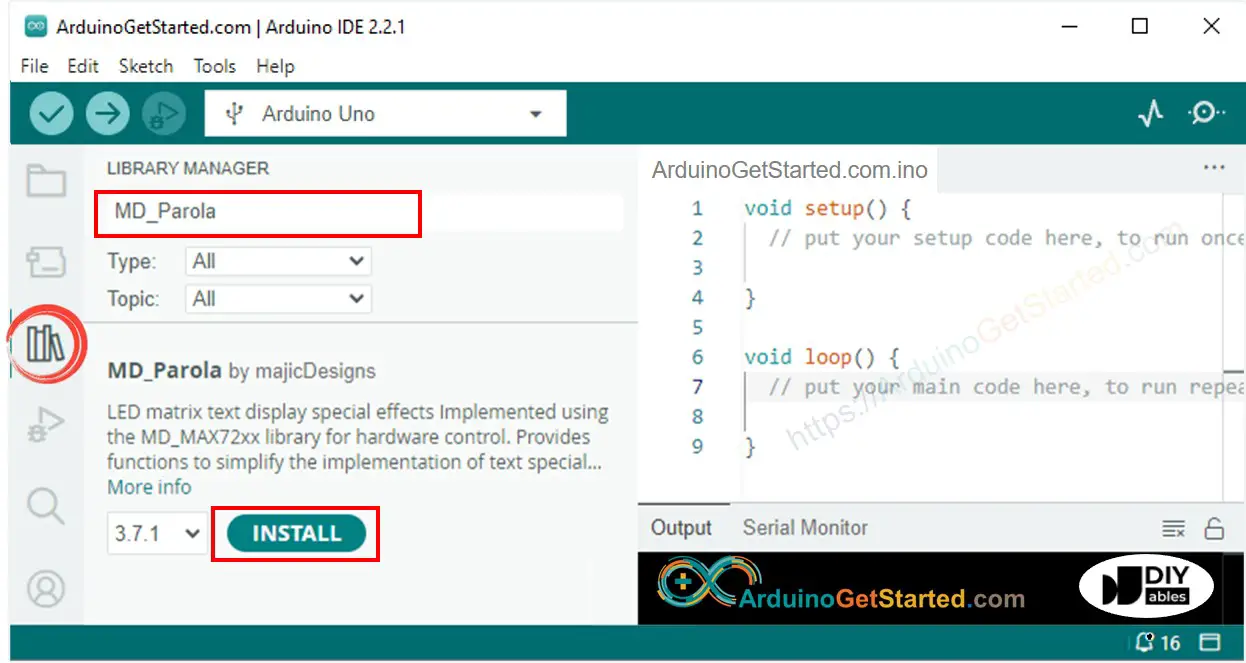
- You will be asked to install the MD_MAX72XX library for dependency. Click Install All button.
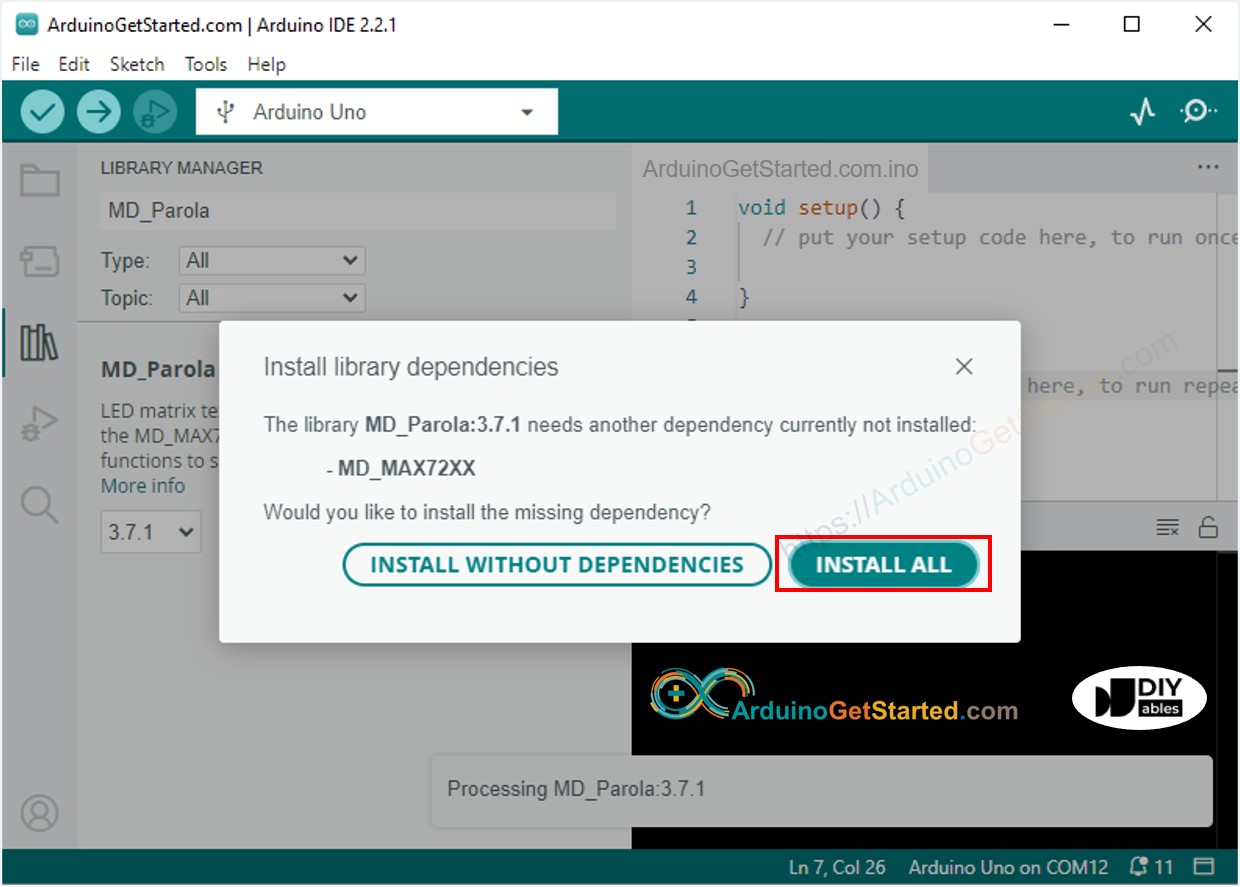
- Create a new sketch. Give it a name, for example, ArduinoGetStarted.com.ino.
- Copy the code provided below and paste it to the created file.
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/tutorials/arduino-led-matrix-via-web
*/
#include <WiFiS3.h>
#include <MD_Parola.h>
#include <MD_MAX72xx.h>
#include "index.h"
#define HARDWARE_TYPE MD_MAX72XX::FC16_HW
#define MAX_DEVICES 4 // 4 blocks
#define CS_PIN 3
// create an instance of the MD_Parola class
MD_Parola ledMatrix = MD_Parola(HARDWARE_TYPE, CS_PIN, MAX_DEVICES);
const char ssid[] = "YOUR_WIFI_SSID"; // change your network SSID (name)
const char pass[] = "YOUR_WIFI_PASSWORD"; // change your network password (use for WPA, or use as key for WEP)
int status = WL_IDLE_STATUS;
WiFiServer server(80);
void setup() {
//Initialize serial and wait for port to open:
Serial.begin(9600);
ledMatrix.begin(); // initialize the object
ledMatrix.setIntensity(15); // set the brightness of the LED matrix display (from 0 to 15)
ledMatrix.displayClear(); // clear led matrix display
ledMatrix.displayScroll("Hello, DIYables", PA_CENTER, PA_SCROLL_LEFT, 100);
String fv = WiFi.firmwareVersion();
if (fv < WIFI_FIRMWARE_LATEST_VERSION) {
Serial.println("Please upgrade the firmware");
}
// attempt to connect to WiFi network:
while (status != WL_CONNECTED) {
Serial.print("Attempting to connect to SSID: ");
Serial.println(ssid);
// Connect to WPA/WPA2 network. Change this line if using open or WEP network:
status = WiFi.begin(ssid, pass);
// wait 10 seconds for connection:
delay(10000);
}
server.begin();
// you're connected now, so print out the status:
printWifiStatus();
}
void loop() {
// listen for incoming clients
WiFiClient client = server.available();
if (client) {
// read the first line of HTTP request header
String HTTP_req = "";
while (client.connected()) {
if (client.available()) {
Serial.println("New HTTP Request");
HTTP_req = client.readStringUntil('\n'); // read the first line of HTTP request
Serial.print("<< ");
Serial.println(HTTP_req); // print HTTP request to Serial Monitor
break;
}
}
// read the remaining lines of HTTP request header
while (client.connected()) {
if (client.available()) {
String HTTP_header = client.readStringUntil('\n'); // read the header line of HTTP request
if (HTTP_header.equals("\r")) // the end of HTTP request
break;
Serial.print("<< ");
Serial.println(HTTP_header); // print HTTP request to Serial Monitor
}
}
if (HTTP_req.indexOf("GET") == 0) { // check if request method is GET
// expected header is one of the following:
// - GET ?/message= "user message"
if (HTTP_req.indexOf("message=") > -1) { // check the path
int pos_1 = HTTP_req.indexOf("message=") + 8; // 8 is the lengh of "message="
int pos_2 = HTTP_req.indexOf(" ", pos_1);
String message = HTTP_req.substring(pos_1, pos_2);
Serial.print("message: ");
Serial.println(message);
ledMatrix.displayClear(); // clear led matrix display
ledMatrix.displayScroll(message.c_str(), PA_CENTER, PA_SCROLL_LEFT, 100);
}
}
// send the HTTP response
// send the HTTP response header
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close"); // the connection will be closed after completion of the response
client.println(); // the separator between HTTP header and body
// send the HTTP response body
String html = String(HTML_CONTENT);
client.println(html);
client.flush();
// give the web browser time to receive the data
delay(10);
// close the connection:
client.stop();
}
if (ledMatrix.displayAnimate()) {
ledMatrix.displayReset();
}
}
void printWifiStatus() {
// print your board's IP address:
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
// print the received signal strength:
Serial.print("signal strength (RSSI):");
Serial.print(WiFi.RSSI());
Serial.println(" dBm");
}
- Change the WiFi information (SSID and password) in the code to yours
- Create the index.h file On Arduino IDE by:
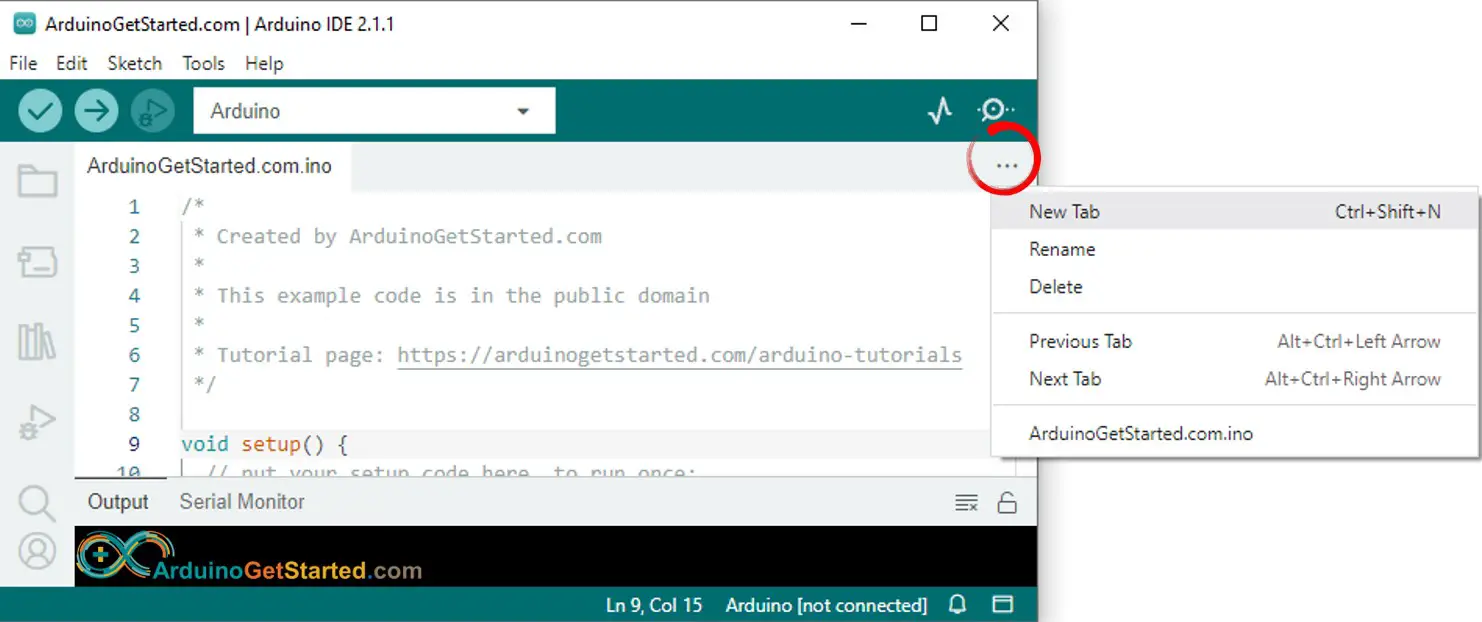
- Either click on the button just below the serial monitor icon and choose New Tab, or use Ctrl+Shift+N keys.
- Give file's name index.h and click OK button
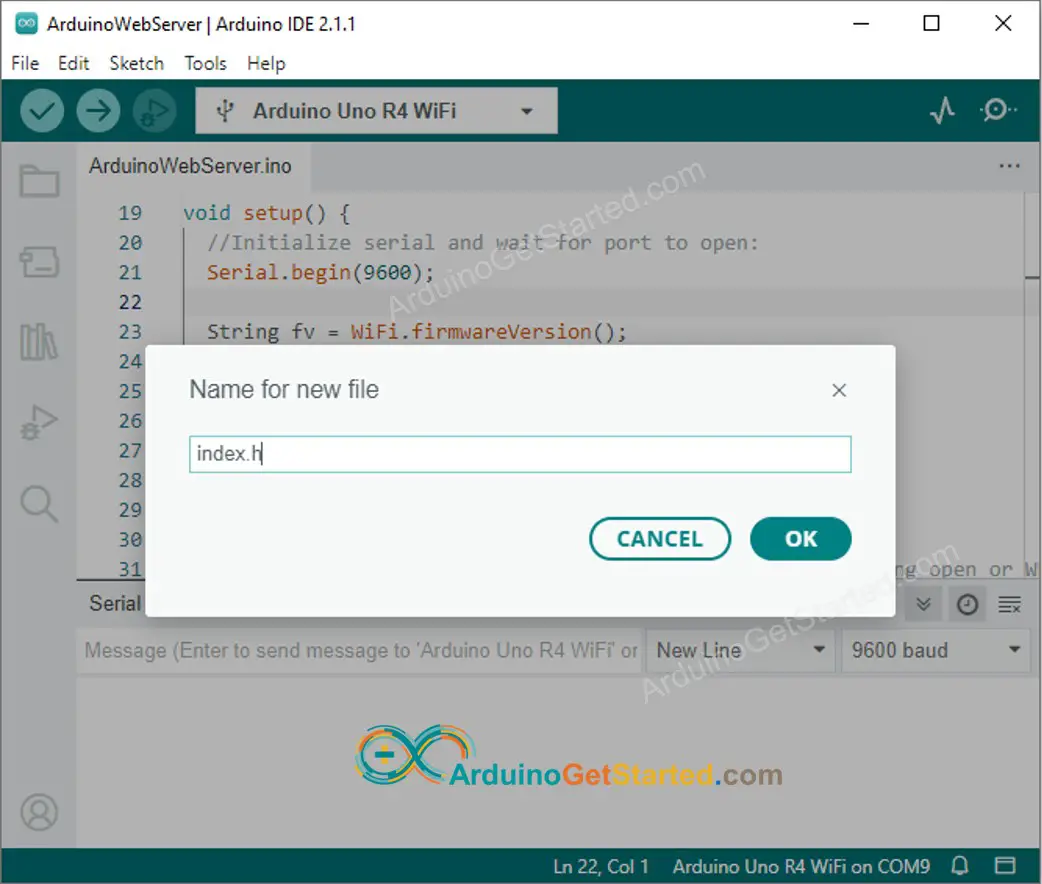
- Copy the below code and paste it to the index.h.
/*
* Created by ArduinoGetStarted.com
*
* This example code is in the public domain
*
* Tutorial page: https://arduinogetstarted.com/tutorials/arduino-led-matrix-via-web
*/
const char *HTML_CONTENT = R"=====(
<!DOCTYPE html>
<html>
<head>
<title>Arduino LED Matrix Web</title>
<meta name="viewport" content="width=device-width, initial-scale=0.7">
<link rel="icon" href="https://diyables.io/images/page/diyables.svg">
<style>
body {
font-size: 16px;
}
.user-input {
margin-bottom: 20px;
}
.user-input input {
flex: 1;
border: 1px solid #444;
padding: 5px;
}
.user-input input[type="submit"] {
margin-left: 5px;
background-color: #007bff;
color: #fff;
border: none;
padding: 5px 10px;
cursor: pointer;
}
</style>
</head>
<body>
<h2>Arduino - LED Matrix via Web</h2>
<form class="user-input" action="" method="GET">
<input type="text" id="message" name="message" placeholder="Message to LED Matrix...">
<input type="submit" value="Send">
</form>
<div class="sponsor">Sponsored by <a href="https://amazon.com/diyables">DIYables</a></div>
</body>
</html>
)=====";
- Now you have the code in two files: ArduinoGetStarted.com.ino and index.h
- Click Upload button on Arduino IDE to upload code to Arduino
- See the result on Serial Monitor.
COM6
Attempting to connect to SSID: YOUR_WIFI
IP Address: 192.168.0.2
signal strength (RSSI):-41 dBm
Autoscroll
Clear output
9600 baud
Newline
- You will see an IP address, for example: 192.168.0.2. This is the IP address of the Arduino Web Server
- Open a web browser and enter one of the IP address into the address bar.
- Kindly be aware that the IP address might vary. Please verify the current value on the Serial Monitor.
- You will also see the below output on Serial Monitor
COM6
Attempting to connect to SSID: YOUR_WIFI
IP Address: 192.168.0.2
signal strength (RSSI):-41 dBm
New HTTP Request
<< GET / HTTP/1.1
<< Host: 192.168.0.2
<< Connection: keep-alive
<< Cache-Control: max-age=0
<< Upgrade-Insecure-Requests: 1
<< User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/119.0.0.0 Safari/537.36
<< Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7
<< Accept-Encoding: gzip, deflate
<< Accept-Language: en-US,en;q=0.9
Autoscroll
Clear output
9600 baud
Newline
- You will see the web page of Arduino board on the web browser as below
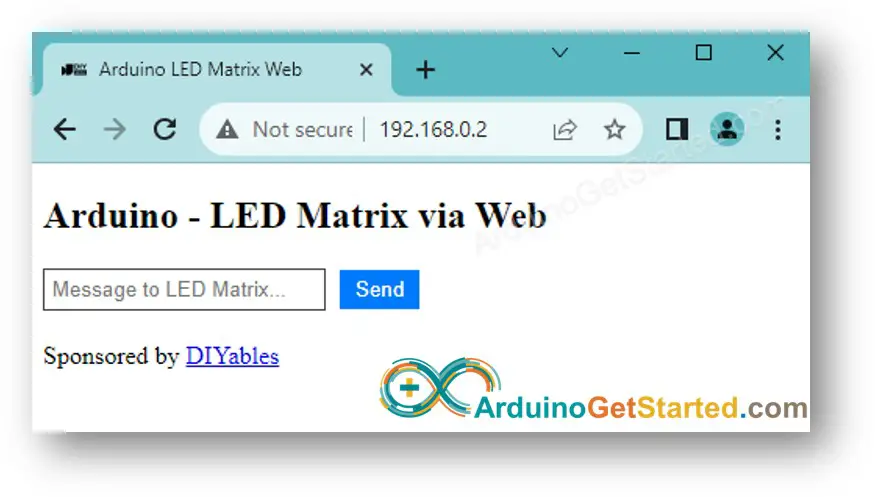
- Type a message and click the send button to send the message to Arduino.
- Check out the LED Matrix display.
※ NOTE THAT:
- If alterations are made to the HTML content within the index.h file without any modifications to the ArduinoGetStarted.com.ino file, the Arduino IDE will not automatically refresh or update the HTML content during the compilation and upload of the code to the ESP32.
- To compel the Arduino IDE to update the HTML content in such a scenario, it is necessary to make a modification in the ArduinoGetStarted.com.ino file. For instance, you can add an empty line or insert a comment. This action prompts the IDE to acknowledge the changes in the project, ensuring that the revised HTML content is included in the upload.