Arduino - WebSocket
In this guide, we'll explore what WebSocket is, why it's useful for controlling Arduino effectively, and how to implement WebSocket with Arduino. Through a hands-on example, we'll demonstrate how to build a chat application that connects a web browser with Arduino, enabling you to:
- Type and send messages from the chat window in your web browser to Arduino. This method can be modified to control Arduino.
- Receive messages from Arduino instantly. This setup can be modified to monitor Arduino in real-time.
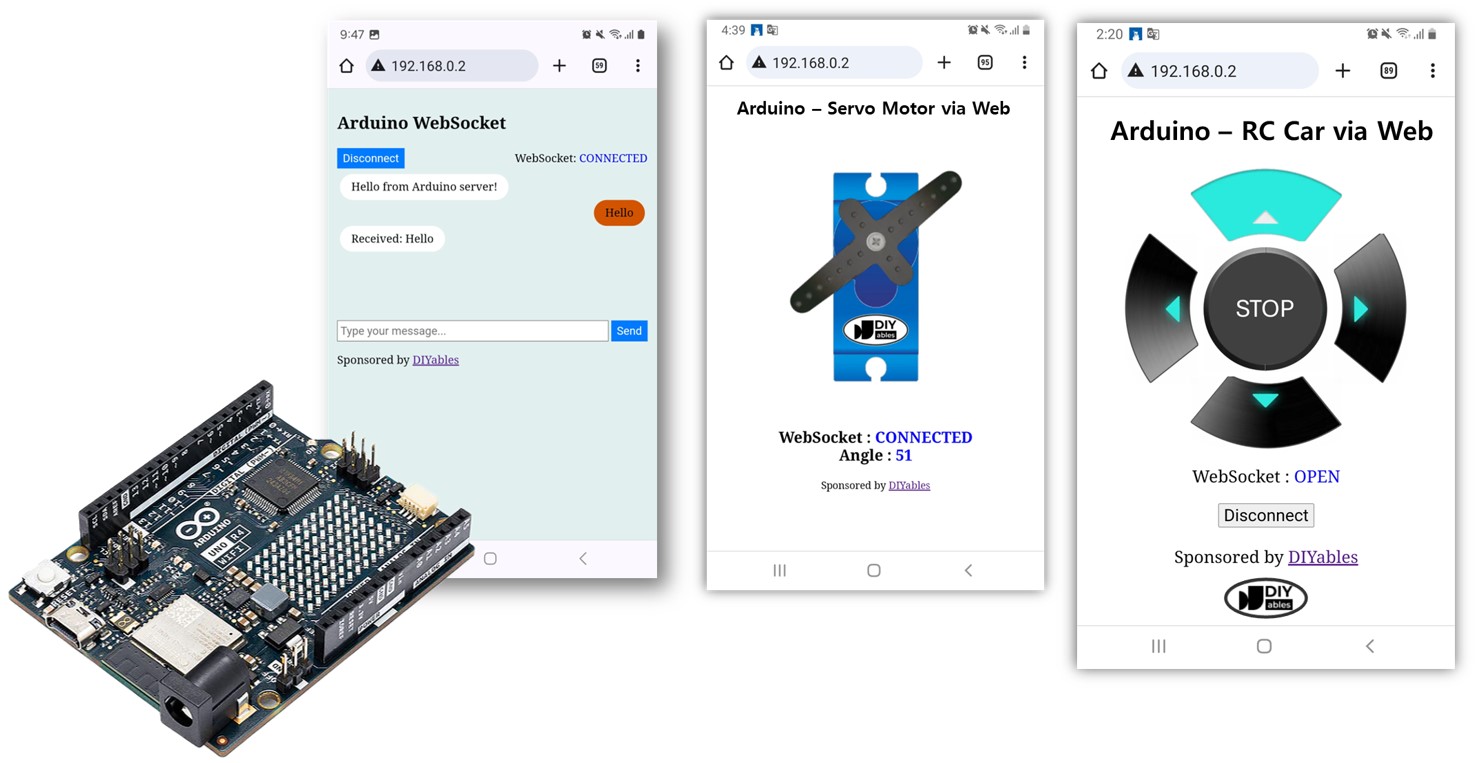
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
What is Arduino Websocket?
You might be wondering, "What is WebSocket?" It's quite straightforward: WebSocket is a technology that enables a web browser to communicate directly with a web server in real time.
- Without WebSocket, you would need to refresh the webpage to see new updates. This isn't very practical.
- With WebSocket, the webpage remains continuously connected to the server. This allows them to exchange information instantly without needing to refresh the page.
You likely encounter WebSocket technology regularly in everyday web applications such as online games, instant messaging, and stock market updates.
Why we need WebSocket to smoothly control Arduino?
Imagine you want to control your remote-controlled car using a web interface on your phone or computer. Without WebSocket, each time you want to change the car's direction or speed, you'd need to refresh the web page. It's like having to press a "reload" button every time you issue a command to the car.
With WebSocket, however, it's as if there is a continuous and direct connection between your phone or computer and the car. You don't need to refresh the page to steer the car or change its speed. It’s as though the car constantly listens to your commands in real-time, without any delays caused by having to reload the page.
Overall, WebSocket facilitates:
- Sending data from the web browser to Arduino without needing to reload the webpage.
- Sending data from Arduino back to the web browser without refreshing the page.
This enables smooth two-way communication in real-time.
Benefits of WebSocket with Arduino:
- Real-Time Control: WebSocket enables instant communication with the Arduino, ensuring quick responses to commands for a seamless user experience.
- Persistent Connection: Maintain a continuous link without refreshing the control page, creating an always-ready line of communication for immediate instructions.
- Efficiency: Experience prompt responses without the need for constant page reloading, enhancing overall user enjoyment and efficiency.
Web Chat with Arduino via WebSocket
The webpage's content (HTML, CSS, JavaScript) are stored separately on an index.h file. So, we will have two code files on Arduino IDE:
- An .ino file that is Arduino code, which creates a web sever and WebSocket server
- An .h file, which contains the webpage's content.
Quick Steps
- If this is the first time you use Arduino Uno R4, see how to setup environment for Arduino Uno R4 on Arduino IDE.
- Connect the Arduino board to your PC via a micro USB cable
- Open Arduino IDE on your PC.
- Select the right Arduino board (Arduino Uno R4 WiFi) and COM port.
- Open the Library Manager by clicking on the Library Manager icon on the left navigation bar of Arduino IDE.
- Search “mWebSockets”, then find the mWebSockets created by Dawid Kurek.
- Click Install button to install mWebSockets library.
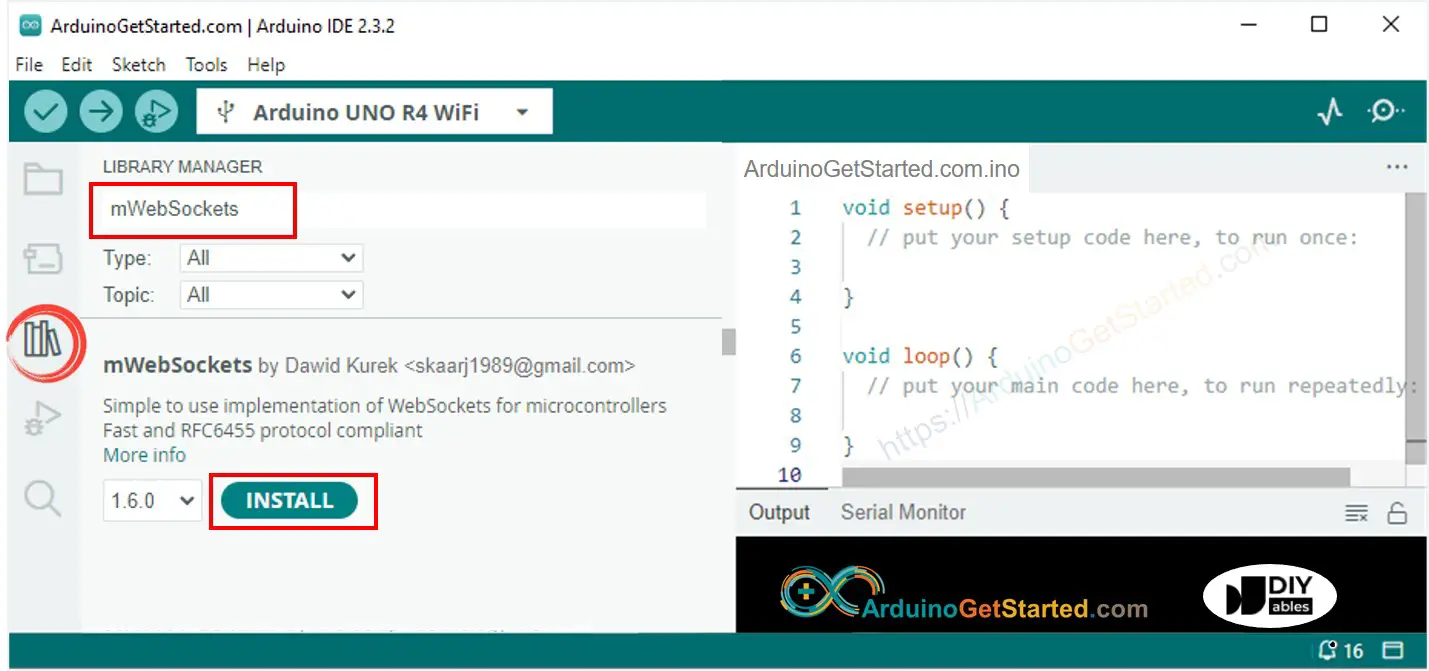
- On Arduino IDE, create new sketch, Give it a name, for example, ArduinoGetStarted.com.ino
- Copy the below code and open with Arduino IDE
- Modify the WiFi information (SSID and password) in the code to match your own network credentials.
- Create the index.h file On Arduino IDE by:
- Either click on the button just below the serial monitor icon and choose New Tab, or use Ctrl+Shift+N keys.
- Give the file's name index.h and click OK button
- Copy the below code and paste it to the index.h.
- Now you have the code in two files: ArduinoGetStarted.com.ino and index.h
- Click Upload button on Arduino IDE to upload code to Arduino.
- Go to C:\Users\YOU_ACCOUNT\Documents\Arduino\libraries\mWebSockets\src/ directory.
- Find the config.h file and open it by a text editor.
- Look at the line 26, you will see it like below:
- Change this line to the below and save it:
- Click Upload button on Arduino IDE to upload code to Arduino.
- Open the Serial Monitor
- Check out the result on Serial Monitor.
- Take note of the IP address displayed, and enter this address into the address bar of a web browser on your smartphone or PC.
- You will see the webpage it as below:
- Click the CONNECT button to connect the webpage to Arduino via WebSocket.
- Type some words and send them to Arduino.
- You will see the response from Arduino.
- If you modify the HTML content in the index.h and does not touch anything in ArduinoGetStarted.com.ino file, when you compile and upload code to Arduino, Arduino IDE will not update the HTML content.
- To make Arduino IDE update the HTML content in this case, make a change in the ArduinoGetStarted.com.ino file (e.g. adding empty line, add a comment....)
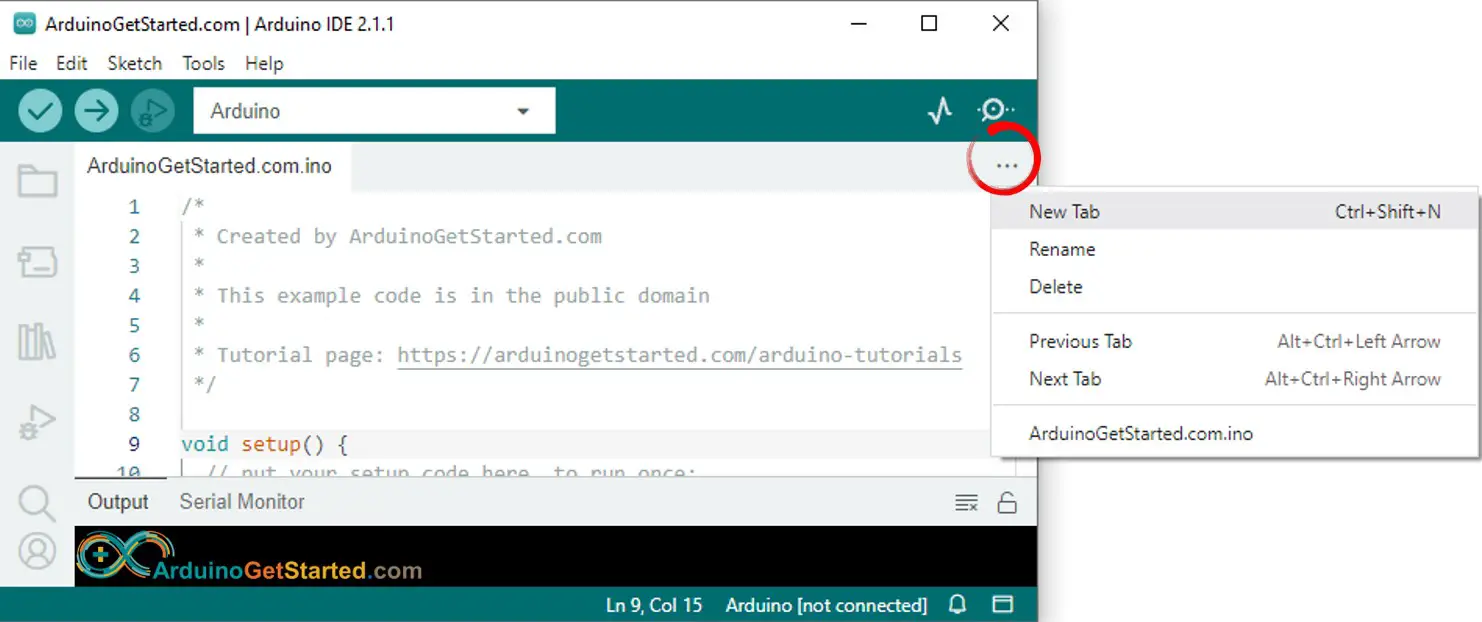
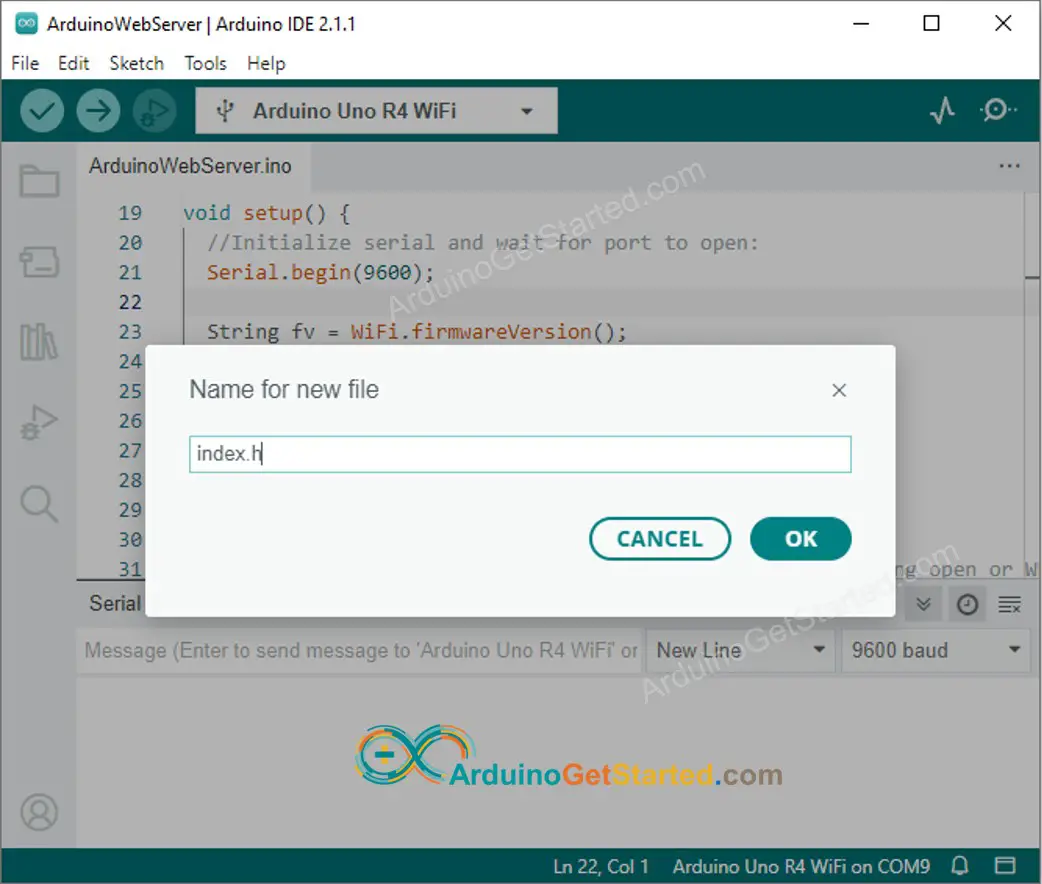
You will see an error like below:
To fix this error:
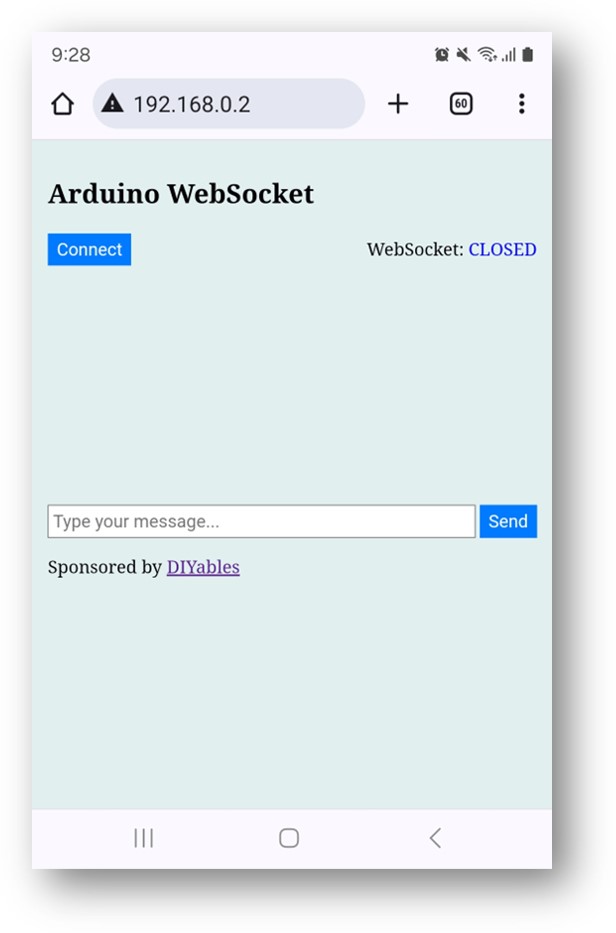
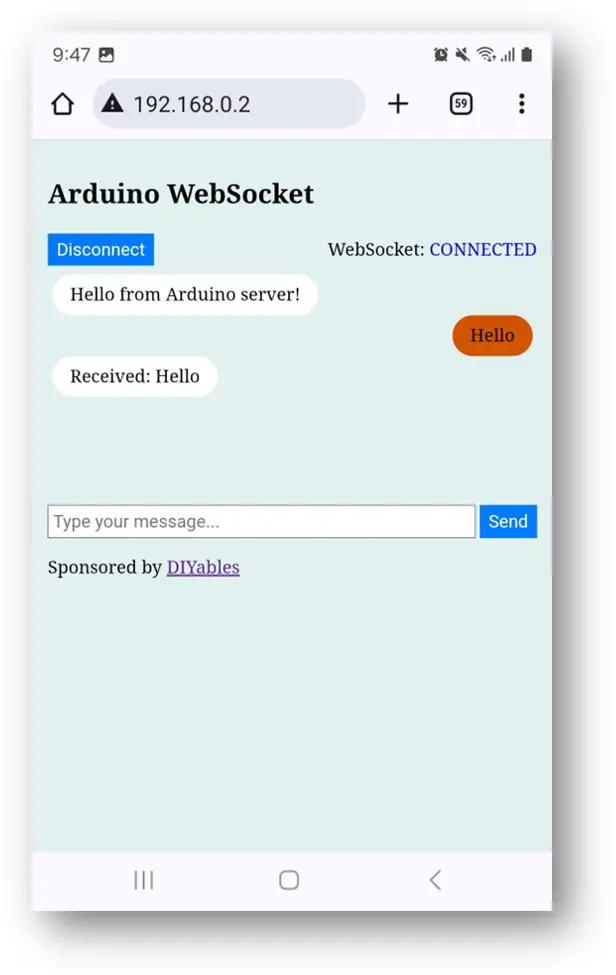
※ NOTE THAT:
Line-by-line Code Explanation
The above Arduino code contains line-by-line explanation. Please read the comments in the code!
How the System Works
The Arduino code operates by setting up both a web server and a WebSocket server. Here’s how it works:
- When you enter the Arduino's IP address into a web browser, it requests the webpage (User Interface) from the Arduino.
- The Arduino’s web server then sends the content of the webpage (HTML, CSS, JavaScript) to your browser.
- Your web browser displays the webpage.
- Upon clicking the CONNECT button on the webpage, the JavaScript code within the page initiates a WebSocket connection with the WebSocket server on the Arduino.
- Once the WebSocket connection is active, if you type something and hit the SEND button, the JavaScript sends your text to the Arduino via the WebSocket connection in the background.
- After receiving your input, the WebSocket server on the Arduino sends a response back to your webpage.
You can learn other Arduino WebSocket examples below: