Arduino - Sound Sensor - LED
In this tutorial, we'll explore how to utilize the sound sensor for LED control. Specifically, we'll delve into two exciting applications:
- Sound switch: When sound is detected (e.g knock), the Arduino toggles the LED, turning it on if it's off, and off if it's on.
- Sound-activated LED: Upon detecting sound, the Arduino turns on the LED for a specific period of time.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
About LED and Sound Sensor
If you do not know about led and sound sensor (pinout, how it works, how to program ...), learn about them in the following tutorials:
Wiring Diagram
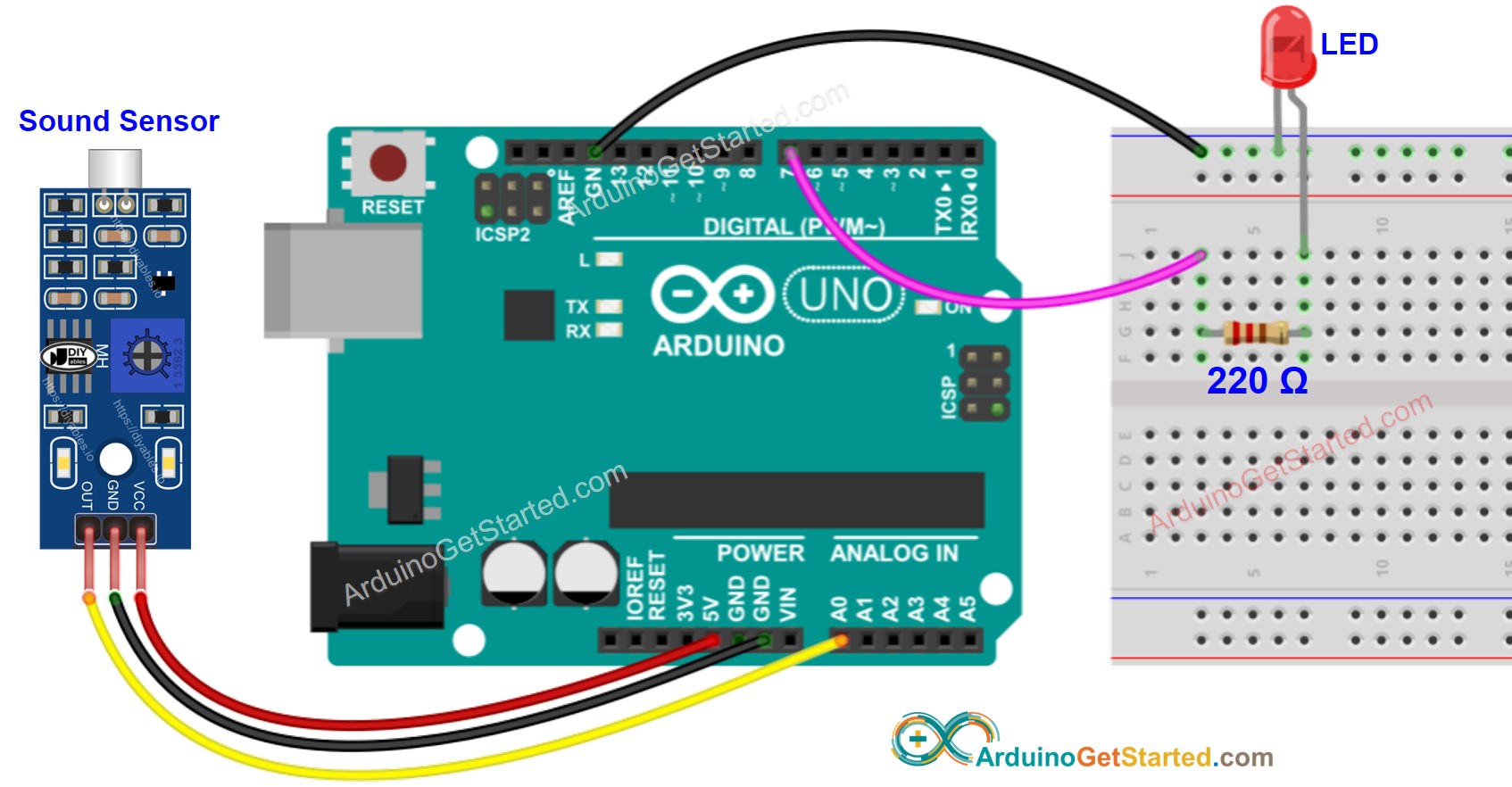
This image is created using Fritzing. Click to enlarge image
Arduino Code - Sound Switch toggles LED
The below code toggles the state of LED each time the sound is detected.
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
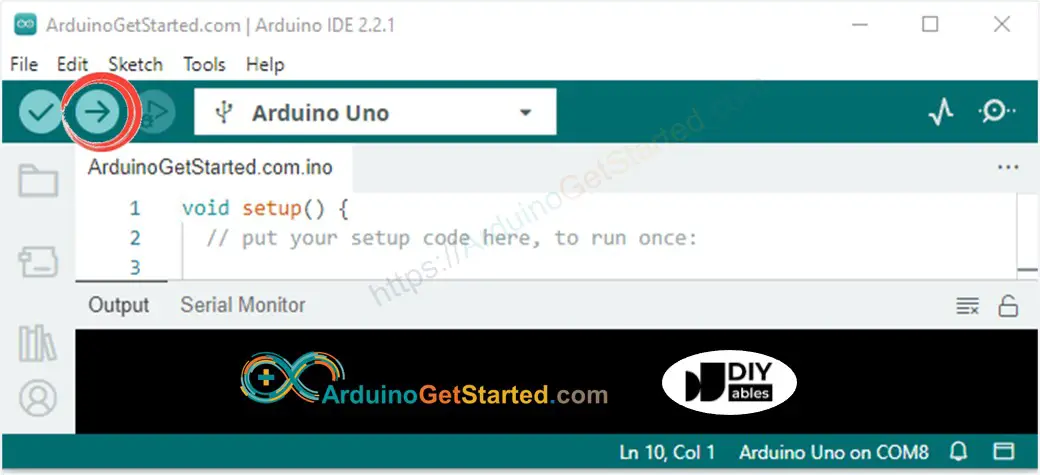
- Clap your hand in front of the sound sensor
- See the change of LED's state
Code Explanation
Read the line-by-line explanation in comment lines of source code!
Arduino Code - Sound-activated LED for a period of time
The below code turns on LED for a period of time when the sound is detected. After the period of time, LED is turned off.
Please take note that the previous code utilizes the delay() function, which is straightforward to understand. However, when additional code is added, the delay() function can cause blocking issues during the delay period. To overcome this, the following code implements a non-blocking approach by utilizing the ezLED library. The ezLED library, working behind the scenes, utilizes the millis() function instead of delay to prevent blocking.
Quick Steps
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezLED”, then find the led library by ArduinoGetStarted
- Click Install button to install ezLED library.
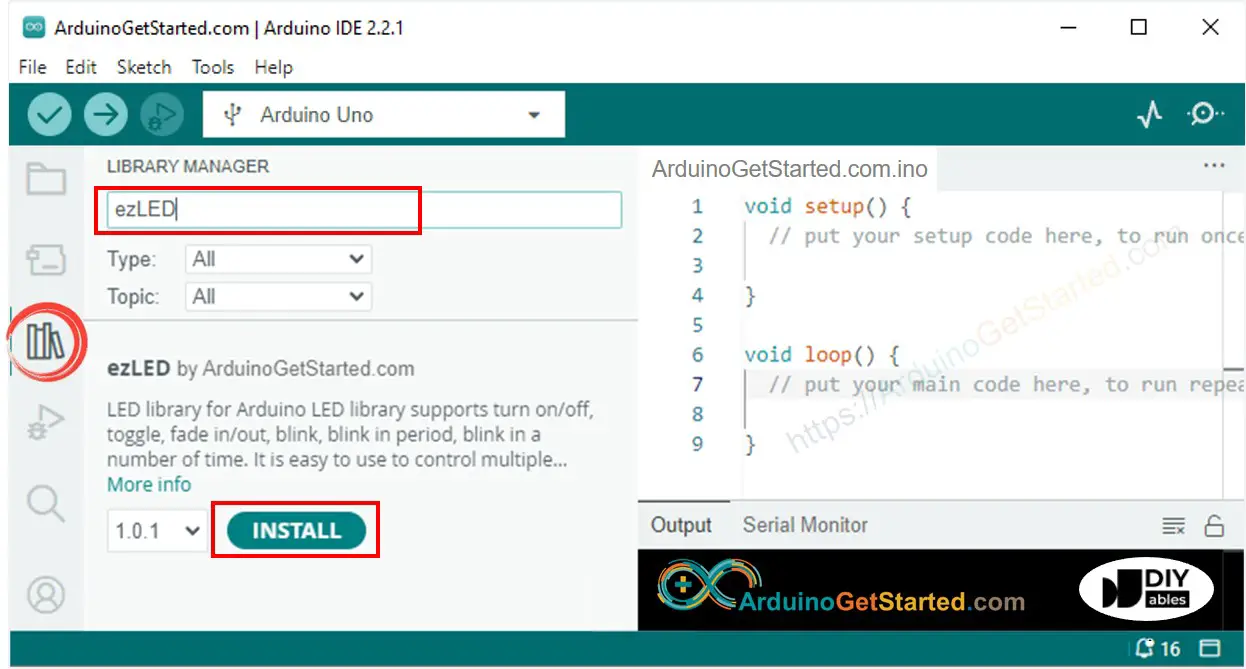
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
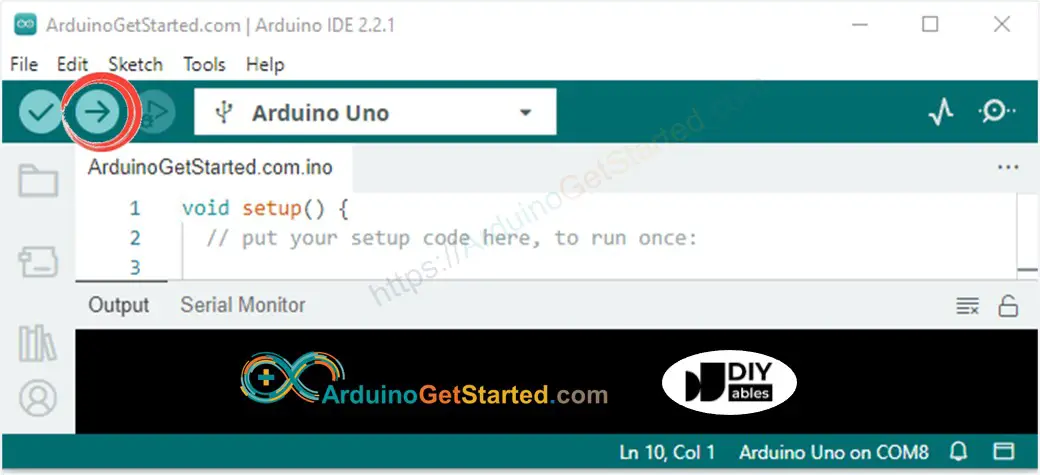
- Clap your hand in front of the sound sensor
- See the change of LED's state
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.