Arduino - Keypad 1x4
In this tutorial, we will learn how to use a keypad 1x4 with an Arduino. In detaile, we will learn:
- How to connect keypad 1x4 to Arduino.
- How to program Arduino to read the pressed keys from keypad 1x4.
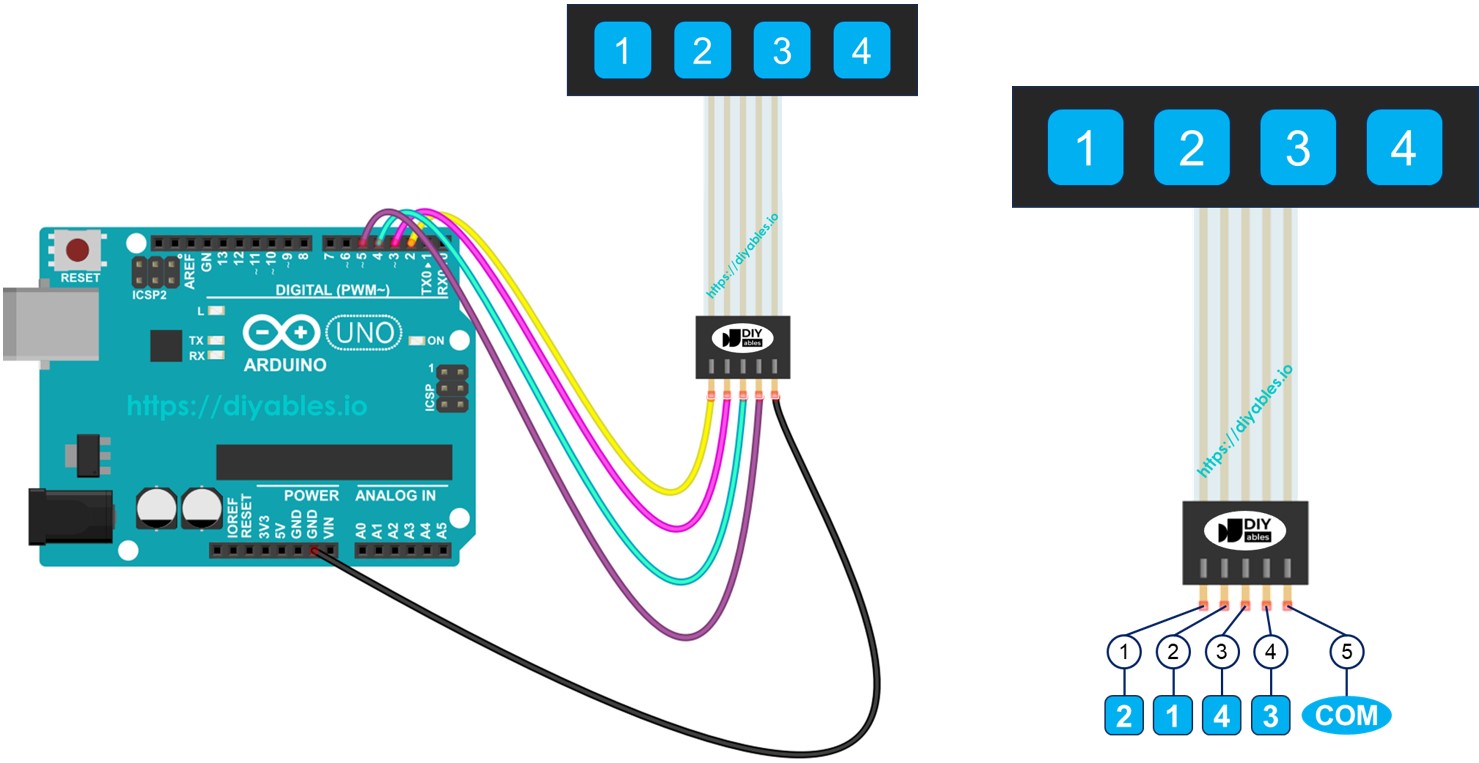
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
About Keypad 1x4
A 1x4 keypad is composed of four membrane buttons arranged in a single row. It is commonly used for user input in projects such as passcode entry, menu navigation, or control interfaces.
Pinout
The 1x4 keypad has 5 pins, which do not correspond directly to the key labels in order. Specifically:
- Pin 1: connects to key 2
- Pin 2: connects to key 1
- Pin 3: connects to key 4
- Pin 4: connects to key 3
- Pin 5: is a common pin that connects to all keys
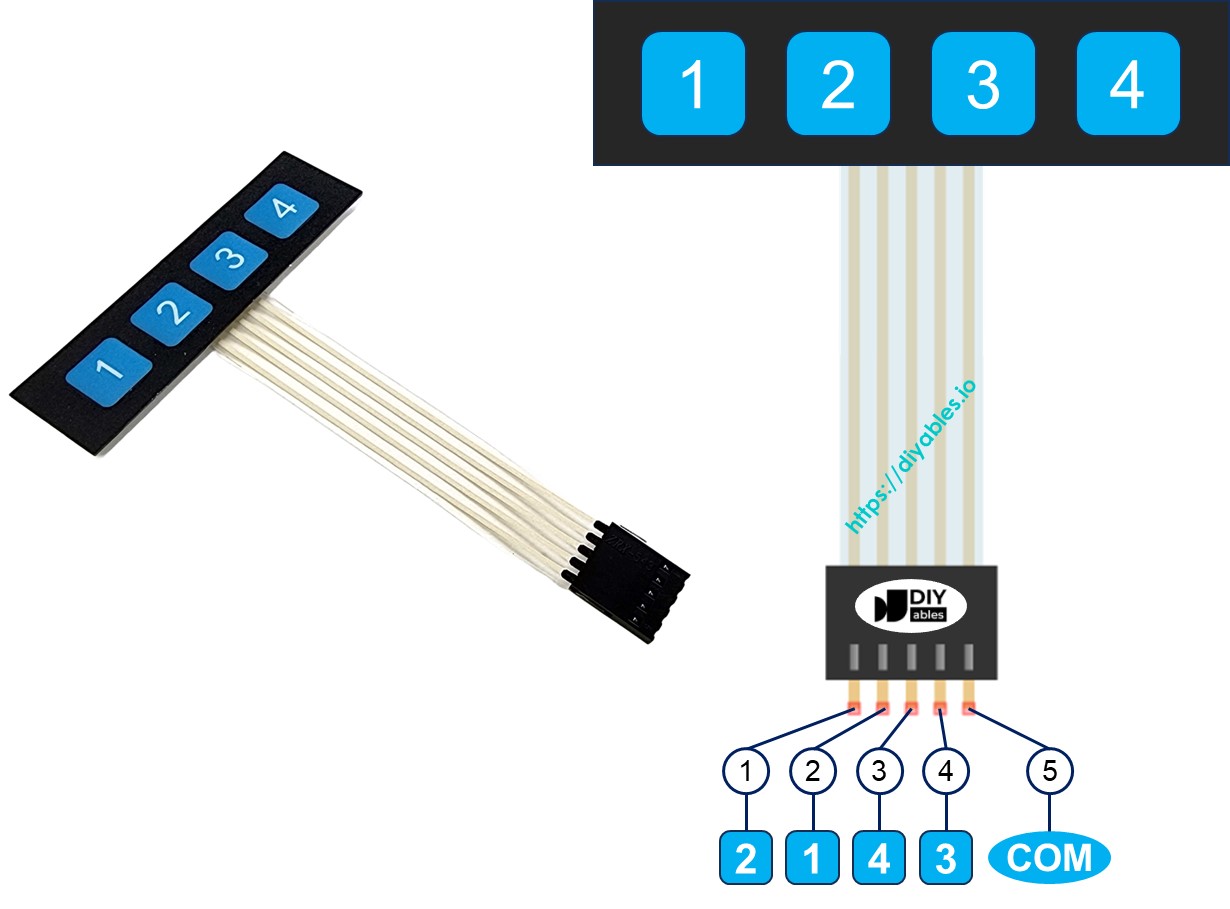
Wiring Diagram
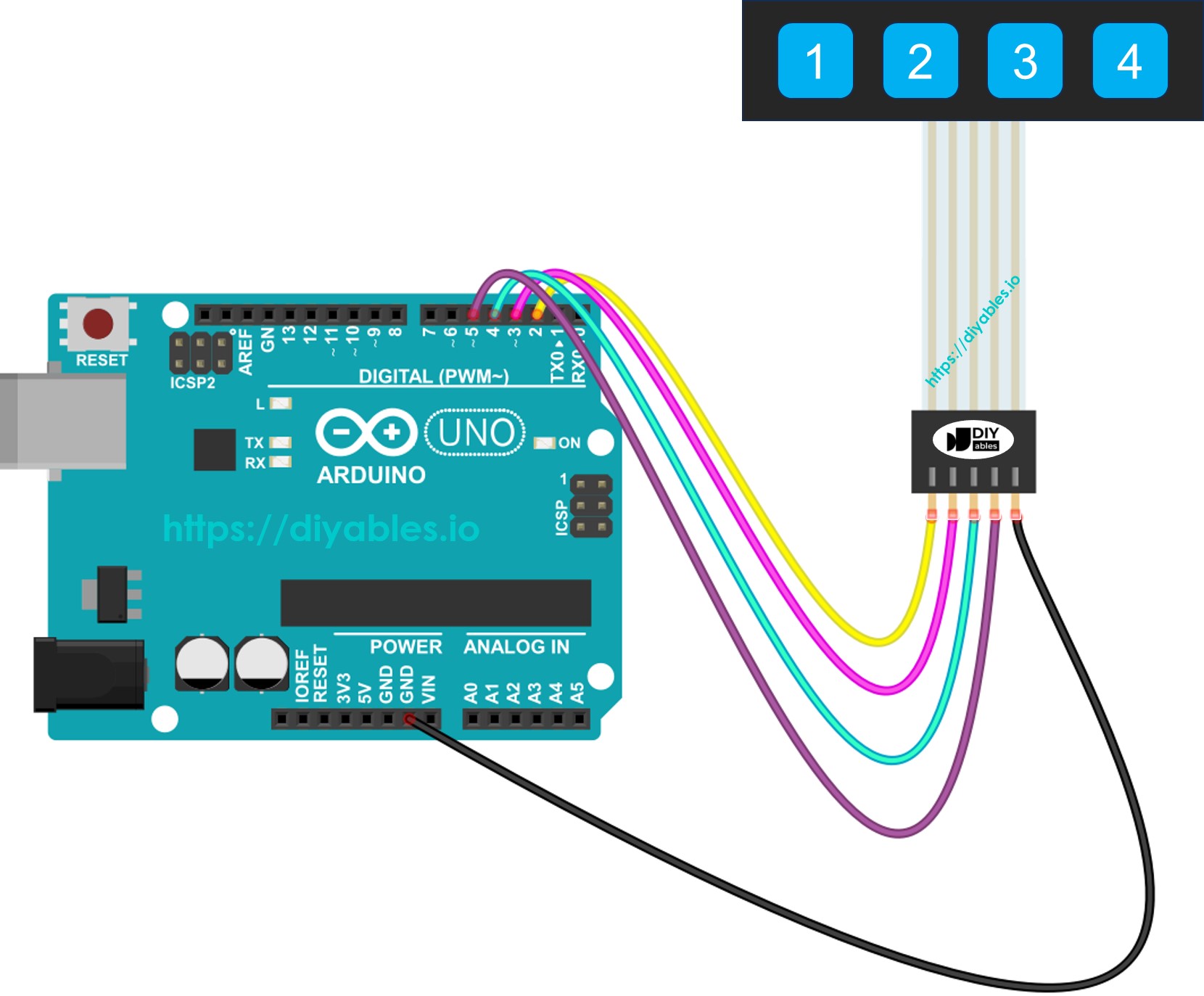
This image is created using Fritzing. Click to enlarge image
Arduino Code
Each key on the 1x4 keypad functions as a button. This means we can use the digitalRead() function to check the status of each key. However, in practice, like with any button, we must handle the issue of bouncing, where a single press might be mistakenly detected as multiple presses. To avoid this, we need to debounce each key. This task becomes challenging when trying to debounce four keys without blocking other parts of the code. Fortunately, the ezButton library simplifies this process.
Quick Steps
- Connect Arduino to the keypad 1x4
- Connect Arduino to PC via USB cable
- Open Arduino IDE, select the right board and port
- Navigate to the Libraries icon on the left bar of the Arduino IDE.
- Search “ezButton”, then find the button library by ArduinoGetStarted.com
- Click Install button to install ezButton library.
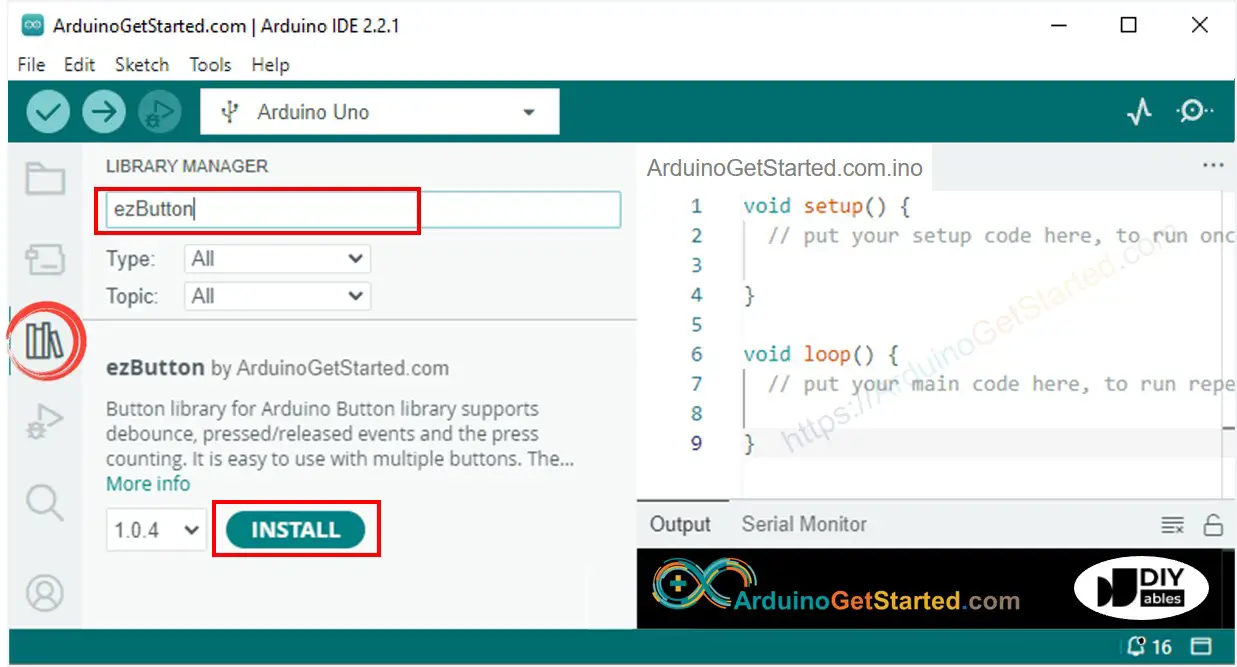
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- Open Serial Monitor
- Press keys on keypad 1x4 one by one
- See the result in Serial Monitor