Arduino multiple Button
This tutorial teaches you how to program an Arduino to make use multiple buttons simultaneously without using the delay() function. The tutorial provides code in two ways:
We will use five buttons as examples. You can easily modify it to adapt for two buttons, four buttons, or even more.
Or you can buy the following kits:
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand,
DIYables .
We have a detailed button tutorial including hardware pinout, working principle, Arduino wiring, and code instructions. Learn more here:
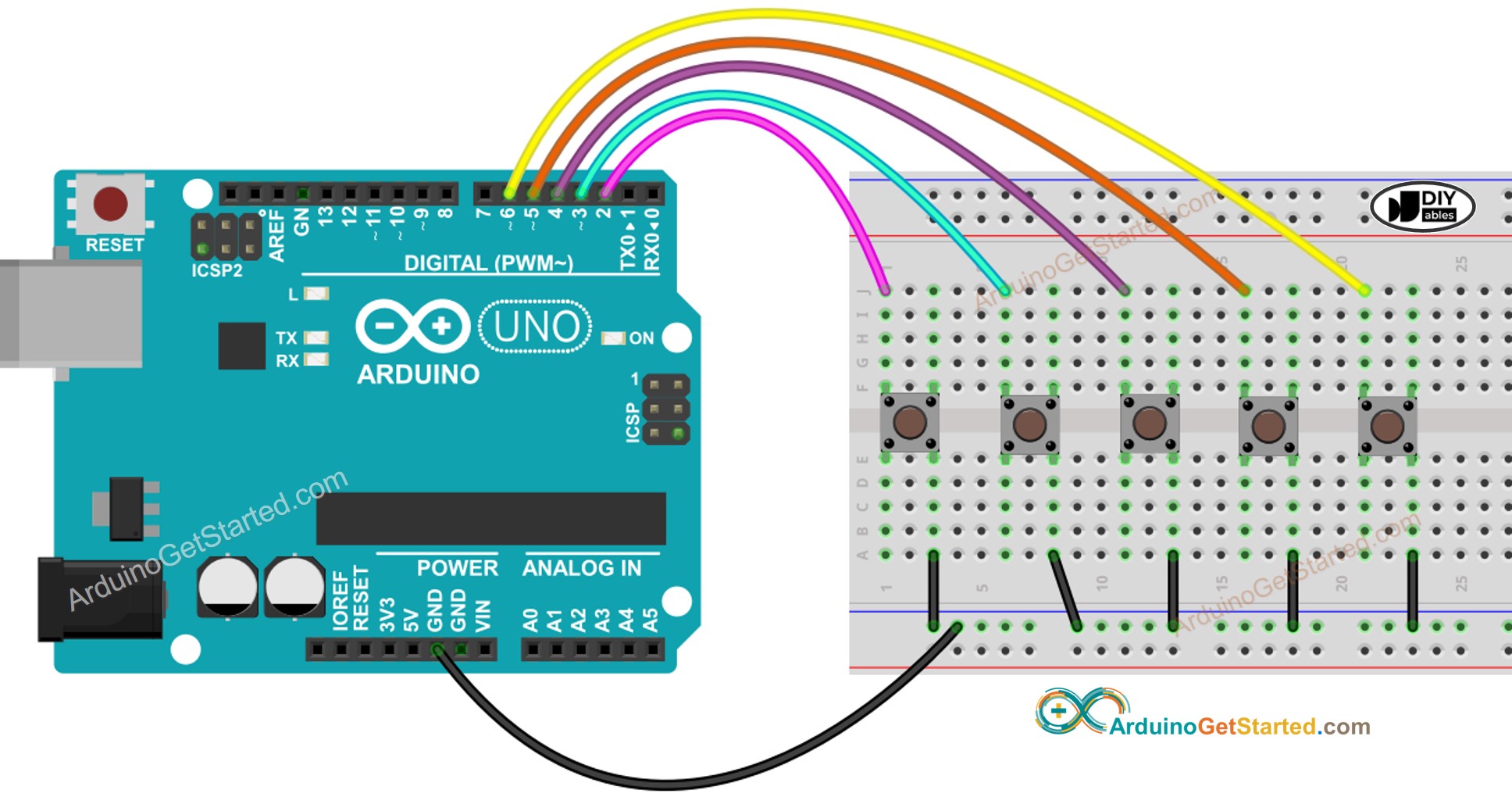
This image is created using Fritzing. Click to enlarge image
When using multiple buttons, things can get complicated in certain scenarios:
Thankfully, the ezButton library streamlines this process by internally managing debounce and button events. This relieves users from the task of managing timestamps and variables when utilizing the library. Additionally, employing an array of buttons can enhance code clarity and brevity.
#include <ezButton.h>
#define BUTTON_NUM 5
#define BUTTON_PIN_1 2
#define BUTTON_PIN_2 3
#define BUTTON_PIN_3 4
#define BUTTON_PIN_4 5
#define BUTTON_PIN_5 6
ezButton button1(BUTTON_PIN_1);
ezButton button2(BUTTON_PIN_2);
ezButton button3(BUTTON_PIN_3);
ezButton button4(BUTTON_PIN_4);
ezButton button5(BUTTON_PIN_5);
void setup() {
Serial.begin(9600);
button1.setDebounceTime(100);
button2.setDebounceTime(100);
button3.setDebounceTime(100);
button4.setDebounceTime(100);
button5.setDebounceTime(100);
}
void loop() {
button1.loop();
button2.loop();
button3.loop();
button4.loop();
button5.loop();
int button1_state = button1.getState();
int button2_state = button2.getState();
int button3_state = button3.getState();
int button4_state = button4.getState();
int button5_state = button5.getState();
if (button1.isPressed())
Serial.println("The button 1 is pressed");
if (button1.isReleased())
Serial.println("The button 1 is released");
if (button2.isPressed())
Serial.println("The button 2 is pressed");
if (button2.isReleased())
Serial.println("The button 2 is released");
if (button3.isPressed())
Serial.println("The button 3 is pressed");
if (button3.isReleased())
Serial.println("The button 3 is released");
if (button4.isPressed())
Serial.println("The button 4 is pressed");
if (button4.isReleased())
Serial.println("The button 4 is released");
if (button5.isPressed())
Serial.println("The button 5 is pressed");
if (button5.isReleased())
Serial.println("The button 5 is released");
}
Do the wiring as above image.
Connect the Arduino board to your PC via a USB cable
Open Arduino IDE on your PC.
Select the right Arduino board (e.g. Arduino Uno) and COM port.
Click to the Libraries icon on the left bar of the Arduino IDE.
Search “ezButton”, then find the button library by ArduinoGetStarted
Click Install button to install ezButton library.
The button 1 is pressed
The button 1 is released
The button 2 is pressed
The button 2 is released
The button 3 is pressed
The button 3 is released
The button 4 is pressed
The button 4 is released
The button 5 is pressed
The button 5 is released
We can improve the code above by employing an array of buttons. The following code utilizes this array to handle button objects.
#include <ezButton.h>
#define BUTTON_NUM 5
#define BUTTON_PIN_1 2
#define BUTTON_PIN_2 3
#define BUTTON_PIN_3 4
#define BUTTON_PIN_4 5
#define BUTTON_PIN_5 6
ezButton buttonArray[] = {
ezButton(BUTTON_PIN_1),
ezButton(BUTTON_PIN_2),
ezButton(BUTTON_PIN_3),
ezButton(BUTTON_PIN_4),
ezButton(BUTTON_PIN_5)
};
void setup() {
Serial.begin(9600);
for (byte i = 0; i < BUTTON_NUM; i++) {
buttonArray[i].setDebounceTime(100);
}
}
void loop() {
for (byte i = 0; i < BUTTON_NUM; i++)
buttonArray[i].loop();
for (byte i = 0; i < BUTTON_NUM; i++) {
int button_state = buttonArray[i].getState();
if (buttonArray[i].isPressed()) {
Serial.print("The button ");
Serial.print(i + 1);
Serial.println(" is pressed");
}
if (buttonArray[i].isReleased()) {
Serial.print("The button ");
Serial.print(i + 1);
Serial.println(" is released");
}
}
}
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
※ OUR MESSAGES
You can share the link of this tutorial anywhere. Howerver, please do not copy the content to share on other websites. We took a lot of time and effort to create the content of this tutorial, please respect our work!