Arduino - Relay
In a previous tutorial, we have learned how to turn on/off an LED. In this tutorial, we are going to learn how to turn on/off some kind of devices that use the high voltage power supply(such as a light bulb, fan, electromagnetic lock, linear actuator...).
The common: Just like controlling LED, we use the Arduino's output pin to turn on/off them.
The difference:
- For LED, we can use power from the Arduino board (≤ 5v). Therefore, we can connect LED directly to Arduino's pin.
- For the light bulb, we MUST use another power source (high voltage and/or high current), which can burn Arduino. Therefore, we CANNOT connect the light bulb directly to Arduino's pin. We need to use a relay between Arduino's pin and light bulb to protect Arduino from high voltage/current.
Hardware Required
Or you can buy the following kits:
1 | × | DIYables Sensor Kit (30 sensors/displays) | |
1 | × | DIYables Sensor Kit (18 sensors/displays) |
Additionally, some links direct to products from our own brand, DIYables .
About Relay
A relay is a programmable electrical switch, which can be controlled by Arduino or any micro-controller. It is used to programmatically control on/off the devices, which use the high voltage and/or high current.
It is a bridge between Arduino and high voltage devices.
WARNING
When you are making projects that are connected to mains voltage, you need to know what you are doing, otherwise, you may shock yourself. This is a serious topic, and we want you to be safe. If you’re NOT 100% sure what you are doing, do yourself a favor and don’t touch anything. Ask someone who knows!
Although some kinds of relays support both DC and AC devices, We highly recommend you to use a DC device (≤24V) for testing.
Relay Pinout
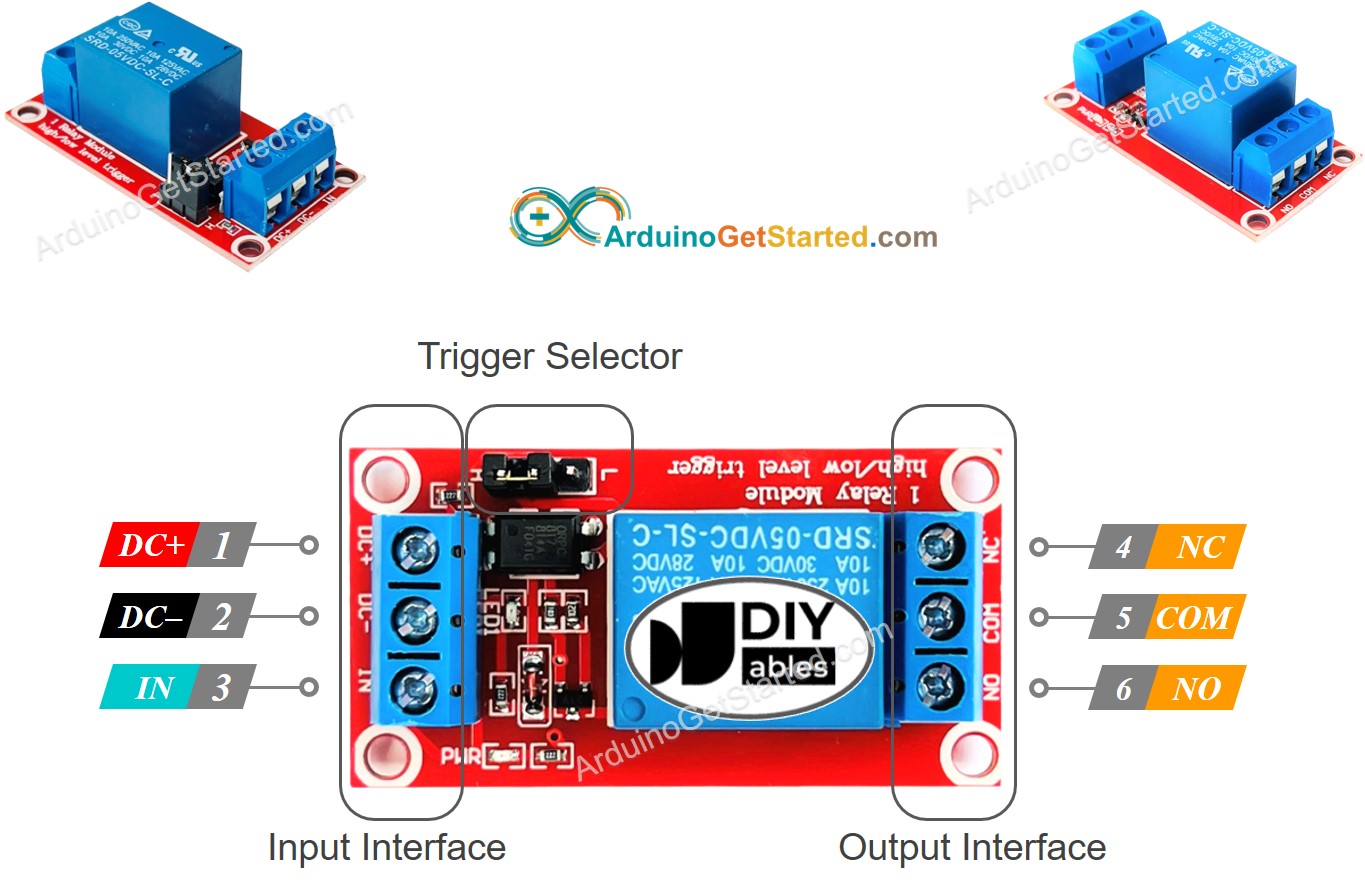
Relay has two groups of pins: input (low voltage) group and output (high voltage) group.
- Pins in the input group are connected to Arduino, including three pins:
- DC- pin: needs to be connected to GND (0V)
- DC+ pin: needs to be connected to VCC (5V)
- IN pin: receives the control signal from Arduino
- Pins in the output group are connected to the high voltage device, including three pins (usually in screw terminal):
- COM pin: is the common pin. It is used in both normally open mode and normally closed mode
- NO pin: is normally open pin. It is used in the normally open mode
- NC pin: is normally closed pin. It is used in the normally closed mode
- We use only COM pin and NO pin if we use normally open mode.
- We use only COM pin and NC pin if we use normally closed mode.
- LOW level trigger mode
- HIGH level trigger mode
- normally open mode
- normally closed mode. These modes are the opposite.
- The normally open and normally closed mode work oppositely
- The most of relay modules supports both normally open and normally closed mode
- The LOW level trigger and HIGH level trigger mode work oppositely
- NOT all of relay modules supports both LOW level trigger and HIGH level trigger mode
- At a time, The relay module can work at only one of two LOW level trigger and HIGH level trigger mode
- If the IN pin is connected to LOW (0V), the switch is open. The device is OFF (or inactive).
- If the IN pin is connected to HIGH (5V), the switch is closed. The device is ON (or active).
- If the IN pin is connected to LOW (0V), the switch is closed. The device is ON (or active).
- If the IN pin is connected to HIGH (5V), the switch is open. The device is OFF (or inactive).
- Connect an Arduino's pin to the IN pin of the relay
- Control the relay by programming the pin to LOW or HIGH
In practice, we usually do NOT use all of the pins in the high voltage group. We use only two of them:
Additionally, if the relay supports both LOW and HIGH level triggers, there is usually a jumper to select one of two: LOW level trigger or HIGH level trigger.
※ NOTE THAT:
The order of the relay module's pins can vary between manufacturers. ALWAYS use the labels printed on the relay. Look closely!
How to Connect the High Voltage Device to Relay
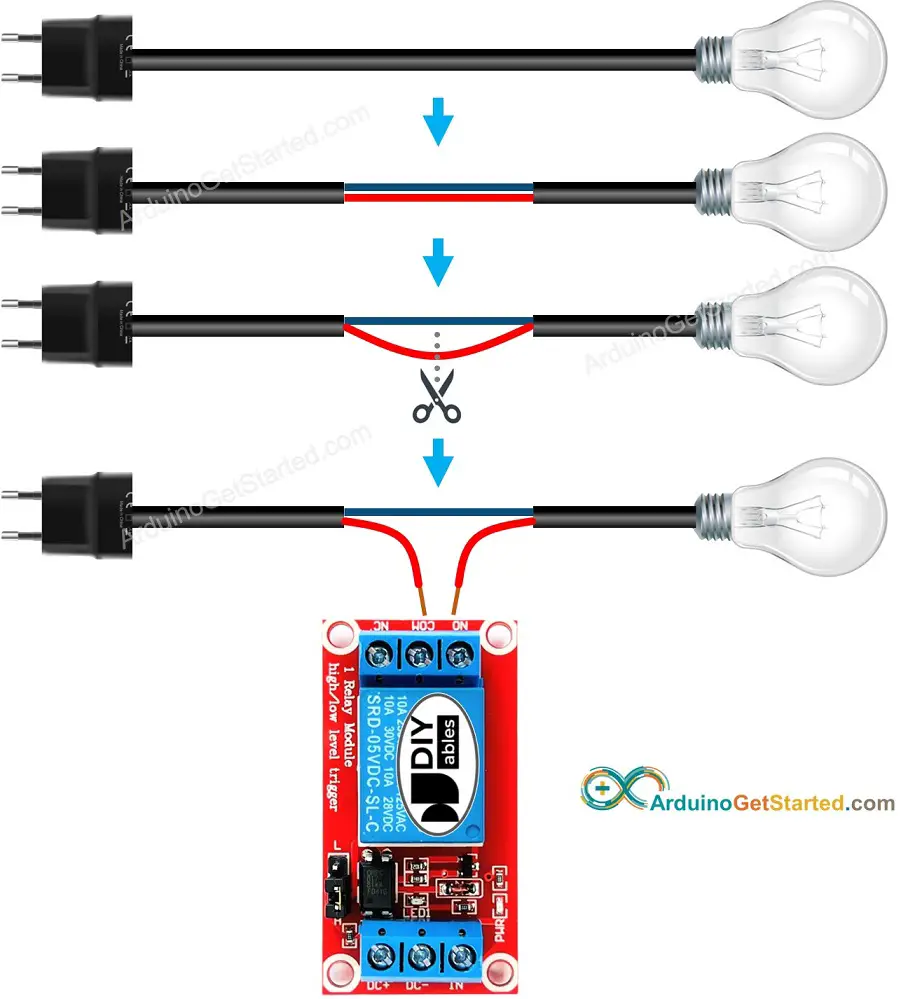
How It Works
Depending on manufacturers and user's installation, a relay can work differently.
The input mode mode (for IN pin): There are two input modes that make relay works oppositely:
The output mode mode (for output pins): There are two output modes that make relay works oppositely:
The “normally” means “if IN pin is connected to LOW (0V)”.
Before going into detail, let's see some quick information:
The combination of the input modes mode and output modes modes creates many use cases. If you are a beginner, we recommend using HIGH level trigger mode and normally open mode
Because the LOW level trigger and HIGH level trigger mode work oppositely, The next will explain the HIGH level trigger mode in detail. The LOW level trigger works oppositely.
HIGH Level Trigger - Normally Open Mode
To use this mode, we need to connect the high voltage device to the COM pin and NO pin.
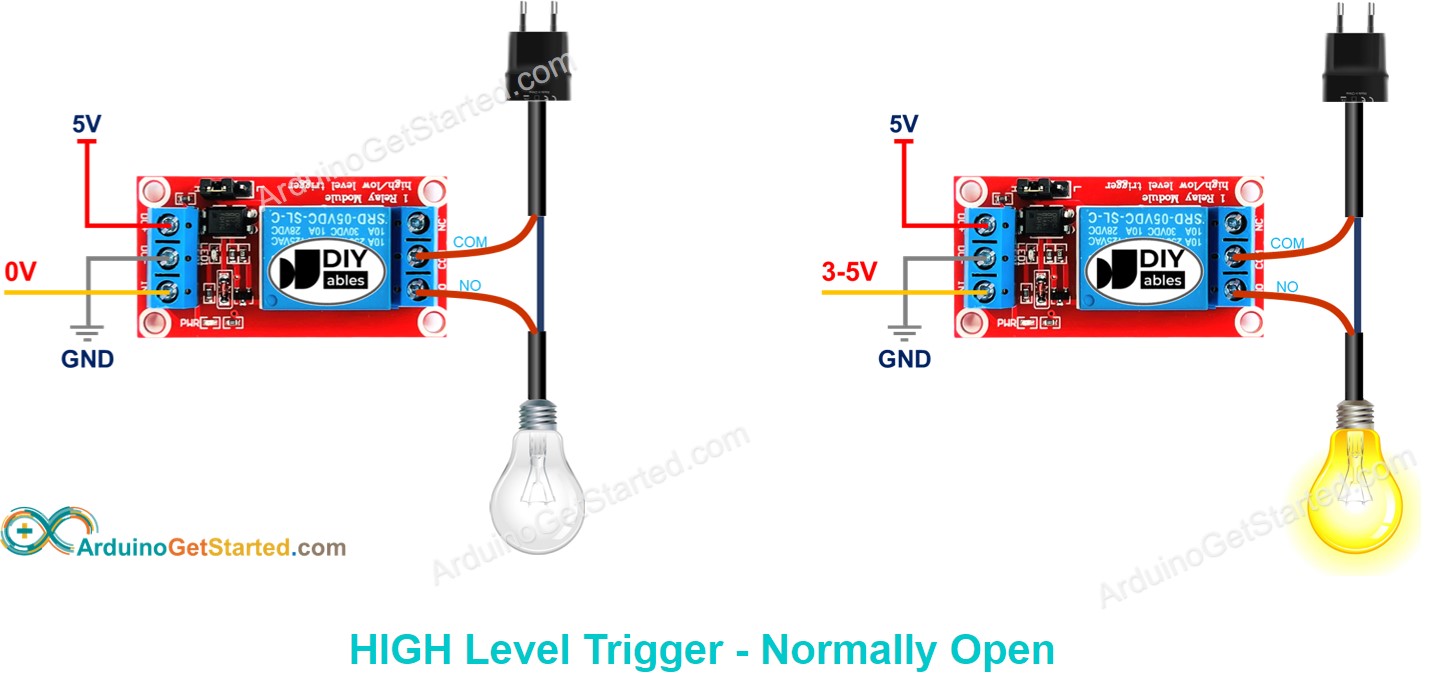
HIGH Level Trigger - Normally Closed Mode
To use this mode, we need to connect the high voltage device to the COM pin and NC pin.
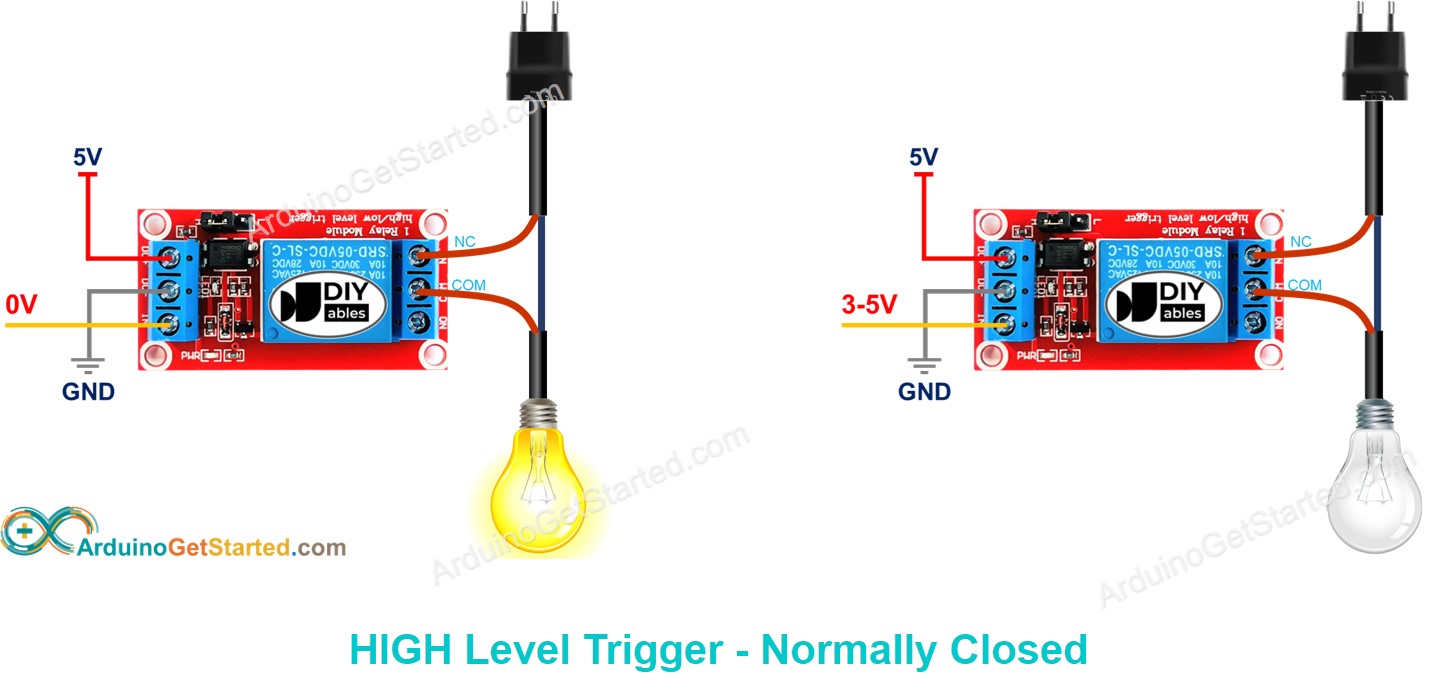
Summary
Input modes | Output Modes | IN pin (programmable) | Output pins | Relay state | Device state |
---|---|---|---|---|---|
HIGH Trigger | Normally Open | LOW | COM and NO pin | ⇒ open | ⇒ OFF |
HIGH Trigger | Normally Open | HIGH | COM and NO pin | ⇒ closed | ⇒ ON |
HIGH Trigger | Normally Closed | LOW | COM and NC pin | ⇒ closed | ⇒ ON |
HIGH Trigger | Normally Closed | HIGH | COM and NC pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Open | LOW | COM and NO pin | ⇒ closed | ⇒ ON |
LOW Trigger | Normally Open | HIGH | COM and NO pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Closed | LOW | COM and NC pin | ⇒ open | ⇒ OFF |
LOW Trigger | Normally Closed | HIGH | COM and NC pin | ⇒ closed | ⇒ ON |
There are up to 8 use cases. It may overload you. However, If you are a newbie, you just need to care about the two first cases, where HIGH level trigger and normally open are used. The rest of this tutorial will use those two use cases
Arduino - Relay
Arduino controls a high voltage device by controlling a relay.
Controlling a relay is simple. We just need:
Wiring Diagram
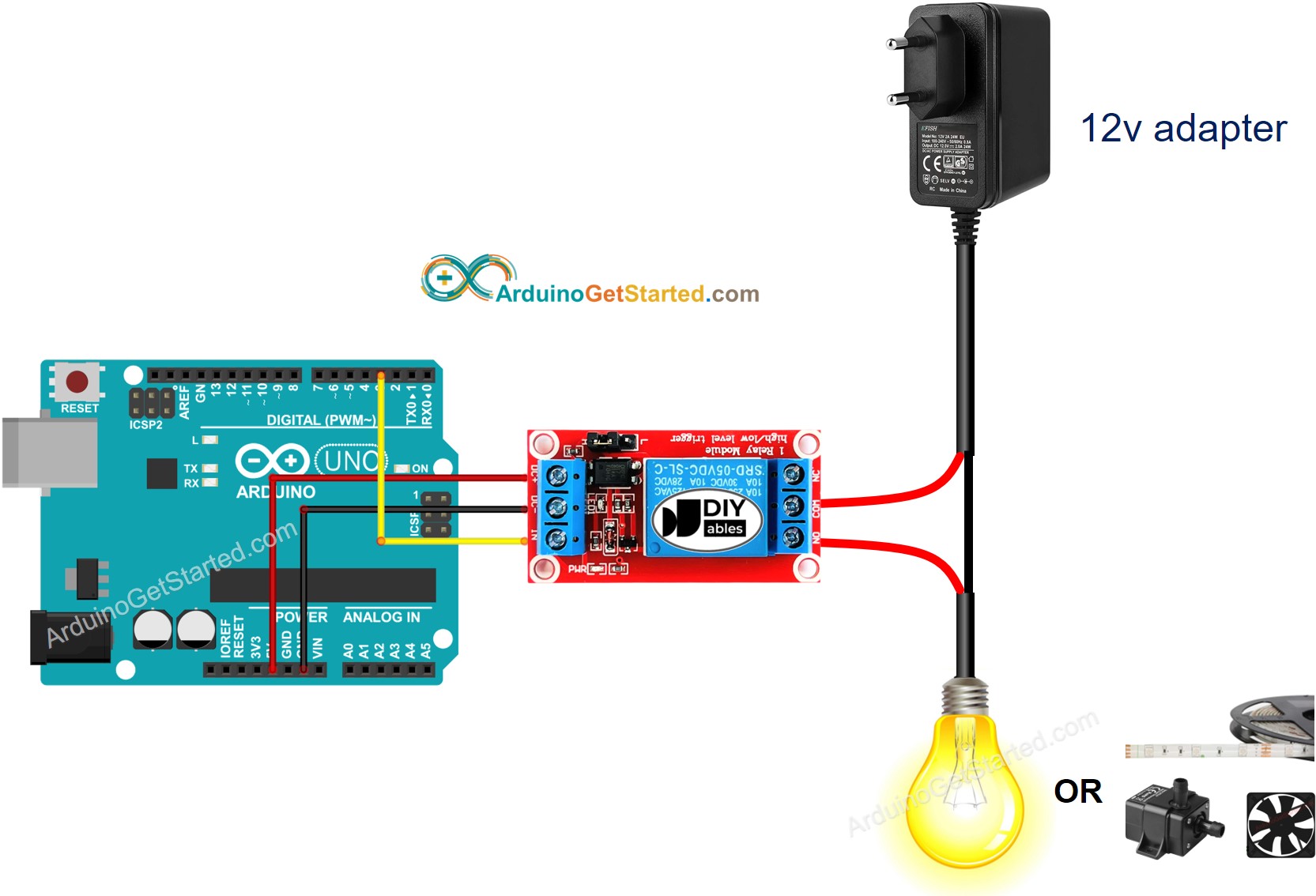
This image is created using Fritzing. Click to enlarge image
How To Program For Relay
- Configure an Arduino's pin to the digital output mode by using pinMode() function. For example, pin 3:
- Program the pin to LOW (0V) by using digitalWrite() function:
- Program the pin to HIGH (5V) by using digitalWrite() function:
Arduino Code
Quick Steps
- Copy the above code and open with Arduino IDE
- Click Upload button on Arduino IDE to upload code to Arduino
- See LED strip state: blinking
Video Tutorial
We are considering to make the video tutorials. If you think the video tutorials are essential, please subscribe to our YouTube channel to give us motivation for making the videos.
Challenge Yourself
- Automatically turn on the light when you enter into your room and turn off the light after you leave 30 seconds. Hint: Refer to Arduino - Motion Sensor.