Arduino - Infrared Obstacle Avoidance Sensor
In this tutorial, we are going to learn how to use Arduino and the infrared obstacle avoidance sensor to detect the presence of the obstacle.
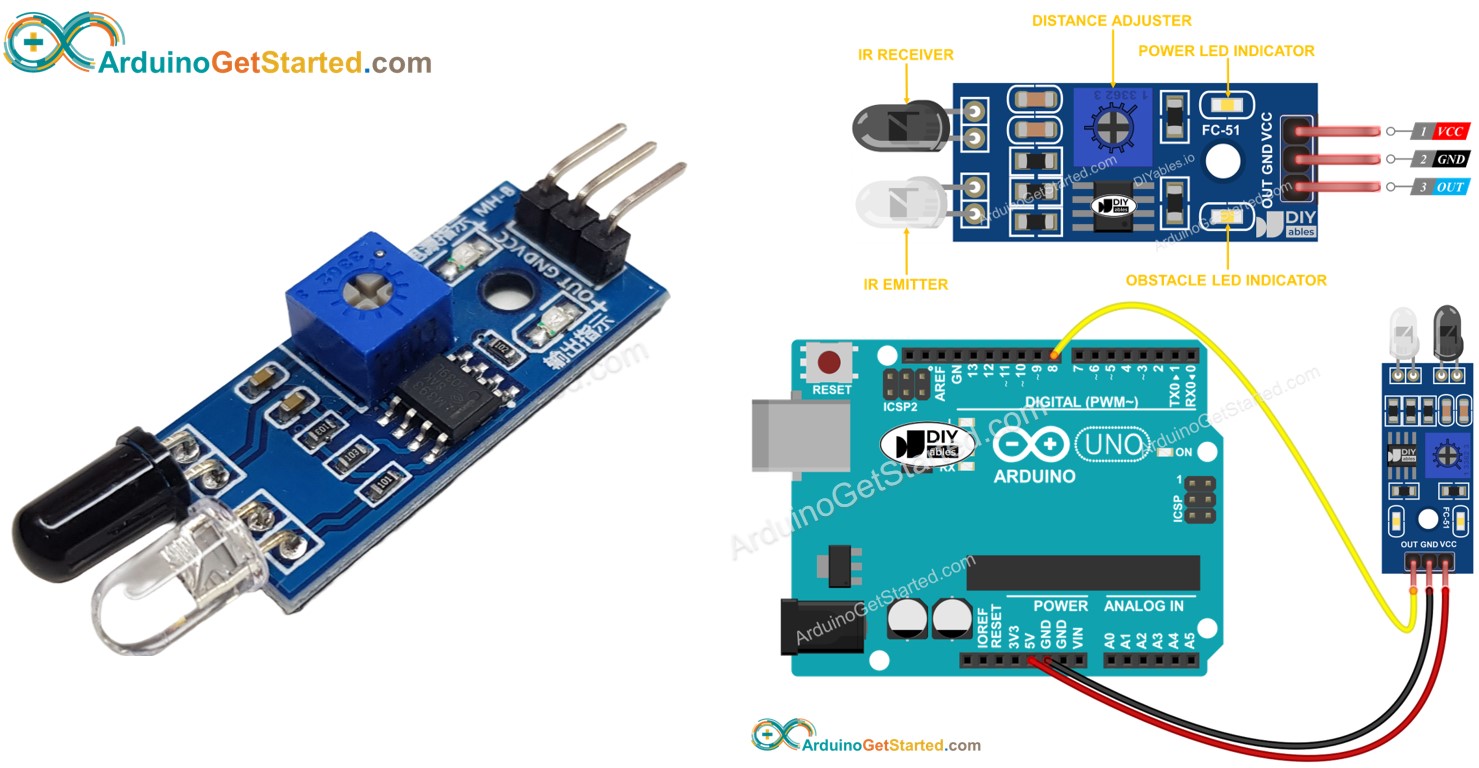
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About IR Obstacle Avoidance Sensor
The infrared (IR) obstacle sensor is used to detect the presence of any obstacle in front of the sensor module by using the infrared signal. The detection range is from 2cm to 30cm. The detection range can be adjusted by a built-in potentiometer.
Pinout
IR obstacle avoidance sensor includes three pins:
- GND pin: needs to be connected to GND (0V)
- VCC pin: needs to be connected to VCC (5V or 3.3v)
- OUT pin: is an output pin: LOW when there is an obstacle, HIGH when there is no obstacle. This pin needs to be connected to Arduino's input pin.
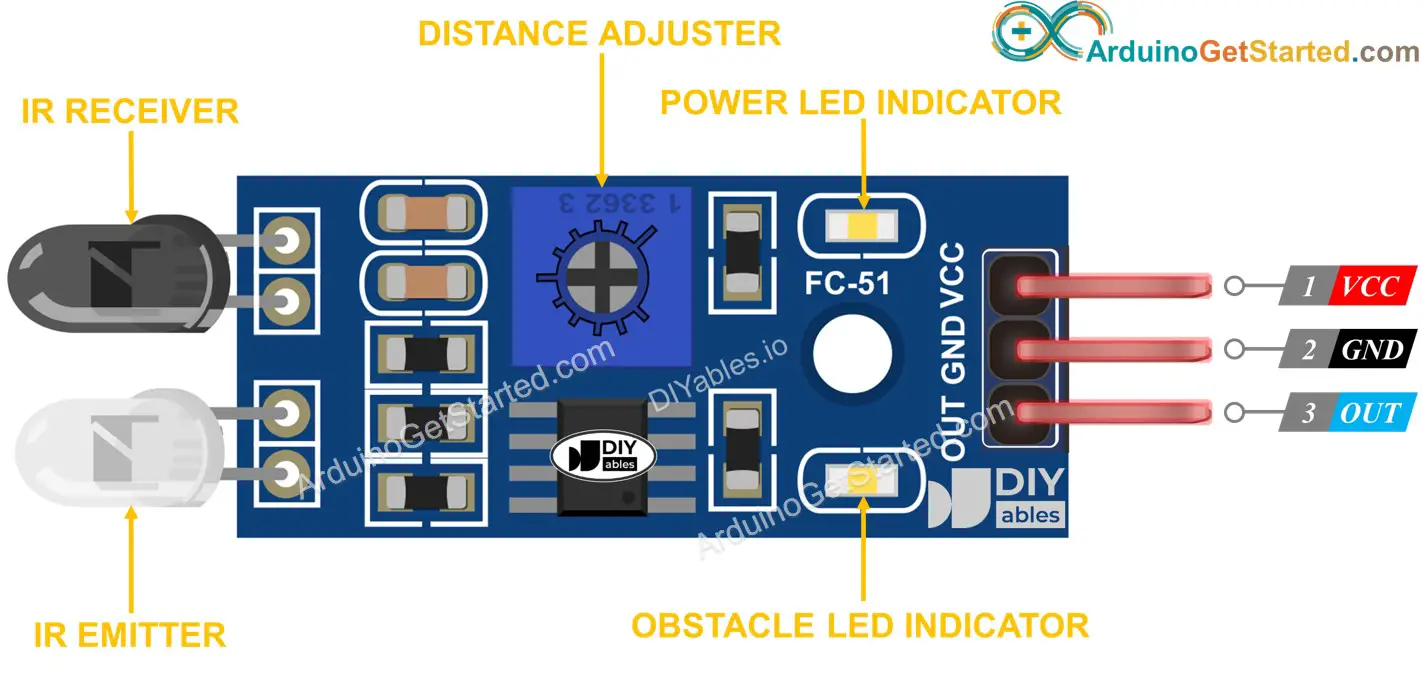
How It Works
An infrared obstacle sensor module has a built-in IR transmitter and IR receiver. The IR transmitter emits the IR signal. The IR receiver looks for the reflected IR signal to determine if the object is present or not. The presence of obstacle is reflected in the OUT pin:
- If the obstacle is present in front of the sensor, the sensor's OUT pin is LOW
- If the obstacle is NOT present in front of the sensor, the sensor's OUT pin is HIGH
※ NOTE THAT:
During shipment, the sensor may get deformed, which can cause malfunctioning. If the sensor is not working properly, adjust the IR transmitter and receiver to make them parallel.
Wiring Diagram
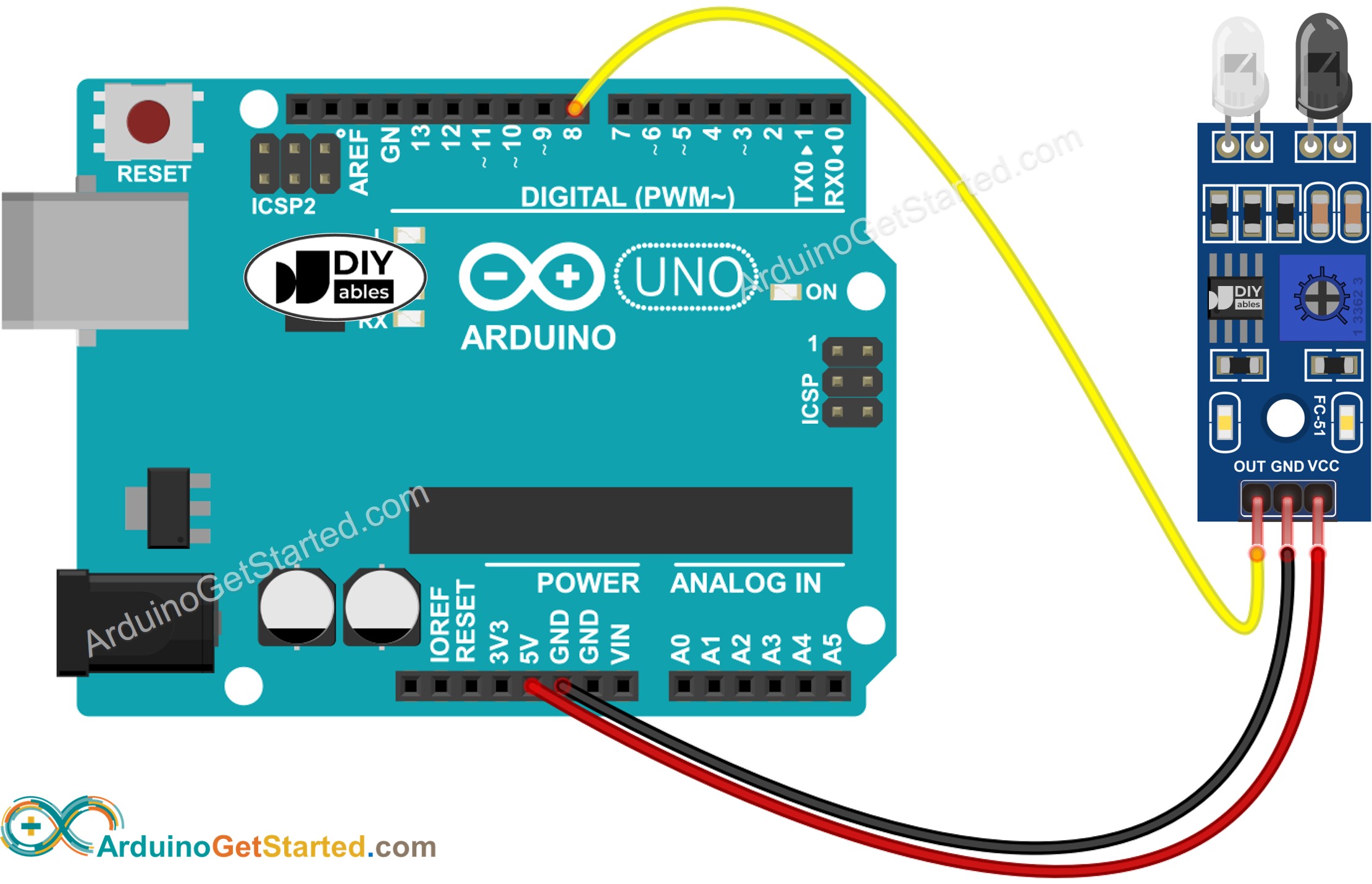
This image is created using Fritzing. Click to enlarge image
How To Program For IR Obstacle Avoidance Sensor
- Initializes the Arduino pin to the digital input mode by using pinMode() function. For example, pin GIOP18
- Reads the state of the Arduino pin by using digitalRead() function.
Arduino Code
There are two ways to program for an obstacle avoidance application:
- Do or don't do something while the obstacle is present or not present
- Do or don't do something when the obstacle is detected or cleared
Arduino code for checking if the obstacle is present
Quick Steps
- Copy the above code and open it with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Put an obstacle in front of the sensor for a while, and then remove it.
- See the result on Serial Monitor.
Arduino code for detecting obstacle
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Put an obstacle in front of the sensor awhile, and then remove it.
- See the result on Serial Monitor.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.