Arduino - Switch
The ON/OFF switch, also called the toggle switch, has two state ON (closed) and OFF (open). The ON/OFF switch's state is toggle between ON/OFF each time it is presed, and the state is kept even when released. In this tutorial, we are going to learn how to use ON/OFF switch with Arduino.
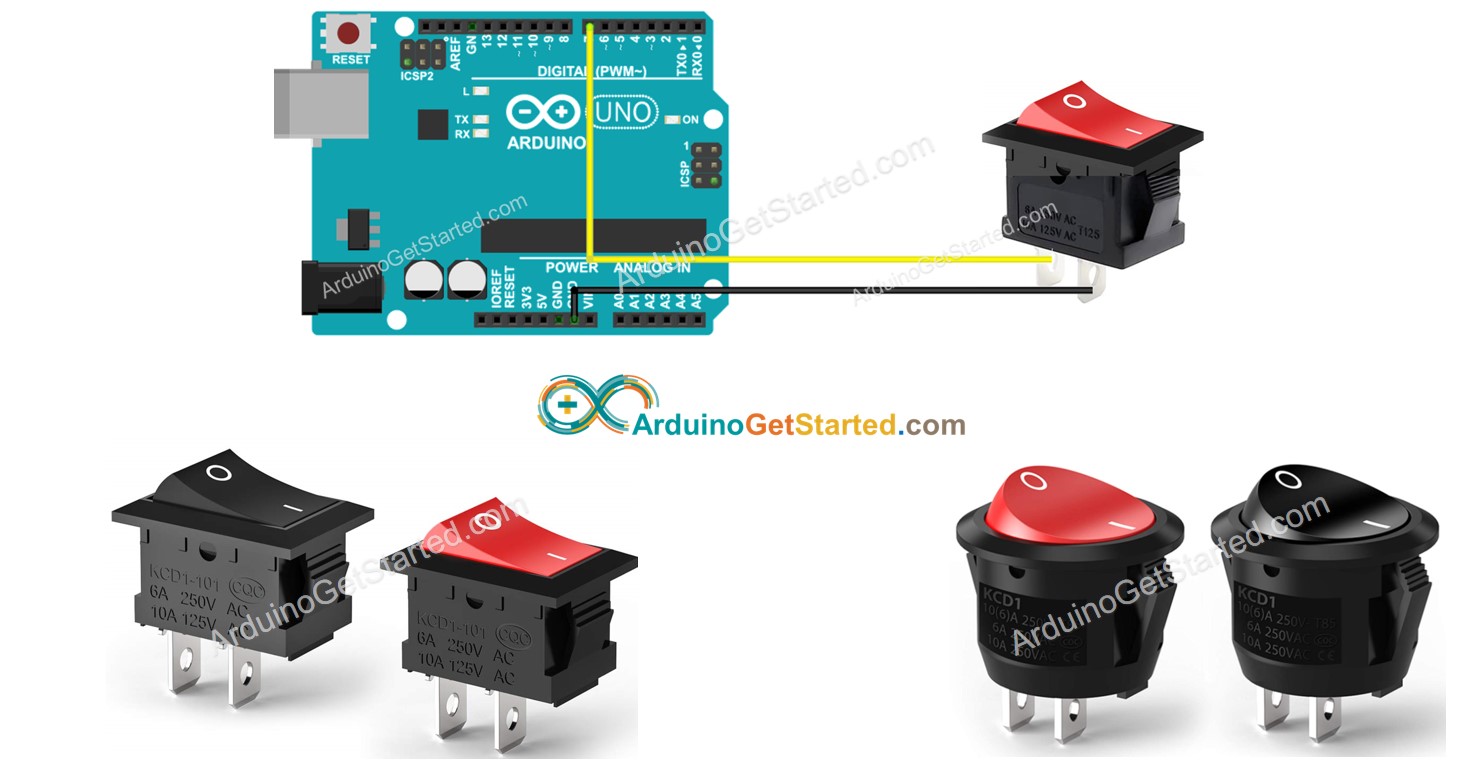
Please do not confuse with the following:
Hardware Required
Or you can buy the following sensor kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About ON/OFF Switch
An ON/OFF Switch is a switch that changes its state (ON to OFF, or OFF to ON) once pressed, and then keeps that state even when released. To change the state, we need to press it again.
Pinout
The ON/OFF Switch basically has two types: two-pin switch and three-pin switch
In this tutorial, we will use two-pin switch. In this type, we do NOT need to distinguish between the two pins.
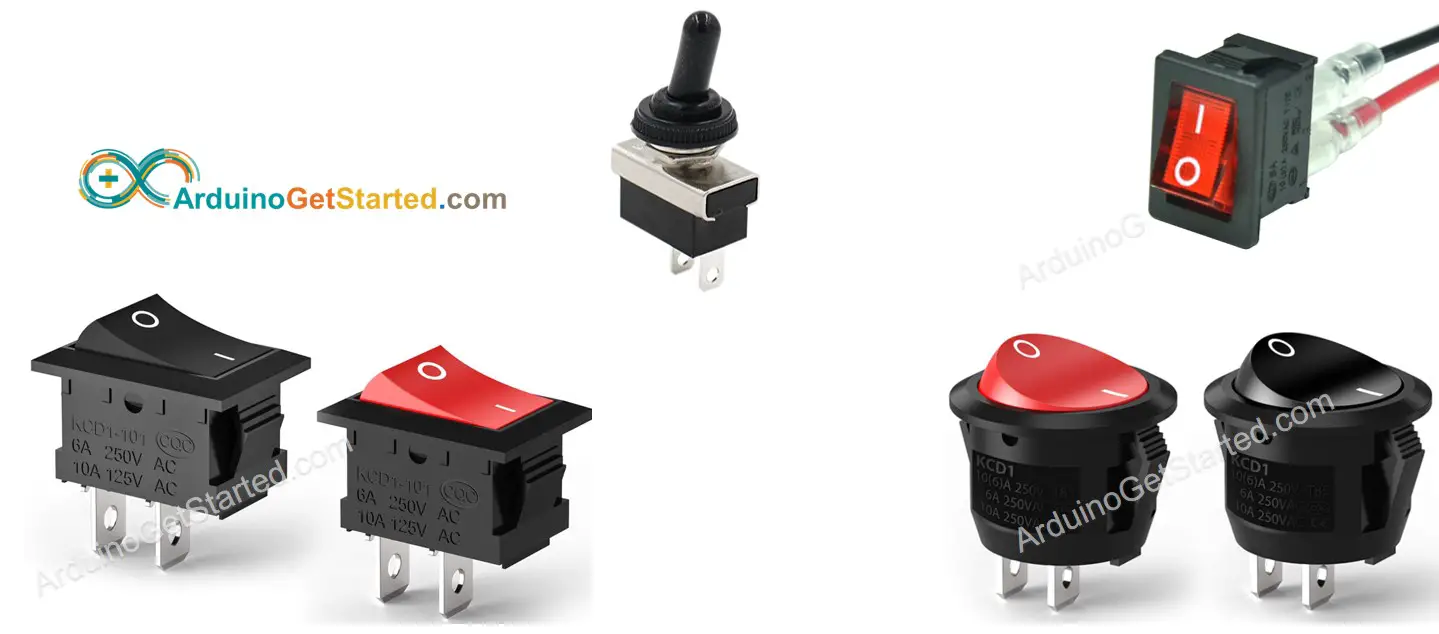
How It Works
There are two ways to use ON/OFF switch. The below is the wiring table for ON/OFF switch and the reading state on Arduino in both ways:
pin 1 | pin 2 | Arduino Input Pin's State | |
---|---|---|---|
1 | GND | Arduino Input Pin (with pull-up) | HIGH ⇒ OFF, LOW ⇒ ON |
2 | VCC | Arduino Input Pin (with pull-down) | HIGH ⇒ ON, LOW ⇒ OFF |
We only need to choose one of the above two ways. The rest of tutorial will use the first way.
Wiring Diagram
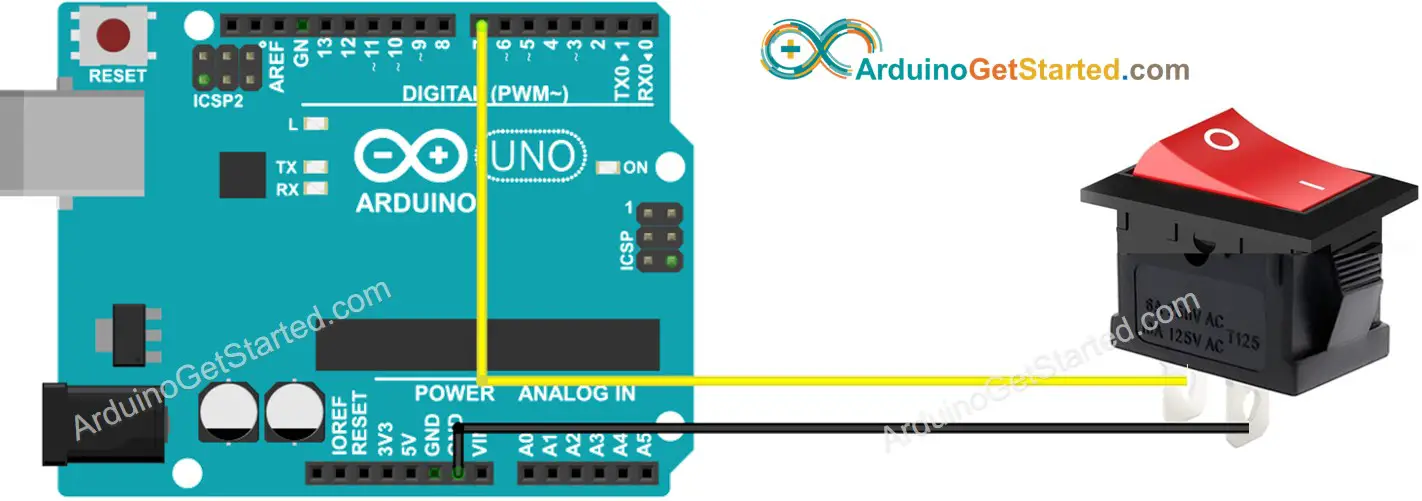
This image is created using Fritzing. Click to enlarge image
To make the wiring connection stable and firm, we recommend using Soldering Iron to solder wires and ON/OFF switch's pin together, and then use Heat Shrink Tube to make it safe.
Arduino Code - ON/OFF Switch
Just like a button, a ON/OFF switch also needs to be debounced (See more at Why needs debounce for the button, ON/OFF switch?). Debouncing make the code complicated. Fortunately, the ezButton library supports the debouncing functionm, The library also uses internal pull-up register. These make easy to us to program.
※ NOTE THAT:
There are two wide-used use cases:
- The first: If the switch's state is ON, do something. If the input state is OFF, do another thing in reverse.
- The second: If the switch's state is changed from ON to OFF (or OFF to ON), do something.
Quick Steps
- Do wiring as above wiring diagram
- Connect Arduino to PC via USB cable
- Open Arduino IDE
- Install ezButton library. See How To
- Select the right board and port
- Click Upload button on Arduino IDE to upload code to Arduino
- Press the switch to ON.
- See the result on Serial Monitor.
- Then press the switch to OFF.
- See the result on Serial Monitor.
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.