Arduino - Ethernet Module
This tutorial shows you how to use the Arduino and Ethenet module to connect to the Internet or your LAN network. Here's what we'll cover:
Connecting the Arduino to a W5500 Ethernet Module
Programming the Arduino to send HTTP requests over Ethernet
Programming the Arduino to create a web server through Ethernet
Or you can buy the following sensor kits:
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand,
DIYables .
The W5500 Ethernet module has two ways to connect:
RJ45 Ethernet Interface: Use an Ethernet cable to connect to a router or switch.
SPI Interface: Use this to connect to an Arduino board. It has 10 pins. These pin need to be connected to Arduino as below table:
Ethenet Module Pins | Arduino Pins |
NC pin | NOT connected |
INT pin | NOT connected |
RST pin | Reset pin |
GND pin | GND pin |
5V pin | 5V pin |
3.3V pin | NOT connected |
MISO pin | 12 (MISO) |
MOSI pin | 11 (MOSI) |
SCS pin | 10 (SS) |
SCLK pin | 13 (SCK) |
image source: diyables.io
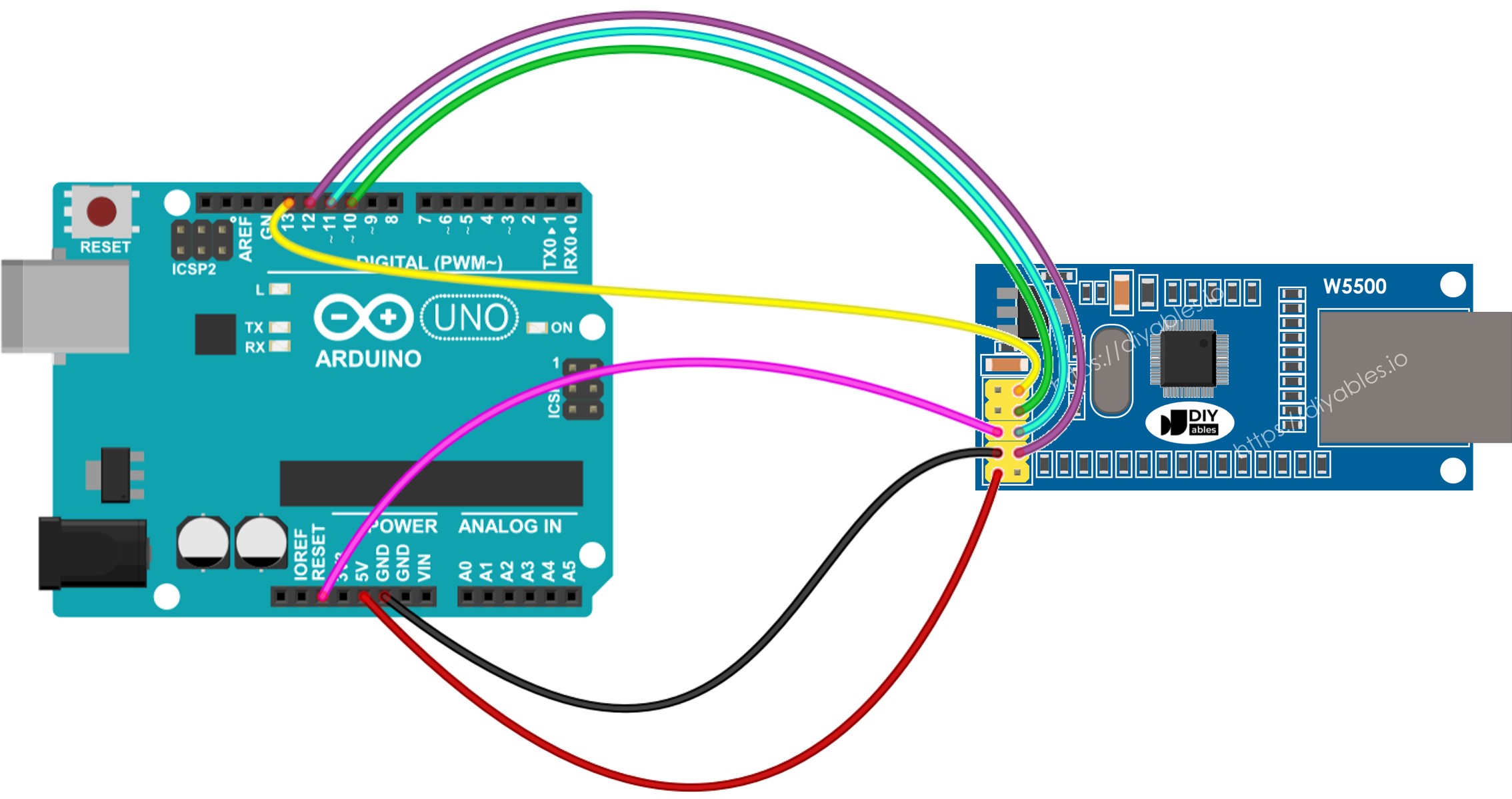
This image is created using Fritzing. Click to enlarge image
The below is real connection between Arduino and W5500 Ethernet module
This code acts as a web client. It sends HTTP requests to the web server located at http://example.com/.
#include <SPI.h>
#include <Ethernet.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
EthernetClient client;
int HTTP_PORT = 80;
String HTTP_METHOD = "GET";
char HOST_NAME[] = "example.com";
String PATH_NAME = "/";
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println("Arduino - Ethernet Tutorial");
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to obtaining an IP address");
if (Ethernet.hardwareStatus() == EthernetNoHardware)
Serial.println("Ethernet shield was not found");
if (Ethernet.linkStatus() == LinkOFF)
Serial.println("Ethernet cable is not connected.");
while (true)
;
}
if (client.connect(HOST_NAME, HTTP_PORT)) {
Serial.println("Connected to server");
client.println(HTTP_METHOD + " " + PATH_NAME + " HTTP/1.1");
client.println("Host: " + String(HOST_NAME));
client.println("Connection: close");
client.println();
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.print(c);
}
}
client.stop();
Serial.println();
Serial.println("disconnected");
} else {
Serial.println("connection failed");
}
}
void loop() {
}
Please follow these steps one by one:
Connect the Ethernet module to your Arduino as shown in the diagram provided.
Use an Ethernet cable to connect the Ethernet module to your router or switch.
Use a USB cable to connect the Arduino board to your computer.
Open the Arduino IDE on your computer.
Choose the correct Arduino board and COM port.
Click on the Libraries icon on the left side of the Arduino IDE.
In the search box, type “Ethernet” and find the Ethernet library by Various.
Click the Install button to add the Ethernet library.
Open the Serial Monitor in the Arduino IDE.
Copy the provided code and paste it into the Arduino IDE.
Click the Upload button in the Arduino IDE to transfer the code to the Arduino.
Check out the Serial Monitor where the results will appear as below.
Arduino - Ethernet Tutorial
Connected to server
HTTP/1.1 200 OK
Accept-Ranges: bytes
Age: 208425
Cache-Control: max-age=604800
Content-Type: text/html; charset=UTF-8
Date: Fri, 12 Jul 2024 07:08:42 GMT
Etag: "3147526947"
Expires: Fri, 19 Jul 2024 07:08:42 GMT
Last-Modified: Thu, 17 Oct 2019 07:18:26 GMT
Server: ECAcc (lac/55B8)
Vary: Accept-Encoding
X-Cache: HIT
Content-Length: 1256
Connection: close
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8" />
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
</head>
<body>
<div>
<h1>Example Domain</h1>
<p>This domain is for use in illustrative examples in documents. You may use this
domain in literature without prior coordination or asking for permission.</p>
<p><a href="https://www.iana.org/domains/example">More information...</a></p>
</div>
</body>
</html>
disconnected
※ NOTE THAT:
If another device on the same network shares your MAC address, there may be issues.
The code below create a web server on the Arduino. This web server sends a simple webpage to the web browsers.
#include <SPI.h>
#include <Ethernet.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
EthernetServer server(80);
void setup() {
Serial.begin(9600);
delay(1000);
Serial.println("Arduino - Ethernet Tutorial");
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to obtaining an IP address");
if (Ethernet.hardwareStatus() == EthernetNoHardware)
Serial.println("Ethernet shield was not found");
if (Ethernet.linkStatus() == LinkOFF)
Serial.println("Ethernet cable is not connected.");
while (true)
;
}
server.begin();
Serial.print("Arduino - Web Server IP Address: ");
Serial.println(Ethernet.localIP());
}
void loop() {
EthernetClient client = server.available();
if (client) {
Serial.println("new client");
bool currentLineIsBlank = true;
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.write(c);
if (c == '\n' && currentLineIsBlank) {
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<body>");
client.println("<h1>Arduino - Web Server with Ethernet</h1>");
client.println("</body>");
client.println("</html>");
break;
}
if (c == '\n') {
currentLineIsBlank = true;
} else if (c != '\r') {
currentLineIsBlank = false;
}
}
}
delay(1);
client.stop();
Serial.println("client disconnected");
}
}
Copy the above code and paste it into Arduino IDE.
Click the Upload button in Arduino IDE to upload the code to Arduino.
Check the result on Serial Monitor, it will display as follows:
Arduino - Ethernet Tutorial
Arduino - Web Server IP Address: 192.168.0.2
※ OUR MESSAGES
You can share the link of this tutorial anywhere. Howerver, please do not copy the content to share on other websites. We took a lot of time and effort to create the content of this tutorial, please respect our work!