Arduino - Door Open Email Notification
In this guide, we will learn how to use an Arduino to send emails when a door opens. We will explain how to set it up, what parts you need, and give you detailed steps to connect an Arduino with a door sensor and an email service. This will help keep your home secure by sending you instant email alerts when the door is opened.
Or you can buy the following sensor kits:
Disclosure: Some links in this section are Amazon affiliate links. If you make a purchase through these links, we may earn a commission at no extra cost to you.
Additionally, some links direct to products from our own brand,
DIYables .
We offer detailed tutorials on Door Sensors and Gmail. Each guide includes comprehensive details and clear, step-by-step instructions on hardware setup, the principles of operation, and how to connect and program the Arduino. You can find out more about these tutorials through these links:
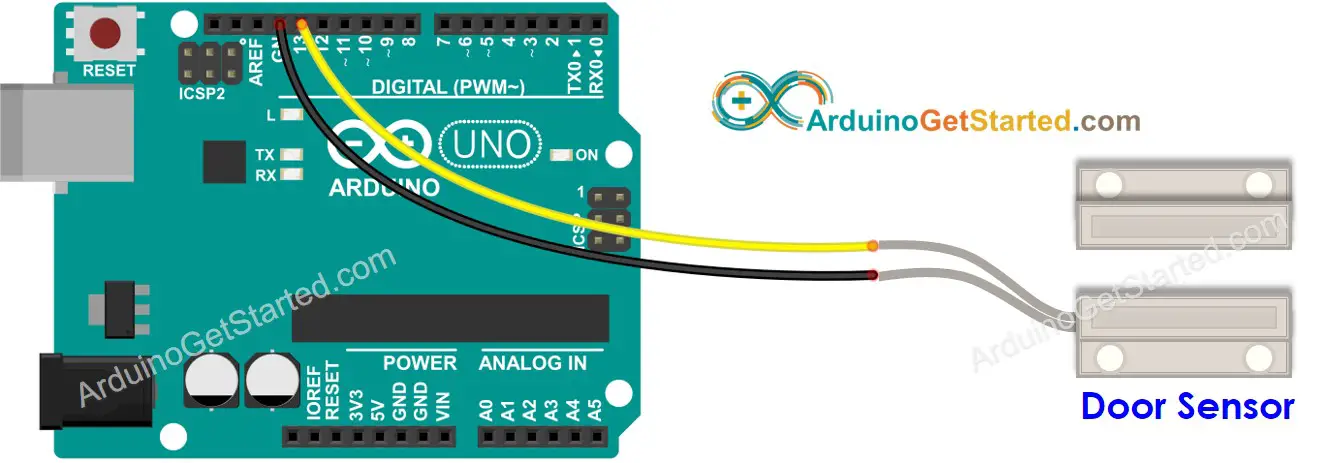
This image is created using Fritzing. Click to enlarge image
#include <WiFiS3.h>
#include <ESP_Mail_Client.h>
#define WIFI_SSID "YOUR_WIFI_SSID"
#define WIFI_PASSWORD "YOUR_WIFI_PASSWORD"
#define SENDER_EMAIL "xxxxxx@gmail.com"
#define SENDER_PASSWORD "xxxx xxxx xxxx xxxx"
#define RECIPIENT_EMAIL "xxxxxx@gmail.com"
#define SMTP_HOST "smtp.gmail.com"
#define SMTP_PORT 587
#define DOOR_SENSOR_PIN 13
int door_state;
int prev_door_state;
SMTPSession smtp;
void setup() {
Serial.begin(9600);
WiFi.begin(WIFI_SSID, WIFI_PASSWORD);
Serial.print("Connecting to Wi-Fi");
while (WiFi.status() != WL_CONNECTED) {
Serial.print(".");
delay(300);
}
Serial.println();
Serial.print("Connected with IP: ");
Serial.println(WiFi.localIP());
Serial.println();
pinMode(DOOR_SENSOR_PIN, INPUT_PULLUP);
door_state = digitalRead(DOOR_SENSOR_PIN);
}
void loop() {
prev_door_state = door_state;
door_state = digitalRead(DOOR_SENSOR_PIN);
if (prev_door_state == LOW && door_state == HIGH) {
Serial.println("The door is opened");
String subject = "Email Notification from Arduino";
String textMsg = "This is an email sent from Arduino.\n";
textMsg += "Your door is opened";
gmail_send(subject, textMsg);
} else if (prev_door_state == HIGH && door_state == LOW) {
Serial.println("The door is closed");
}
}
void gmail_send(String subject, String textMsg) {
MailClient.networkReconnect(true);
smtp.debug(1);
smtp.callback(smtpCallback);
Session_Config config;
config.server.host_name = SMTP_HOST;
config.server.port = SMTP_PORT;
config.login.email = SENDER_EMAIL;
config.login.password = SENDER_PASSWORD;
config.login.user_domain = F("127.0.0.1");
config.time.ntp_server = F("pool.ntp.org,time.nist.gov");
config.time.gmt_offset = 3;
config.time.day_light_offset = 0;
SMTP_Message message;
message.sender.name = F("Arduino");
message.sender.email = SENDER_EMAIL;
message.subject = subject;
message.addRecipient(F("To Whom It May Concern"), RECIPIENT_EMAIL);
message.text.content = textMsg;
message.text.transfer_encoding = "base64";
message.text.charSet = F("utf-8");
message.priority = esp_mail_smtp_priority::esp_mail_smtp_priority_low;
message.addHeader(F("Message-ID: <abcde.fghij@gmail.com>"));
if (!smtp.connect(&config)) {
Serial.println("Connection error: ");
Serial.print("- Status Code: ");
Serial.println(smtp.statusCode());
Serial.print("- Error Code: ");
Serial.println(smtp.errorCode());
Serial.print("- Reason: ");
Serial.println(smtp.errorReason().c_str());
return;
}
if (!smtp.isLoggedIn()) {
Serial.println("Not yet logged in.");
} else {
if (smtp.isAuthenticated())
Serial.println("Successfully logged in.");
else
Serial.println("Connected with no Auth.");
}
if (!MailClient.sendMail(&smtp, &message)) {
Serial.println("Connection error: ");
Serial.print("- Status Code: ");
Serial.println(smtp.statusCode());
Serial.print("- Error Code: ");
Serial.println(smtp.errorCode());
Serial.print("- Reason: ");
Serial.println(smtp.errorReason().c_str());
}
}
void smtpCallback(SMTP_Status status) {
Serial.println(status.info());
if (status.success()) {
for (size_t i = 0; i < smtp.sendingResult.size(); i++) {
SMTP_Result result = smtp.sendingResult.getItem(i);
Serial.print("Status: ");
if (result.completed)
Serial.println("success");
else
Serial.println("failed");
Serial.print("Recipient: ");
Serial.println(result.recipients.c_str());
Serial.print("Subject: ");
Serial.println(result.subject.c_str());
}
Serial.println("----------------\n");
smtp.sendingResult.clear();
}
}
Attach the door sensor to your door.
Link the Arduino board with the door sensor.
Connect the Arduino board to your computer using a micro USB cable.
Launch the Arduino IDE on your computer.
Select the right Arduino board (Arduino Uno R4 WiFi) and COM port.
In Arduino IDE, click on the "Library Manager" icon on the left menu to open it.
Type ESP Mail Client in the search box, and look for ESP Mail Client by Mobizt.
Press the Install button to add the ESP Mail Client library.
Copy the provided code and open it using Arduino IDE.
Update your WiFi details (network name and password) in the code. Change the values of WIFI_SSID and WIFI_PASSWORD to your own.
Edit the sender's email and password in the code. Modify SENDER_EMAIL and SENDER_PASSWORD.
※ NOTE THAT:
Make sure the sender's email is from Gmail. Use the App password from the previous step as the sender's password. The recipient's email can be any email type.
The door is opened
#### Email sent successfully
> C: Email sent successfully
----------------
Message sent success: 1
Message sent failed: 0
----------------
Message No: 1
Status: success
Date/Time: May 27, 2024 04:42:50
Recipient: xxxxxx@gmail.com
Subject: Email Notification from Arduino
----------------
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
※ OUR MESSAGES
You can share the link of this tutorial anywhere. Howerver, please do not copy the content to share on other websites. We took a lot of time and effort to create the content of this tutorial, please respect our work!