Arduino - LCD I2C
In this Arduino LCD I2C tutorial, we will learn how to connect an LCD I2C (Liquid Crystal Display) to the Arduino board. LCDs are very popular and widely used in electronics projects for displaying information. There are many types of LCD. This tutorial takes LCD 16x2 (16 columns and 2 rows) as an example. The other LCDs are similar.
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables.
About LCD I2C 16x2
In the previous tutorial, we had learned how to use the normal LCD. However, wiring between Arduino and the normal LCD is complicated. Therefore, LCD I2C has been created to simplify the wiring. Actually, LCD I2C is composed of a normal LCD, an I2C module and a potentiometer.
Pinout
LCD I2C uses I2C interface, so it has 4 pins:
- GND pin: needs to be connected to GND (0V).
- VCC pin: the power supply for the LCD, needs to be connected to VCC (5V).
- SDA pin: I2C data signal
- SCL pin: I2C clock signal
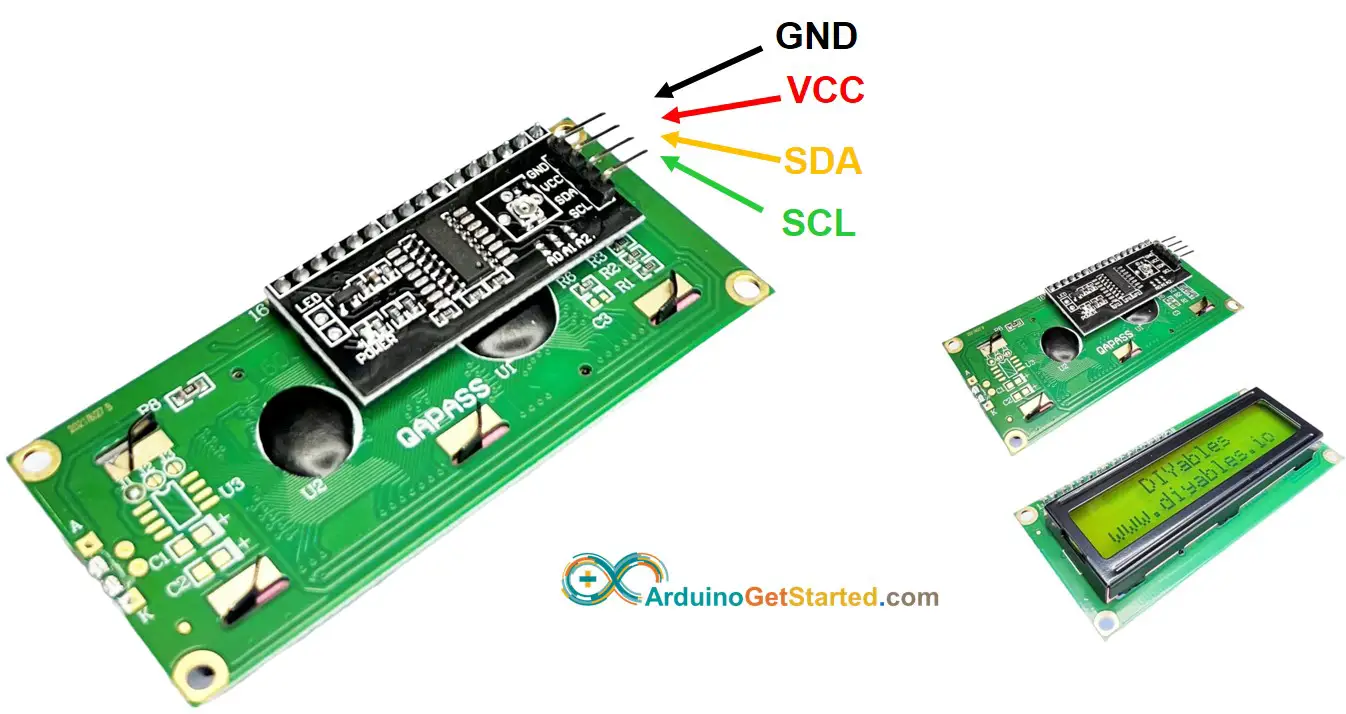
LCD Coordinate
LCD I2C 16x2 includes 16 columns and 2 rows. the conlums and rows are indexed from 0.
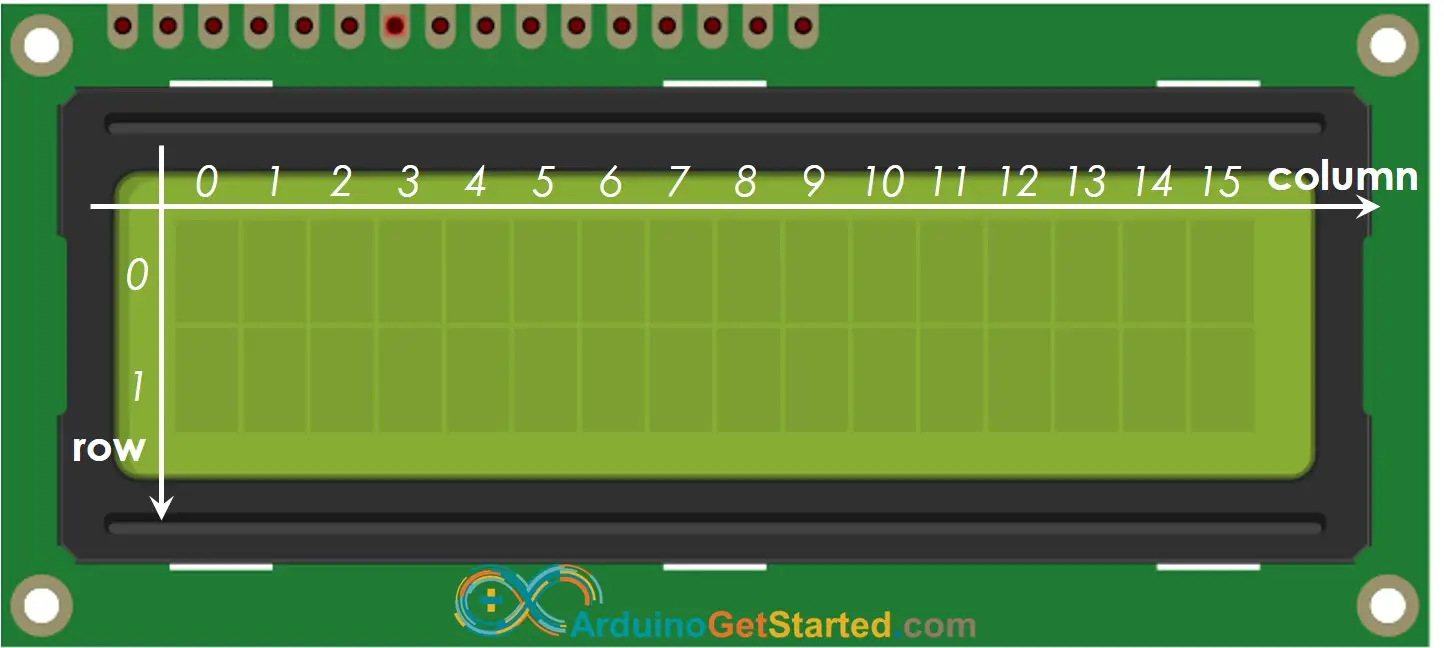
Wiring Diagram
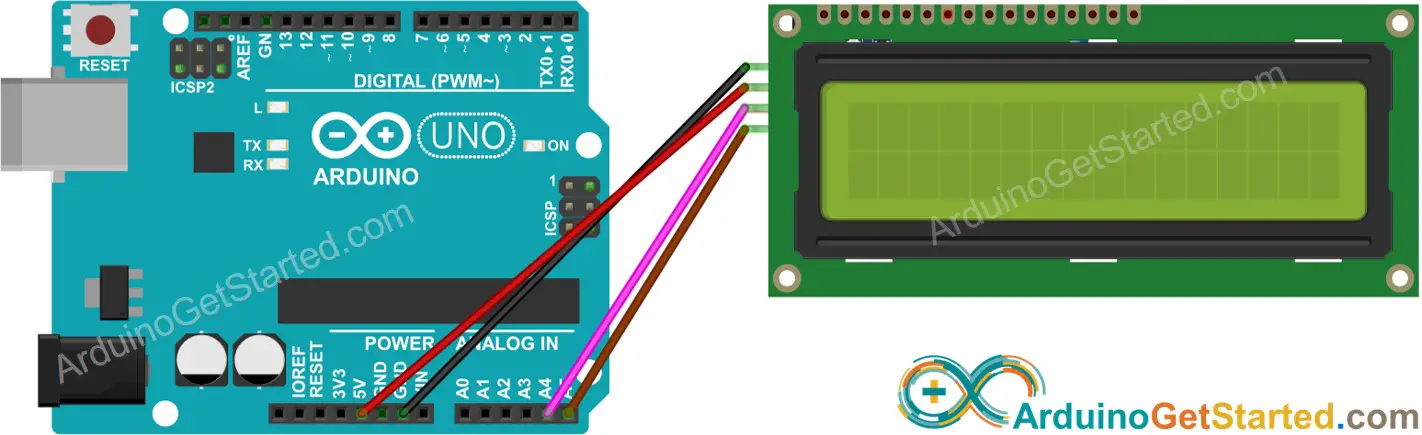
This image is created using Fritzing. Click to enlarge image
LCD I2C | Arduino Uno, Nano | Arduino Mega |
---|---|---|
Vin | 5V | 5V |
GND | GND | GND |
SDA | A4 | 20 |
SCL | A5 | 21 |
How To Program For LCD I2C
Thanks to the LiquidCrystal_I2C library, the using LCD is a piece of cake.
- Include the library:
- Declare a LiquidCrystal_I2C object with I2C address, the number of columns, the number of rows:
- Initialize the LCD.
- Move cursor to the desired position (column_index, row_index)
- Print a message to the LCD.
There are many things more that we can do with LCD (see Do More with LCD part)
※ NOTE THAT:
The I2C address of LCD can vary according to the manufacturers. In the code, we used 0x27 that is specified by DIYables brand
Arduino Code
Quick Steps
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search "LiquidCrystal I2C", then find the LiquidCrystal_I2C library by Frank de Brabander
- Click Install button to install LiquidCrystal_I2C library.
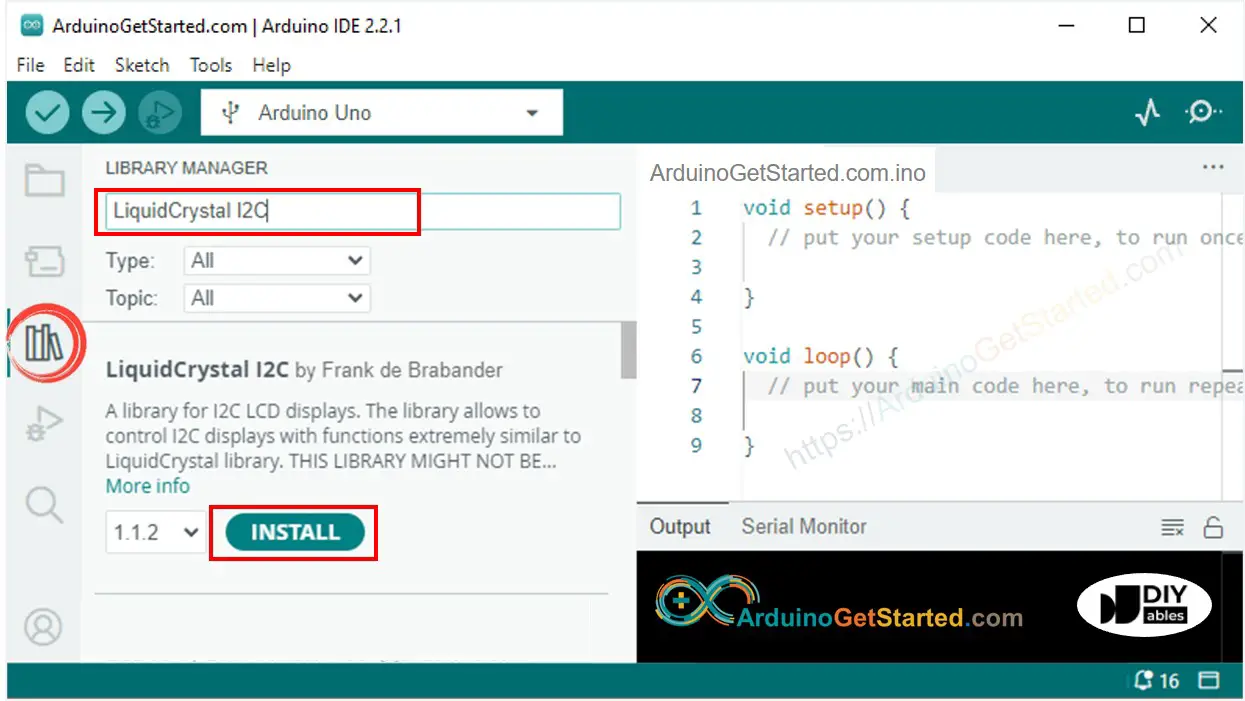
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See the result on LCD
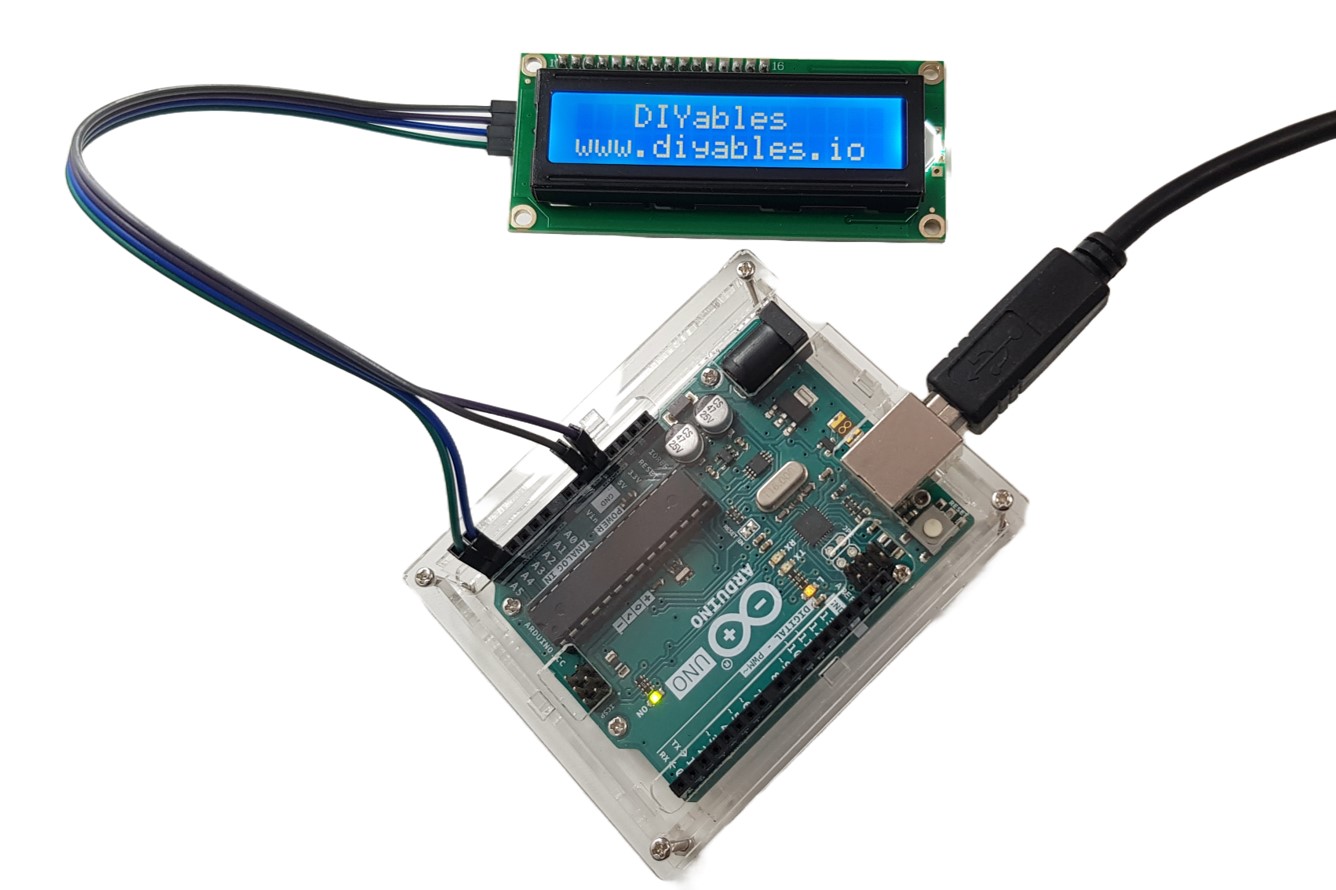
- Try modifying text and position
LCD I2C Selection Guide
There are various types of LCD I2C displays available in the market, mainly differing in background color and the shape of the I2C interface module.
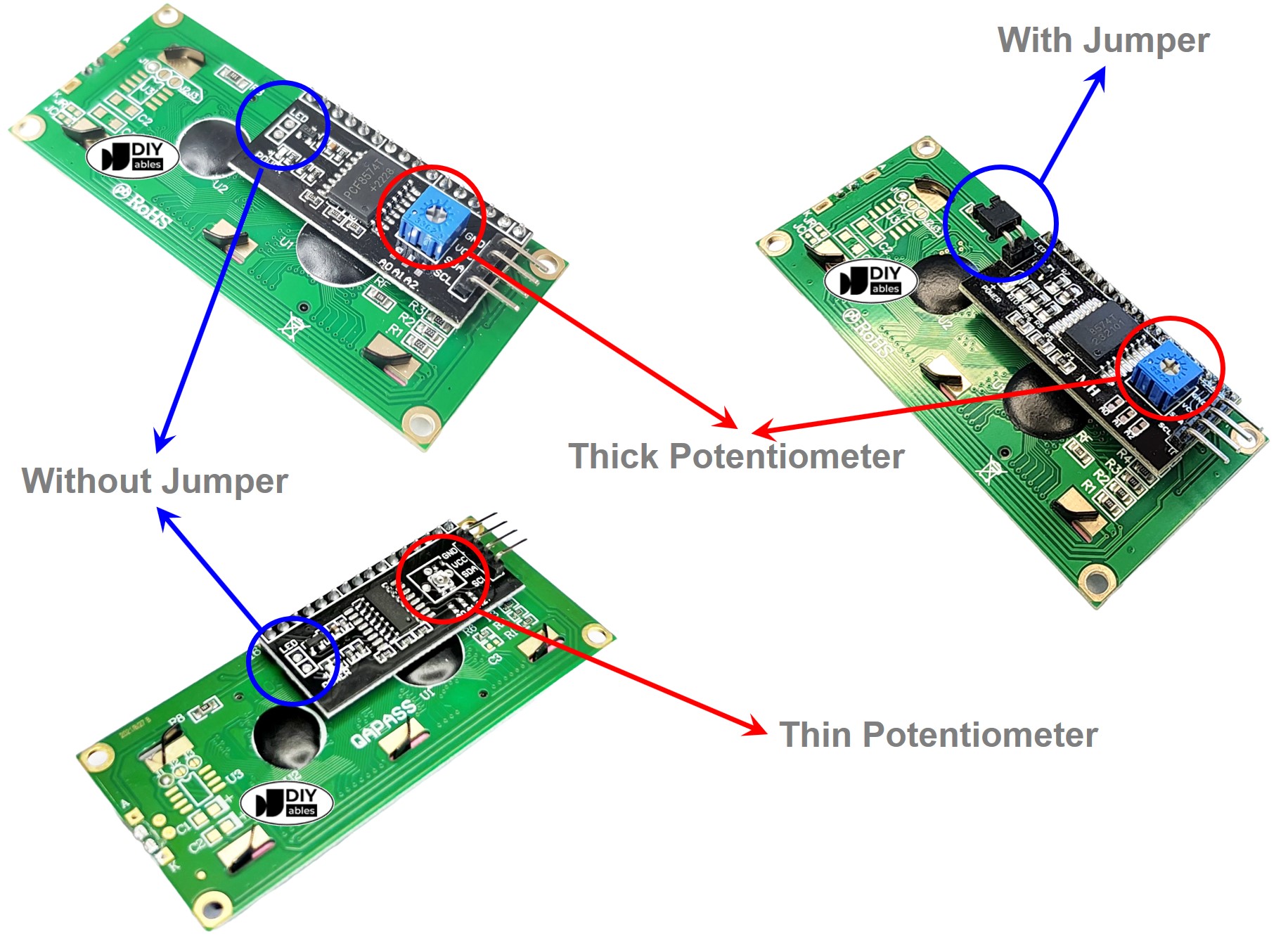
Regarding color, these displays usually come in two background colors: green and blue.
For the I2C interface module shape, there are two main aspects to consider:
- Potentiometer size: This includes options for thin and thick potentiometers.
- Thick potentiometer: Easier to adjust but occupies more space.
- Thin potentiometer: More compact, saving space with its smaller height.
- I2C selection jumper:
- With jumper: Allows switching between two I2C addresses, useful when your code uses two LCD displays simultaneously. However, it can cause confusion with I2C addresses when only one LCD is used and it also requires more space.
- Without jumper: This has a fixed I2C address and is very convenient when your code uses just one LCD display. Since most applications use only one LCD, this type is highly recommended.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
Do More with LCD
Custom Character
lcd.print() function supports only ASCII characters. If you want to display a special character or symbol (e.g. heart, angry bird), you need to use the below character generator.
LCD 16x2 can display 32 characters (2 rows and 16 columns). Each character is composed of 40 pixels (8 rows and 5 columns).
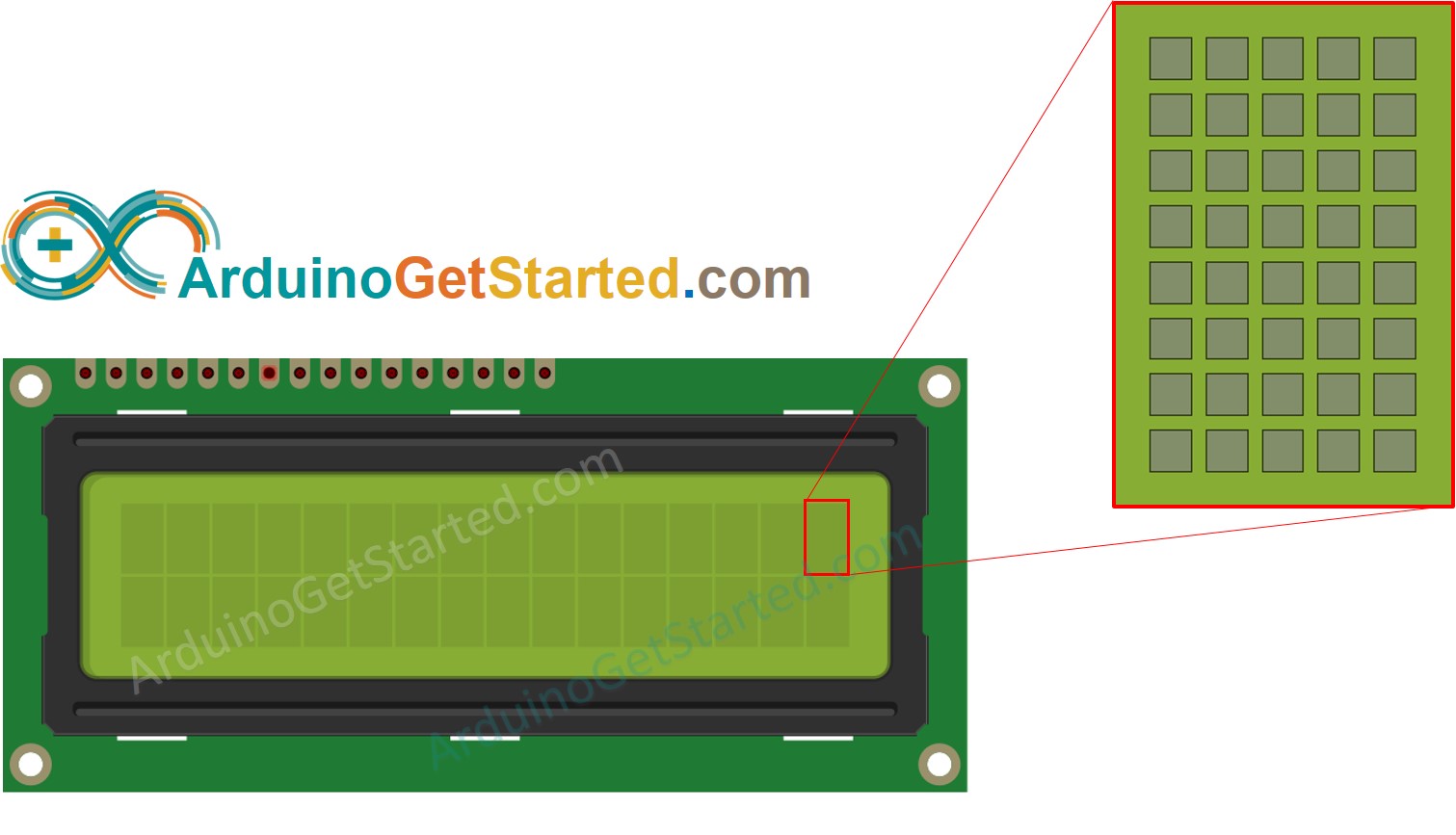
The character generator represents a character (40 pixels). You just need to do the following steps:
Result on LCD:
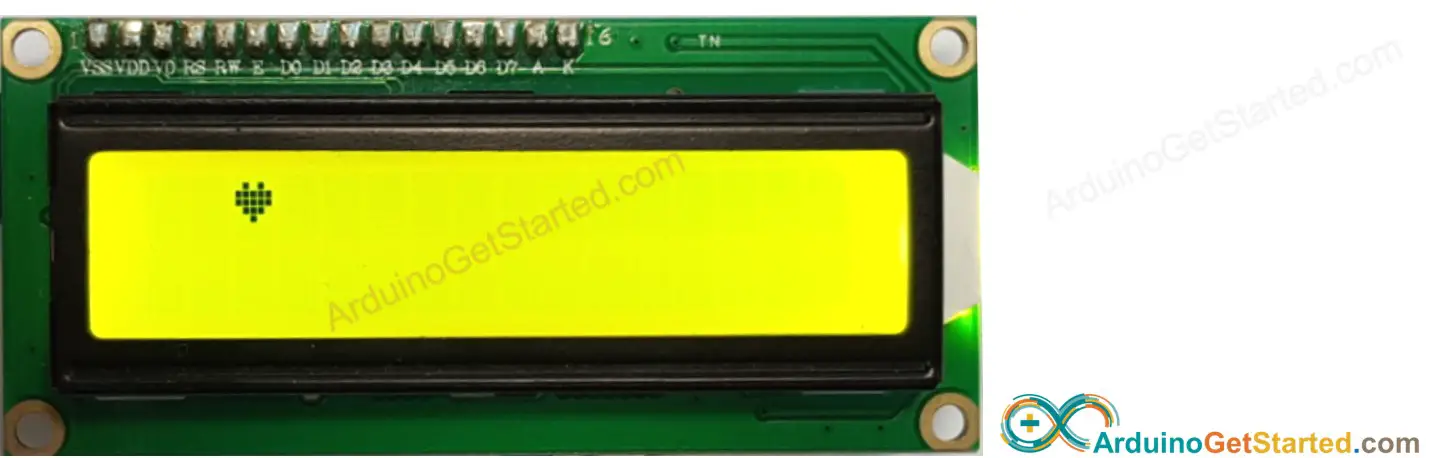
Multiple custom characters
We can create up to 8 custom characters (indexed 0 to 7). The below example creates and displays three characters.
Result on LCD:
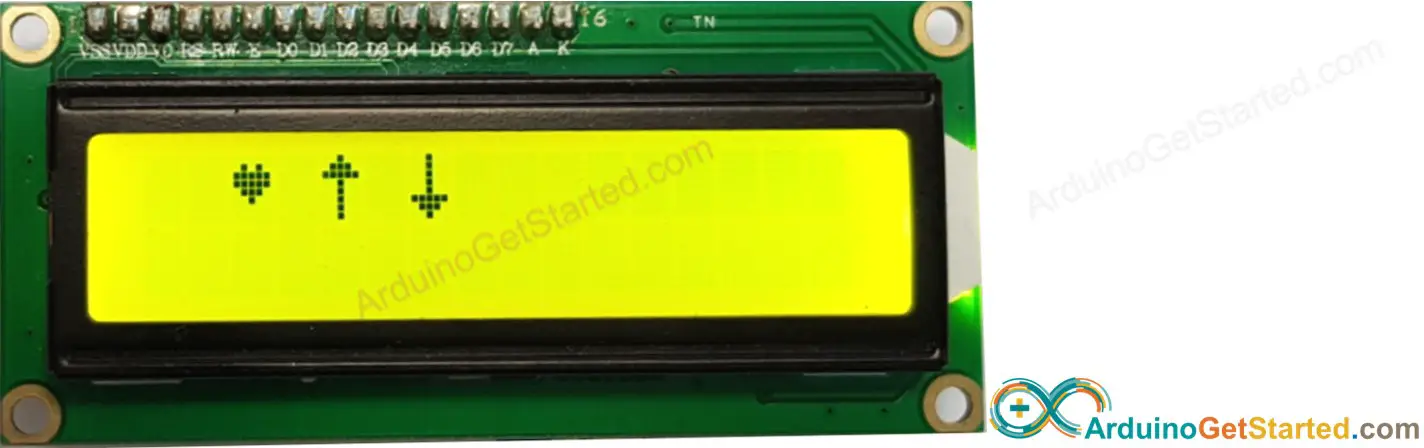
Summary: how to use custom character on LCD
- Use the above character generator to create binary code for the custom character.
- Declare the binary code for the custom character (copy from above step)
- Create custom character and assign to an index value (from 0 to 7) in setup() function
- Print the custom character in LCD anytime, anywhere (in setup() or loop() function)
Other functions
Add the below functions into loop() function one by one. And add delay(5000) after each function
- Clear LCD screen
- Move the cursor to the upper-left of the LCD
- Move the cursor to the a position (column, row)
- Display the LCD cursor
- Hides the LCD cursor.
- Display the blinking LCD cursor
- Turns off the blinking LCD cursor.
- And more at LiquidCrystal Library Reference
Challenge Yourself
Use LCD to do one of the following projects:
- Sending text from PC (via Serial Monitor) and display on LCD. Hint: Refer to How to send data from PC to Aduino
- Displaying the pressed key of the keypad on LCD. Hint: Refer to Arduino - Keypad
Troubleshooting on LCD I2C
If the text is not displayed on LCD I2C, please check the following issues:
- Adjust the brightness of LCD by rotating potentiometer in the backside of LCD
- Depending on manufacturers, the I2C address of LCD may be different. Usually, the default I2C address of LCD is 0x27 or 0x3F. Try these values one by one. If you still failed, run the below code to find the I2C address.
The result on Serial Monitor: